Mongoose query where value is not null
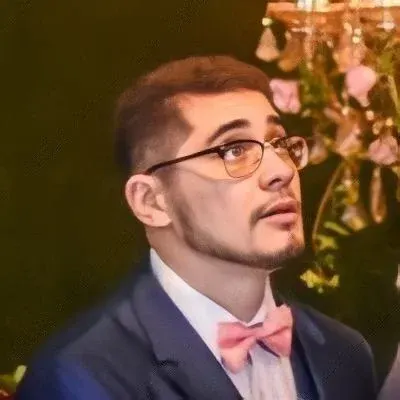
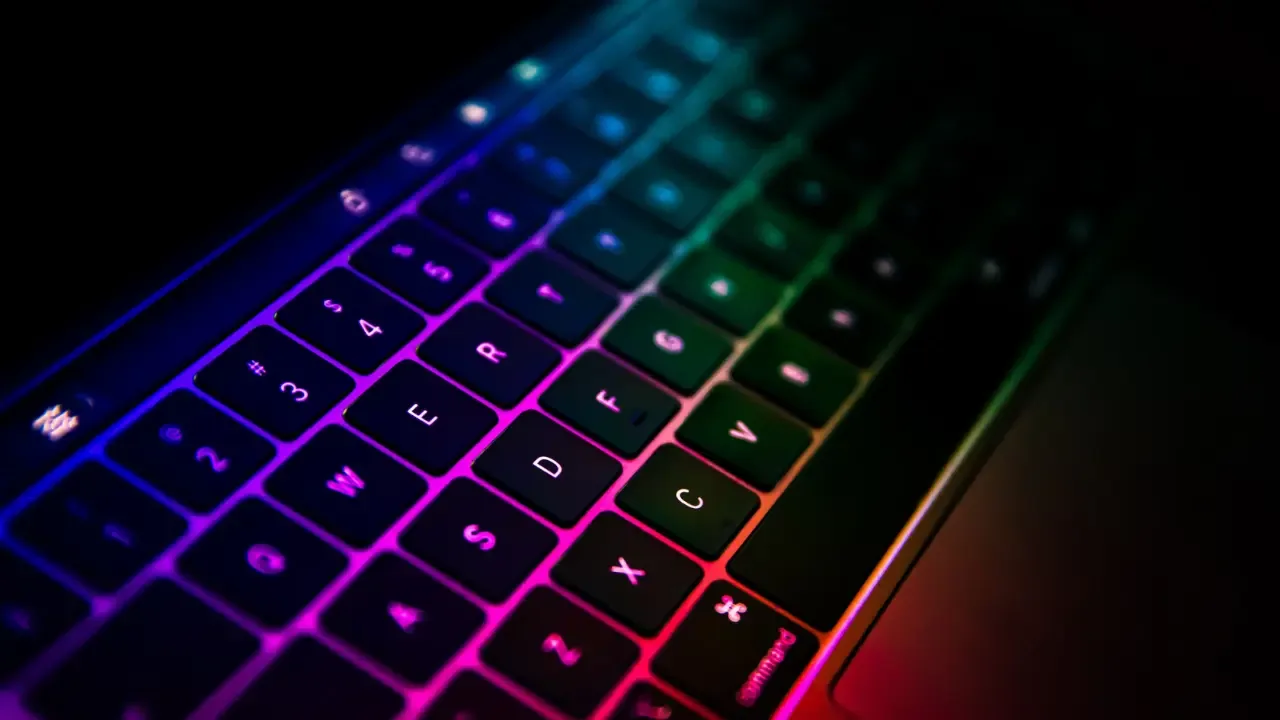
Mongoose Query: Finding Documents with a Non-null Value
Are you struggling to write a Mongoose query that selects documents where a specific field is not null? You're in the right place! In this blog post, we'll tackle this common issue and provide you with simple and effective solutions. 🙌
The Issue: Selecting Documents with a Non-null Field
Let's take a look at the context that led to this question:
Entrant
.find()
.where('enterDate').equals(oneMonthAgo)
.where('confirmed').equals(true)
.where('pincode.length > 0')
.exec((err, entrants) => {
// Handle the results here
});
The above code attempts to find documents satisfying three conditions: enterDate
equals oneMonthAgo
, confirmed
is true
, and pincode
has a length greater than zero. However, the question arises: is the where
clause properly written to select documents where pincode
is not null?
The Solution: Querying Non-null Values
Unfortunately, the where
clause used in the provided code snippet doesn't directly address the requirement of selecting non-null values. Instead, it checks if the length of pincode
is greater than zero.
To fix this, we'll introduce a better approach. We'll utilize the $ne
operator, which stands for "not equals." We can compare the field against the JavaScript value null
using this operator.
Here's the updated code snippet:
Entrant
.find()
.where('enterDate').equals(oneMonthAgo)
.where('confirmed').equals(true)
.where('pincode').ne(null)
.exec((err, entrants) => {
// Handle the results here
});
By using .where('pincode').ne(null)
, we explicitly specify that we want the pincode
field to not be equal to null
. This allows us to select only documents where pincode
is not null.
Example: Demonstrating the Solution
To demonstrate the effectiveness of our solution, let's consider a specific scenario. Suppose we have the following two documents in our collection:
{
_id: 1,
enterDate: '2022-01-01',
confirmed: true,
pincode: '12345'
},
{
_id: 2,
enterDate: '2021-12-01',
confirmed: true,
pincode: null
}
By executing the updated Mongoose query, we will retrieve only the first document with _id: 1
, as it satisfies all our conditions, including the non-null pincode
requirement.
The Call-to-Action: Engage and Share!
Now that you have a clear solution to selecting documents with a non-null field in Mongoose, it's time to put it into practice! 🚀
Feel free to apply this technique to your own projects and share your experience in the comments section below. If you have any other Mongoose-related questions or topics you'd like us to cover, let us know as well!
Happy coding! 👩💻👨💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
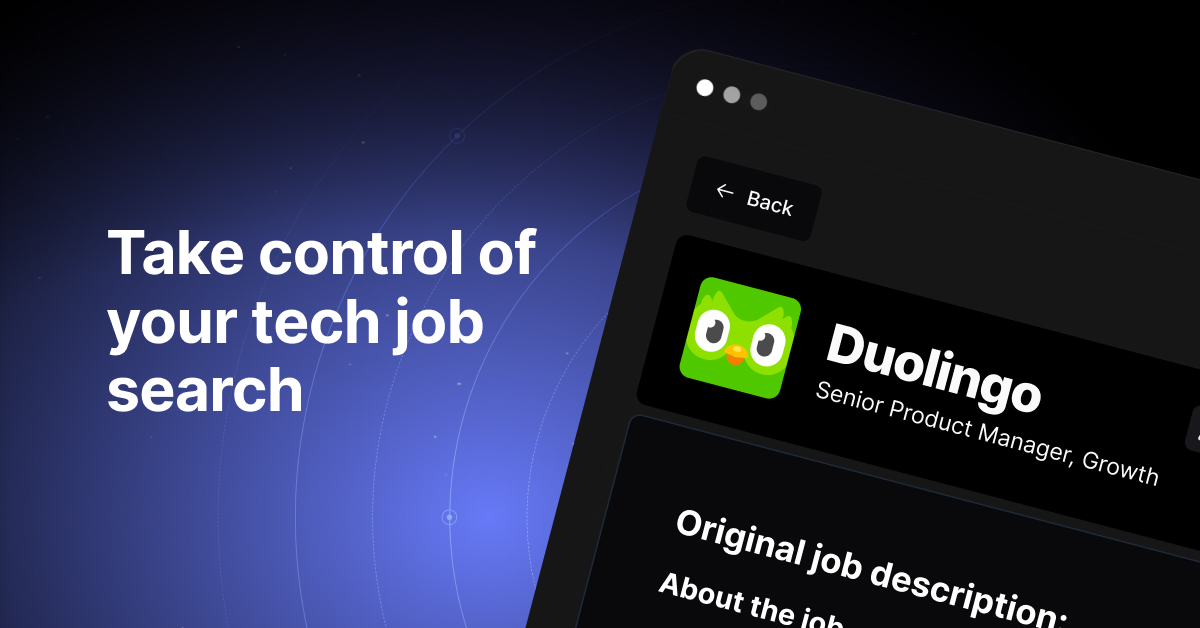