Mongoose -- Force collection name
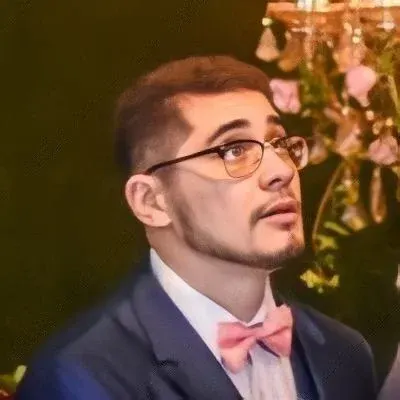
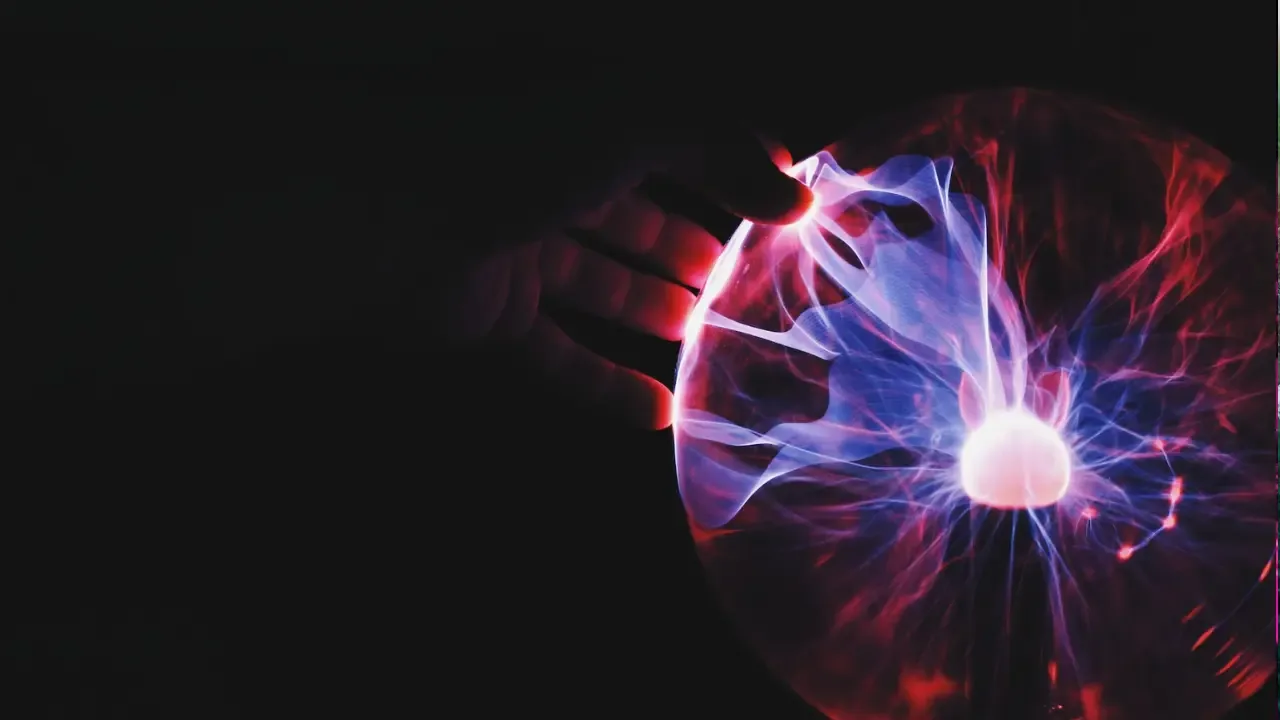
š Blog Post Title: "Mongoose: How to Force Collection Naming in MongoDB?"
š Introduction: So you're using Mongoose to create a database and a collection in MongoDB, but you've run into a snag. Instead of the collection name being "userinfo," it's being created as "UserInfo" instead. Fear not, because in this blog post, we'll explore common issues with collection naming in Mongoose and provide you with easy solutions to force the collection name you desire.
ā”ļø Problem:
The issue you're facing is that Mongoose, by default, takes the name of the model you assign using mongoose.model()
and pluralizes it to create the collection name. In your case, the model name is "UserInfo," and MongoDB automatically creates a collection called "UserInfo" instead of "userinfo."
ā Solution: To force a specific collection name in Mongoose, you can opt for either of the two approaches below:
1ļøā£ Approach 1: Manually Specify the Collection Name You have the option to manually specify the collection name when defining your schema. Here's an example of how you can modify your code to achieve this:
var mongoose = require('mongoose');
var db = mongoose.connect('mongodb://localhost/testdb');
var Schema = mongoose.Schema;
var UserInfo = new Schema({
username: String,
password: String
}, { collection: 'userinfo' }); // Specify the desired collection name
mongoose.model('UserInfo', UserInfo);
var user = db.model('UserInfo');
var admin = new user();
admin.username = "sss";
admin.password = "ee";
admin.save();
By adding { collection: 'userinfo' }
as the second argument to your schema definition, you can explicitly set the desired collection name to "userinfo."
2ļøā£ Approach 2: Use Mongoose Plugins Another way to force collection naming is by utilizing Mongoose plugins. Here's an example:
var mongoose = require('mongoose');
var db = mongoose.connect('mongodb://localhost/testdb');
var Schema = mongoose.Schema;
var UserInfo = new Schema({
username: String,
password: String
});
UserInfo.set('collection', 'userinfo'); // Specify the desired collection name using set()
mongoose.model('UserInfo', UserInfo);
var user = db.model('UserInfo');
var admin = new user();
admin.username = "sss";
admin.password = "ee";
admin.save();
By calling .set('collection', 'userinfo')
on your schema object, you can achieve the same result of forcing the collection name to be "userinfo."
š Call-to-Action: We hope this guide has helped you understand how to force collection naming in Mongoose. Try out the solutions provided and let us know in the comments if you encountered any issues or have any other questions related to Mongoose or MongoDB. Share this blog post with others who might find it helpful and spread the knowledge!
ā”ļø Always keep exploring and happy coding! š»āØ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
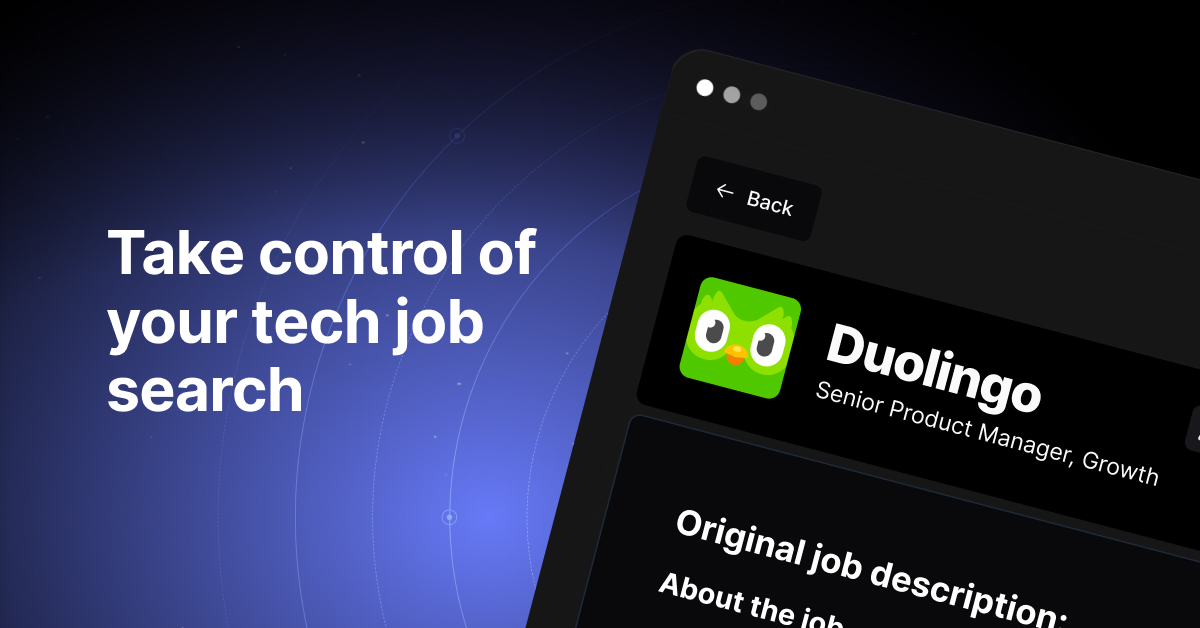