MongoDB: update every document on one field
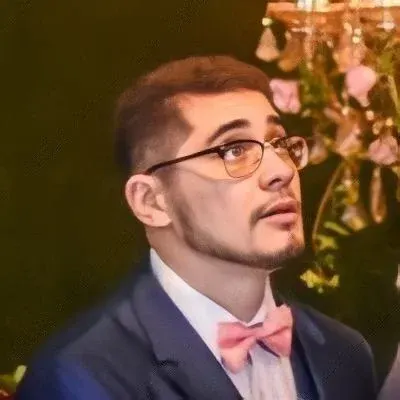
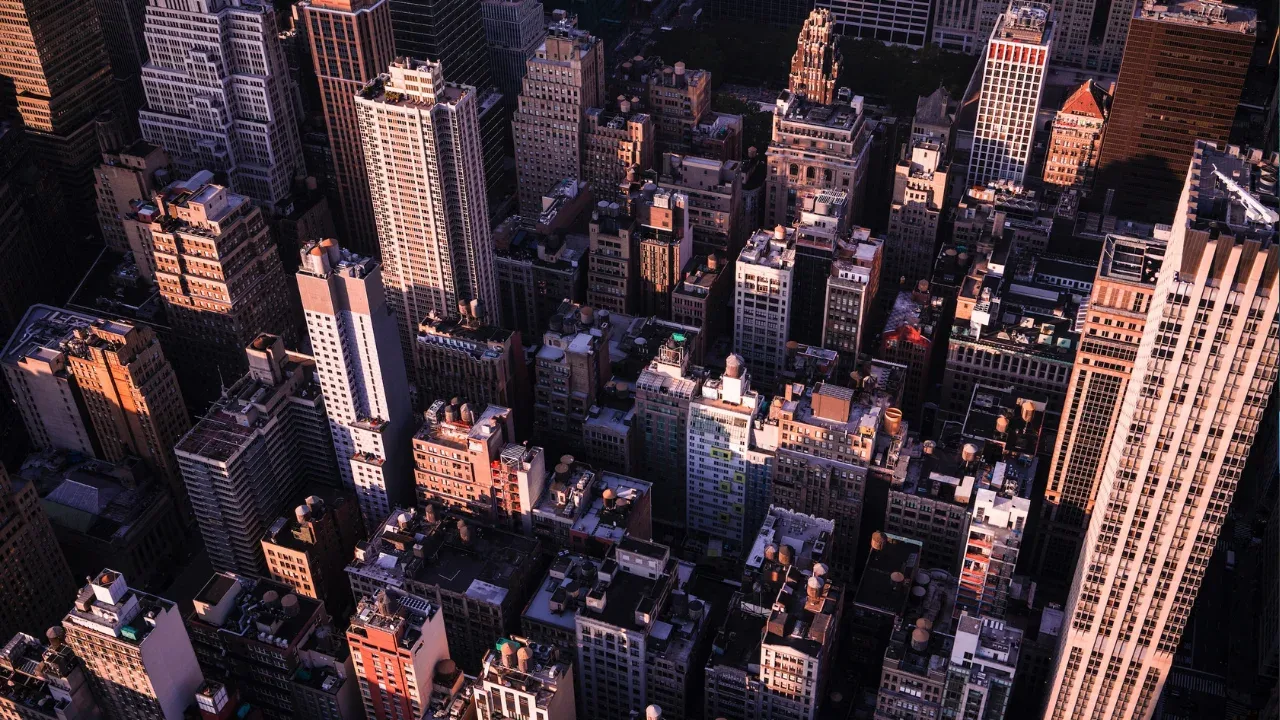
Updating Every Document on One Field in MongoDB: Easy Solutions for a Common Problem š©āš»š”
š Hey there, tech enthusiasts! Are you working with MongoDB and have stumbled upon the question of how to update every document on one specific field? You're not alone! Many developers face the same dilemma when dealing with large datasets. But don't worry, we're here to provide you with easy solutions to this common problem. Let's dive right in! šŖ
The Context š
One of our readers, let's call them DevKing, reached out to us with the following scenario:
"I have a collection named
foo
, hypothetically speaking. Each instance offoo
has a field calledlastLookedAt
, which is a UNIX timestamp since epoch. I'd like to be able to go through the MongoDB client and set that timestamp for all existing documents (about 20,000 of them) to the current timestamp. What's the best way of handling this?"
The Challenge š¤
DevKing wants to update the lastLookedAt
field for every document in the foo
collection. With 20,000 documents at hand, manually updating each one is simply not feasible. So, how can DevKing tackle this challenge efficiently?
Solution 1: Using the MongoDB Shell š»
š MongoDB provides a powerful command-line interface called the MongoDB Shell. This tool allows you to interact with MongoDB databases programmatically.
Open the MongoDB Shell in your command-line interface (CLI) by typing
mongo
.Switch to the relevant database by executing the command
use yourDatabaseName
.Execute the update command to set the
lastLookedAt
field for all documents to the current timestamp:
db.foo.updateMany({}, { $set: { lastLookedAt: new Date() } })
db.foo
specifies the collection name. Replacefoo
with the actual name of your collection.{}
as the first argument matches all documents in the collection.$set
is a MongoDB operator used to update fields.{ lastLookedAt: new Date() }
specifies the new value for thelastLookedAt
field, which is the current timestamp.
That's it! All documents in the foo
collection will now have their lastLookedAt
field set to the current timestamp.
Solution 2: Using a MongoDB Driver in Your Preferred Programming Language š
š If you're more comfortable with writing code, you can leverage MongoDB drivers in your preferred programming language to update every document on the lastLookedAt
field programmatically.
Here's an example in JavaScript using the official MongoDB Node.js driver:
Install the MongoDB Node.js driver by running
npm install mongodb
in your project directory.Establish a connection to your MongoDB database using the driver:
const { MongoClient } = require('mongodb');
async function updateTimestamp() {
const uri = 'mongodb://localhost:27017';
const client = new MongoClient(uri);
try {
await client.connect();
const collection = client.db('yourDatabaseName').collection('foo');
// Update all documents
const result = await collection.updateMany({}, { $set: { lastLookedAt: new Date() } });
console.log(`${result.modifiedCount} documents updated.`);
} finally {
await client.close();
}
}
updateTimestamp().catch(console.error);
Make sure to replace
'mongodb://localhost:27017'
with the appropriate MongoDB connection string.Modify
'yourDatabaseName'
and'foo'
with your actual database and collection names.
The Call-to-Action š¬
Congratulations! You've learned two straightforward ways to update every document on a specific field in MongoDB. Give them a try and see which solution works best for your project.
Feel free to comment below and let us know your experience! Have you encountered any challenges or found alternative approaches? We'd love to hear your thoughts. Let's keep the discussion going! š
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
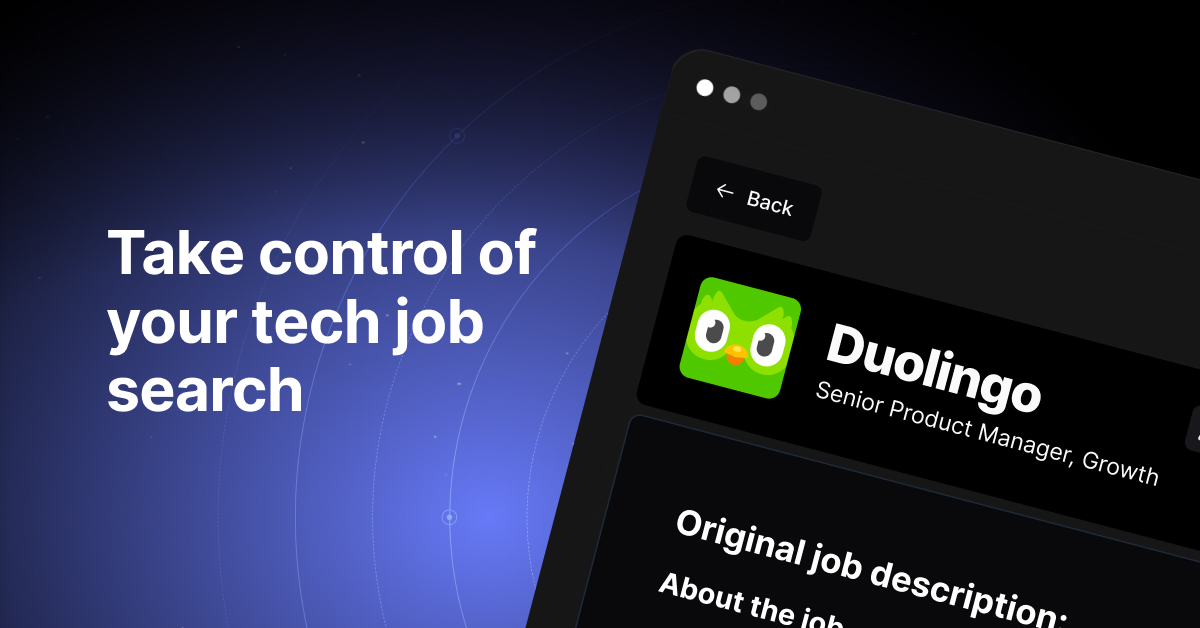