How to update the _id of one MongoDB Document?
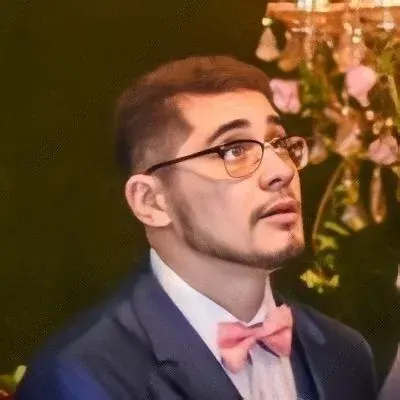
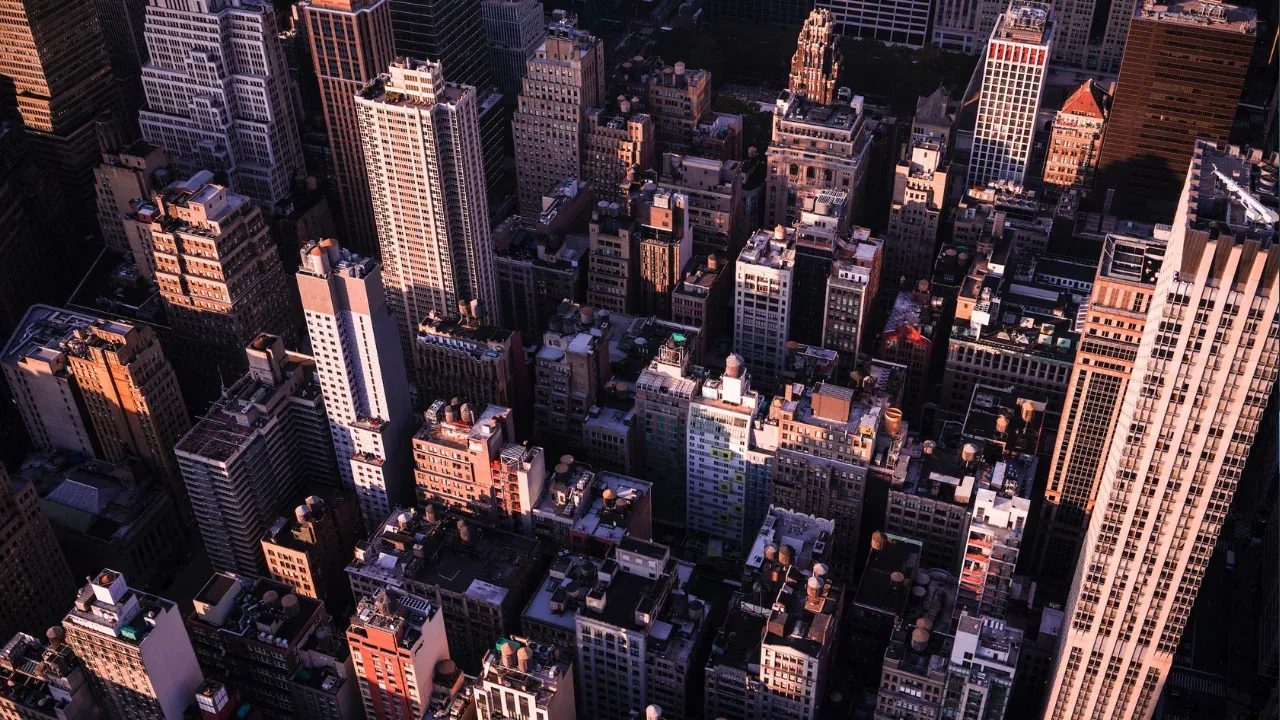
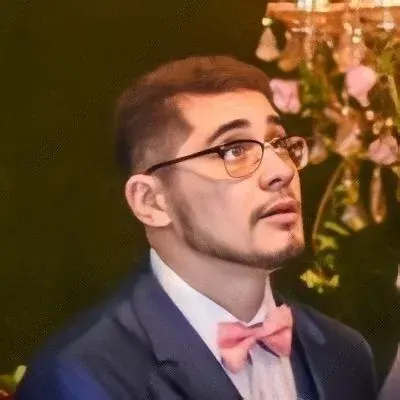
How to update the _id
of one MongoDB Document? 🔄
So you find yourself in need of updating the _id
field of a MongoDB document? While it might not be the best practice, sometimes certain technical requirements might push you to make such updates. Let's go through the process of updating the _id
field, addressing common issues and providing easy solutions along the way. Hang on tight, because we're about to dive into the 🌊 of MongoDB.
The Problem: Rejected Updates ❌
When you attempt to update the _id
field using a query like this:
db.clients.update({ _id: ObjectId("123")}, { $set: { _id: ObjectId("456")}})
You receive the following error message 🗯️:
Performing an update on the path '_id' would modify the immutable field '_id'
And sadly, your update is rejected. But fret not, as we have a few workarounds to help you overcome this hurdle.
Solution 1: Removing the Existing Document and Inserting a New One 🗑️
Sometimes the simplest solution is also the most effective. In this case, you can remove the document with the old _id
and insert a new document with the updated _id
value. Here's how you can achieve this using the MongoDB shell:
Find the document you want to update using the existing
_id
:db.clients.findOne({ _id: ObjectId("123") })
Once you have confirmed that this is indeed the document you wish to update, remove it using the following command:
db.clients.remove({ _id: ObjectId("123") })
Finally, insert a new document with the updated
_id
:db.clients.insert({ _id: ObjectId("456"), ... })
Keep in mind that the ...
represents the rest of the fields and values you want to include in the new document.
Solution 2: Using Aggregation to Reconstruct the Document 🔄
If removing and inserting new documents is not a feasible option, you can use an aggregation pipeline to reconstruct the document with the updated _id
. Here's how you can do it:
db.clients.aggregate([
{ $match: { _id: ObjectId("123") } },
{ $addFields: { newId: ObjectId("456") } },
{ $unset: "_id" },
{ $merge: { into: "clients", on: "newId" } }
])
Let's break down what's happening in this aggregation pipeline:
The
$match
stage filters for the document with the old_id
.The
$addFields
stage adds a new field callednewId
with the updated_id
value.The
$unset
stage removes the existing_id
field.The
$merge
stage merges the resulting document with the rest of theclients
collection using thenewId
field.
Time to Level Up Your MongoDB Game! ⚡️
Updating the _id
field of a MongoDB document might not be the norm, but with these solutions up your sleeve, you can confidently tackle this challenge when it arises. Remember to exercise caution when performing such updates, as it can have implications on your application's data integrity.
Now it's time for you to dive into your MongoDB playground and give these solutions a try. Let us know how it goes! Have you encountered any other MongoDB challenges? Share them in the comments below and let's help each other level up our MongoDB skills. 🚀