How to listen for changes to a MongoDB collection?
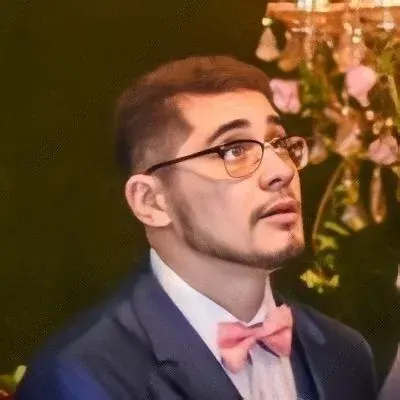
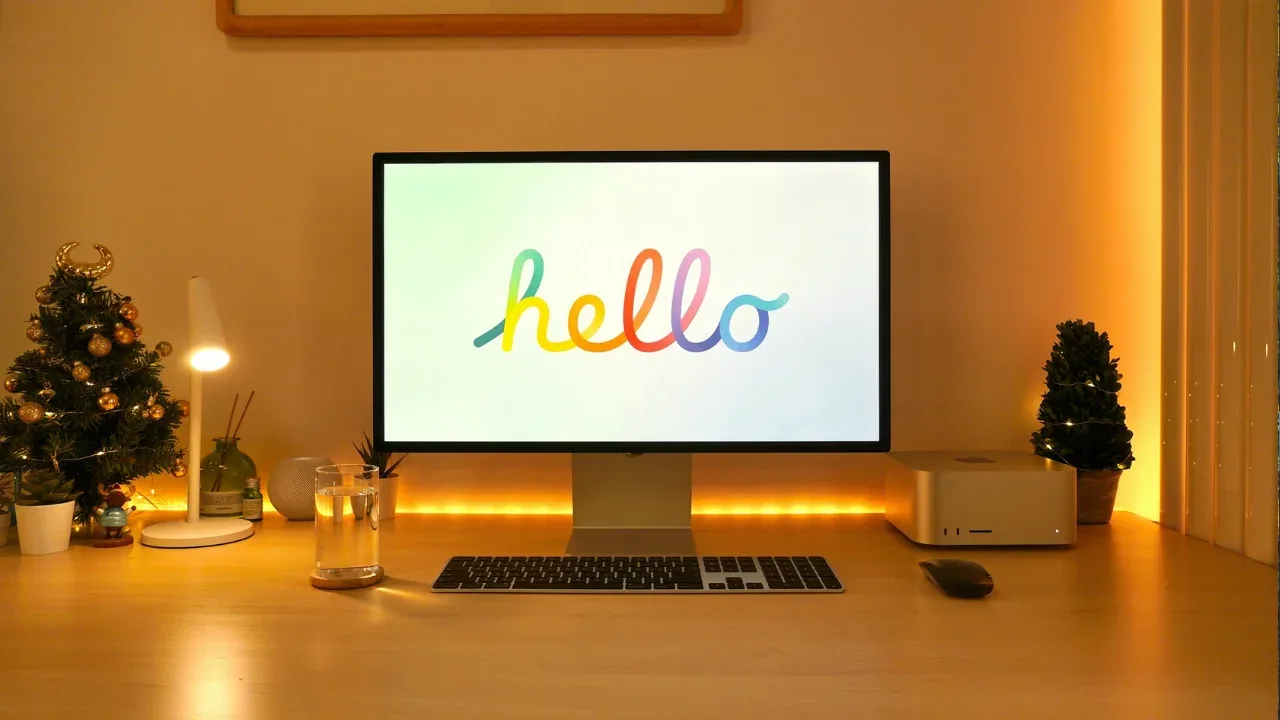
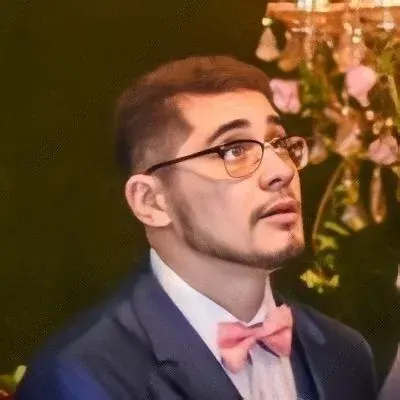
📢 Hey tech enthusiasts! Are you looking for an efficient way to listen for changes to a MongoDB collection? 🤔 Well, you're in luck! Today, we'll be diving into this exciting topic and exploring easy solutions to help you keep track of those inserts. So, let's get started! 💪
The Challenge: Imagine you're building a background job queue system using MongoDB as your data store. Your goal is to listen for inserts to a MongoDB collection and spawn workers to process the job as soon as a new document is added. But the question is, how do you achieve this without constantly polling the database or wasting resources? 🤔
The Solution: Luckily, MongoDB provides a simple yet powerful solution to address this challenge - Change Streams. Change Streams allow you to open a real-time stream of data changes at the collection level. You can then listen for specific events, such as inserts, updates, or deletions, and take immediate action when changes occur. 🔄✨
How to Implement Change Streams in PHP: Let's address the PHP aspect of your project, but don't worry, the concepts can be applied to other programming languages as well. Here's a step-by-step guide to get you started:
Ensure MongoDB PHP Driver Extension: Before diving into Change Streams, make sure you have the MongoDB PHP driver extension installed and loaded in your PHP environment. You can check the official MongoDB documentation for detailed instructions.
Connecting to MongoDB: Establish a connection to your MongoDB server using the MongoDB\Driver\Manager class. This manager will act as the entry point for working with the database.
<?php $manager = new MongoDB\Driver\Manager("mongodb://localhost:27017");
Opening a Change Stream: Use the MongoDB\Driver\Manager class to open a change stream on the desired collection. Specify any additional options for filtering or customization. In our case, we want to listen for inserts, so we will filter for "insert" operations.
<?php $pipeline = []; $options = ['fullDocument' => 'updateLookup']; $changeStream = $manager->watch($pipeline, $options);
Listening for Changes: Now that we have our change stream, we can start listening for changes. Use a loop to continuously check for new events, and handle each event as it arrives.
<?php foreach ($changeStream as $change) { // Handle the change event $operationType = $change->operationType; $fullDocument = $change->fullDocument; // Perform your desired action based on the event if ($operationType == 'insert') { // Spawn worker to process the job // ... your code here ... } }
And there you have it! By using Change Streams in your PHP project, you can listen for inserts to your MongoDB collection without the need for constant polling or wasting resources. Now, let's take it one step further! 🚀
Level Up Your Project: Take advantage of this opportunity to optimize your background job queue system even further. You can consider the following enhancements to make your system more robust and efficient:
Implement error handling and retry mechanisms for worker processes.
Leverage multi-threading or process management techniques to handle multiple workers simultaneously.
Integrate this solution with your favorite queueing system (e.g., RabbitMQ, Redis) for a more scalable design.
Your Turn to Shine! 💫 Now that you know about Change Streams and how to implement them in your PHP project, it's time to put your newfound knowledge into action! Share your thoughts, experiences, and any interesting use cases in the comments section below. Let's spark some engaging conversations and learn from each other! 🗣️💡
That's all for now, techies! We hope you found this guide helpful and inspiring. Remember, listening for changes to a MongoDB collection doesn't have to be complicated or resource-intensive. With Change Streams, you can make your project more efficient and responsive. So go ahead, give it a try, and keep those inserts in check! 🎯🔍🔄