How to get the last N records in mongodb?
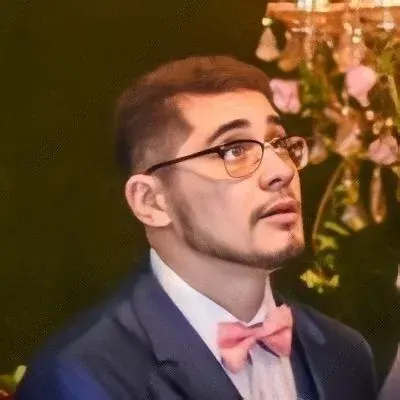
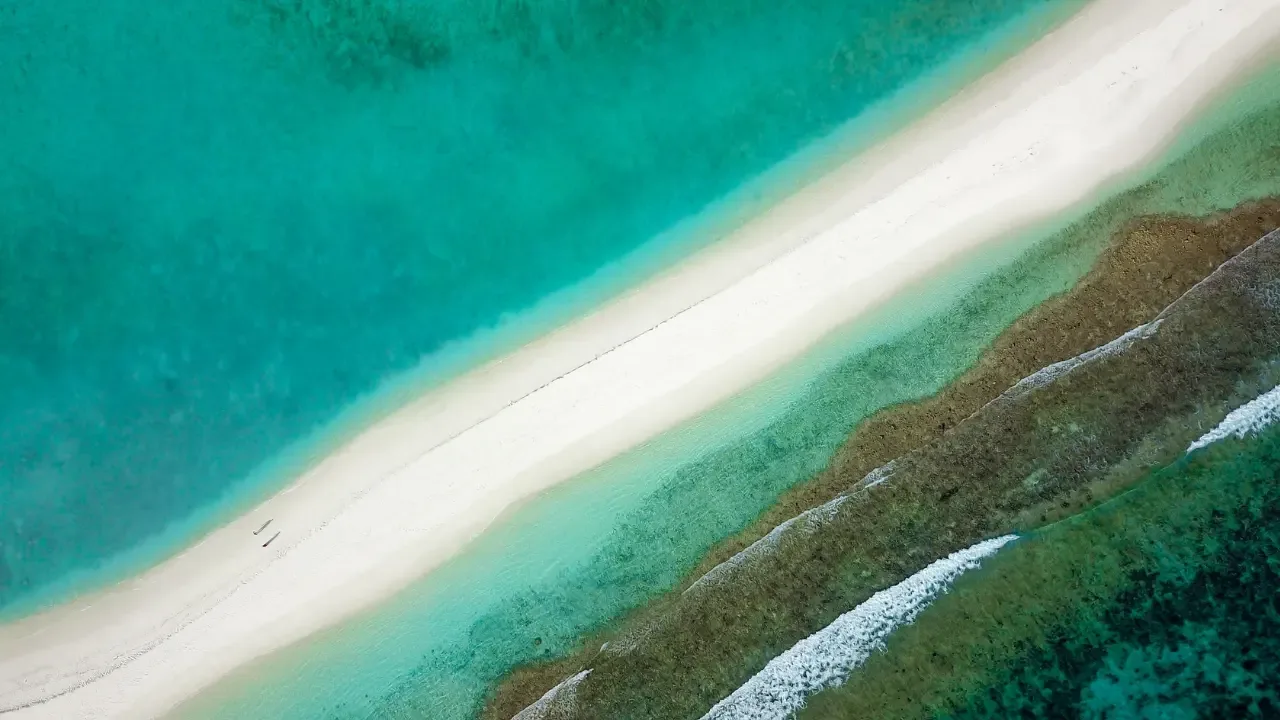
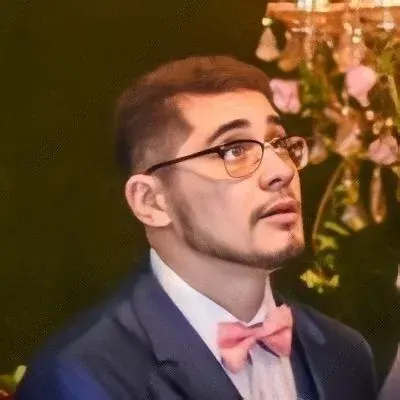
📜 MongoDB: How to Get the Last N Records
Hey there, tech enthusiasts! 👋 Are you struggling with getting the last N records in MongoDB? Don't worry, you're not alone! This is a common issue that many developers face, but fear not - I've got you covered! In this blog post, I'll walk you through easy solutions and provide code examples to help you retrieve the desired data. So, let's dive right in! 💪
The Problem 🤔
By default, the MongoDB find()
operation retrieves records from the beginning, making it a bit tricky to fetch the last N records. Additionally, if you want the result ordered from less recent to most recent, you're in for an extra challenge. That's a lot to tackle, but don't fret! I've got some amazing solutions for you. 💡
Solution 1: Use sort()
and limit()
🗂️
The first approach involves combining the sort()
and limit()
methods to fetch the last N records:
db.collection.find().sort({ _id: -1 }).limit(N)
In the above code snippet, replace collection
with the actual name of your collection and N
with the number of records you wish to retrieve. By sorting the records in descending order based on their unique _id
using -1
, we ensure that the most recent entries appear first. The limit()
method restricts the result to the specified number, giving you the last N records you desire. 📃
Solution 2: Utilize count()
and skip()
😎
Another approach involves using count()
to determine the total number of records, and then applying skip()
to retrieve the required documents:
const totalCount = db.collection.count();
const skipCount = totalCount - N;
db.collection.find().skip(skipCount);
In the code snippet above, we first calculate totalCount
by using the count()
function on the collection. We then compute the skipCount
by subtracting the desired number of records (N
) from the totalCount
. Finally, by utilizing skip()
in conjunction with find()
, we can retrieve the last N records without explicitly sorting them. 🧮
Solution 3: Leverage aggregate()
🔄
If you're seeking a more robust solution, aggregate()
is here to save the day! We can leverage the $sort
and $limit
aggregation operators to fetch the last N records:
db.collection.aggregate([
{ $sort: { _id: -1 } },
{ $limit: N }
]);
In the code snippet above, the aggregation pipeline utilizes $sort
to sort the records based on their _id
in descending order. Next, $limit
is applied, restricting the result to the specified number (N
) and giving us our desired output. 🌪️
Engage and share your experience! 📣
There you have it! Three simple yet effective solutions to get the last N records in MongoDB. Give them a try, and let me know your thoughts! Have any other strategies or questions? Share your experience in the comments section below. Your feedback could help fellow developers facing similar challenges! 👇
Keep exploring, keep coding! 💻✨