How to filter array in subdocument with MongoDB
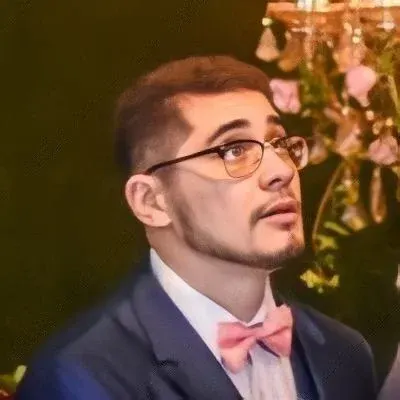
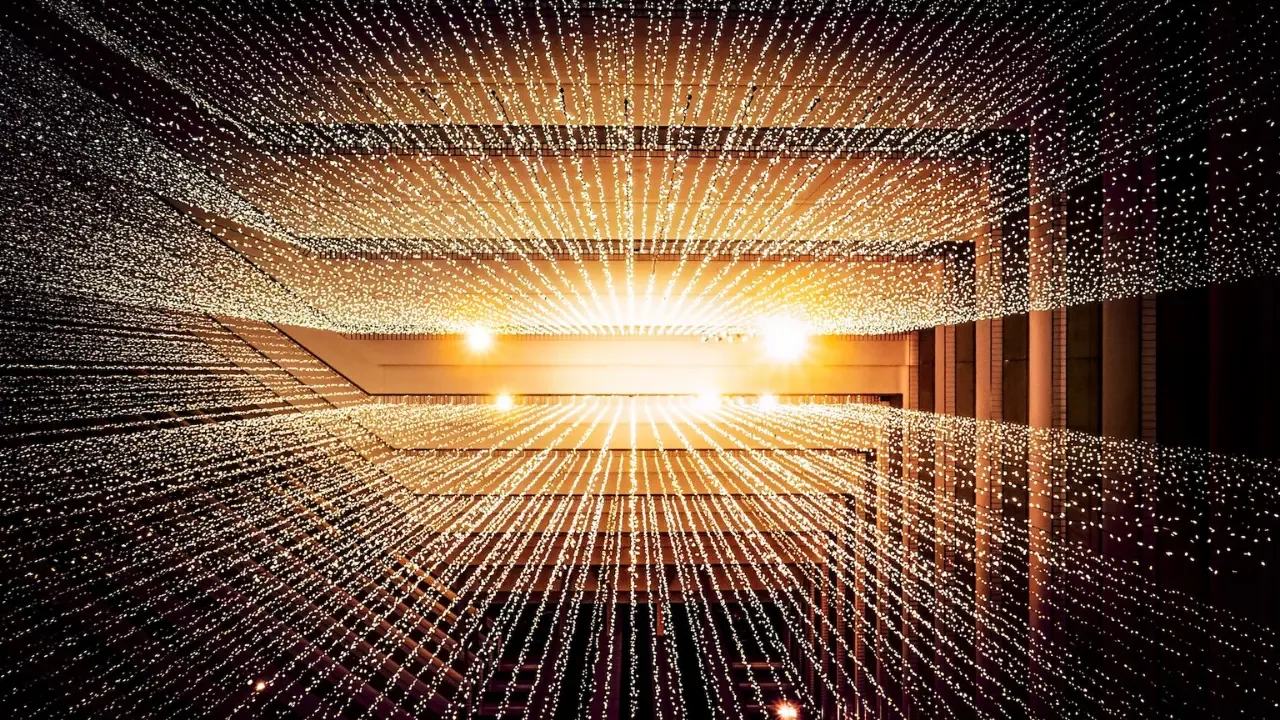
How to Filter Array in Subdocument with MongoDB 😎
Are you struggling to filter an array within a subdocument using MongoDB? Don't worry, we've got you covered! In this guide, we will address common issues faced when filtering subdocuments in MongoDB arrays and provide easy and effective solutions to help you achieve your desired results. 💪
The Problem 😕
Let's start by understanding the problem at hand. Imagine you have a document with an array in a subdocument like this:
{
"_id" : ObjectId("512e28984815cbfcb21646a7"),
"list" : [
{
"a" : 1
},
{
"a" : 2
},
{
"a" : 3
},
{
"a" : 4
},
{
"a" : 5
}
]
}
You want to filter this subdocument to retrieve only the elements where the value of "a"
is greater than 3. The expected result should look like this:
{
"_id" : ObjectId("512e28984815cbfcb21646a7"),
"list" : [
{
"a" : 4
},
{
"a" : 5
}
]
}
Solution 1: Using $elemMatch 🧩
Your initial attempt might be to use the $elemMatch
operator in your query. However, this approach returns only the first matching element in the array. Let's take a look at your query and its result:
db.test.find(
{
"_id" : ObjectId("512e28984815cbfcb21646a7")
},
{
list: {
$elemMatch: {
a: {
$gt: 3
}
}
}
}
)
Result:
{
"_id" : ObjectId("512e28984815cbfcb21646a7"),
"list" : [
{
"a" : 4
}
]
}
As you can see, it only returns the first matching element instead of all elements greater than 3.
Solution 2: Using Aggregation Pipeline with $match 🔄
To achieve the desired result, you can use the aggregation framework in MongoDB along with the $match
stage. Let's modify your query to use the $match
stage:
db.test.aggregate([
{
$match: {
"_id": ObjectId("512e28984815cbfcb21646a7"),
"list.a": { $gt: 3 }
}
}
])
Result:
{
"_id" : ObjectId("512e28984815cbfcb21646a7"),
"list" : [
{
"a" : 4
},
{
"a" : 5
}
]
}
Now, the aggregation framework returns the expected result by filtering all elements greater than 3 within the array.
Conclusion 🎉
Filtering an array within a subdocument in MongoDB can be challenging, especially when you want to retrieve all matching elements. By using either the $elemMatch
operator or the aggregation framework with the $match
stage, you can easily overcome this problem and achieve your desired results.
Don't hesitate to try out different approaches and explore the power of MongoDB to solve various data filtering challenges.
If you found this guide helpful or have any further questions, feel free to leave a comment below. Happy coding! 😄👨💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
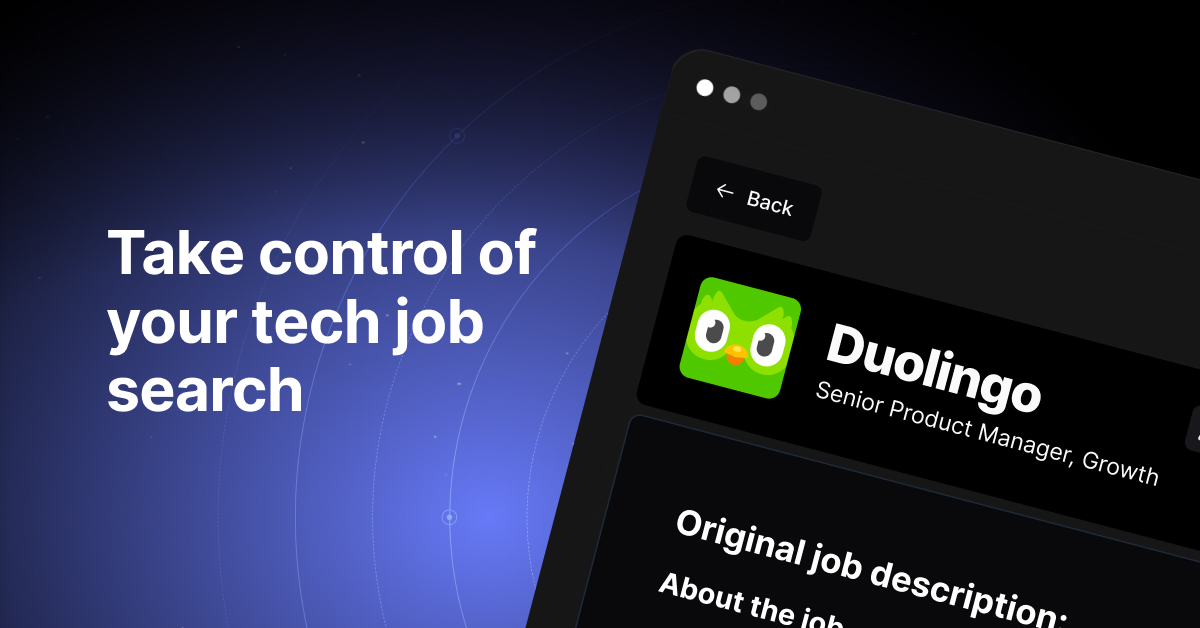