How to access a preexisting collection with Mongoose?
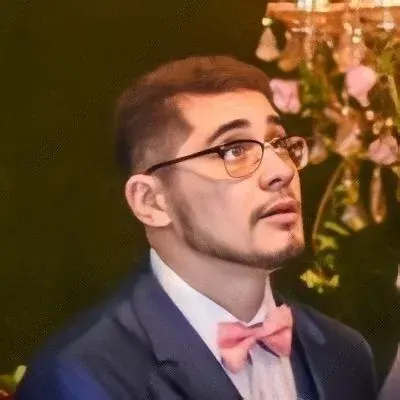
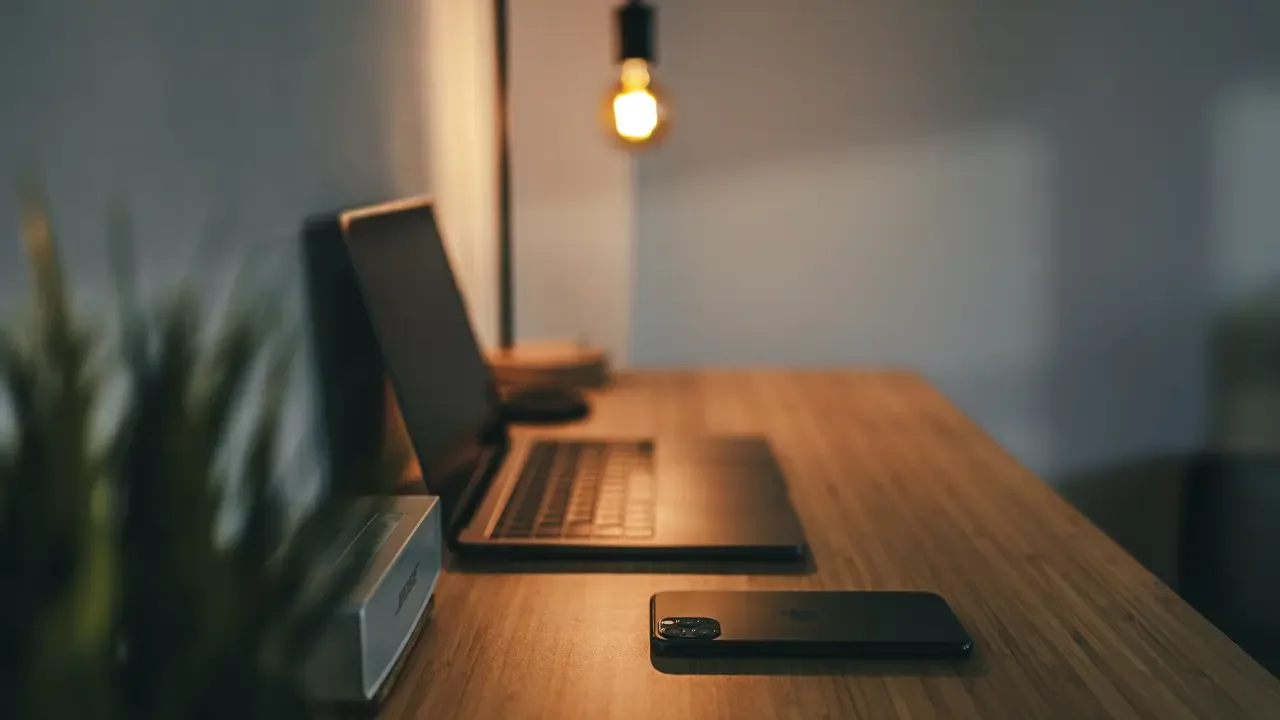
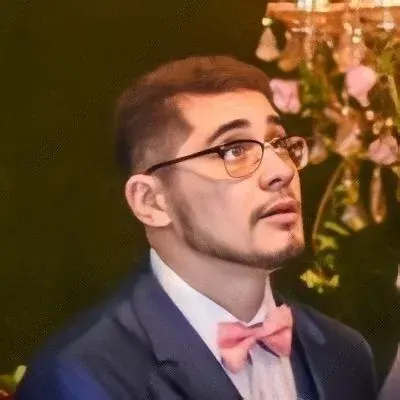
ššš¢ How to access a preexisting collection with Mongoose? š¦š
Do you have a preexisting collection in MongoDB that you want to access using Mongoose in your Express.js application? š¤ Don't worry! We have the solution for you. š
š Understanding the Problem: By default, Mongoose doesn't automatically create a model for an existing collection. So, when you try to access an already existing collection, you may encounter an empty array as the result. This can be frustrating, especially if you have a large dataset that you want to use.
š” Easy Solution: To access an existing collection using Mongoose, you need to explicitly define a schema and model that matches the collection's structure. š In your code snippet, you're missing a vital step of specifying the existing collection name while creating the model.
Here's the modified code snippet to help you access the preexisting collection: š„ļø
var mongoose = require('mongoose');
var Schema = mongoose.Schema;
mongoose.connect('mongodb://localhost/test');
// Specify the existing collection name while creating the model
var questionSchema = Schema({ url: String, text: String, id: Number }, { collection: 'question' });
var questions = mongoose.model('question', questionSchema);
questions.find({}, function(err, data) { console.log(err, data, data.length); });
š Understanding the Solution:
In the modified code snippet, we define a questionSchema
using the Schema
constructor and explicitly mention the collection name as 'question'
using the collection
option. This ensures that Mongoose maps the model to the existing collection with the same name.
š» Example Explanation: Let's break down the modified code snippet to understand what's happening step by step:
We require
mongoose
and create aSchema
variable.Establish a connection to the MongoDB database using
mongoose.connect()
, passing the appropriate connection string.Create a schema for the preexisting collection, specifying the fields
'url'
,'text'
, and'id'
.Explicitly mention the collection name as
'question'
using thecollection
option while creating the schema.Create a model named
'question'
usingmongoose.model()
and pass the createdquestionSchema
.Use the
find()
method on thequestions
model to query the preexisting collection. The results will be logged to the console, including any potential errors encountered and the retrieved data.
š£ Call-to-Action: Now that you know how to access a preexisting collection with Mongoose, you can handle large datasets in your Express.js application like a pro! š If you found this guide helpful, don't forget to share it with your friends and fellow developers. Let us know if you have any questions or encounter any issues. Happy coding! āØš»
ššš¢ Happy Mongoose Data Accessing! š¦š