How do you use Mongoose without defining a schema?
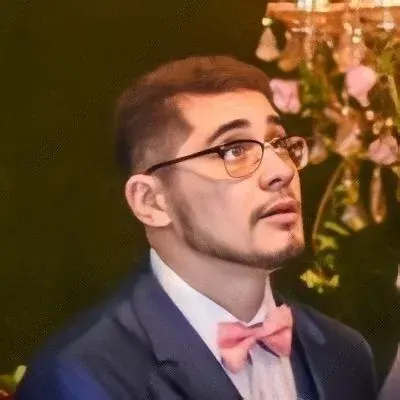
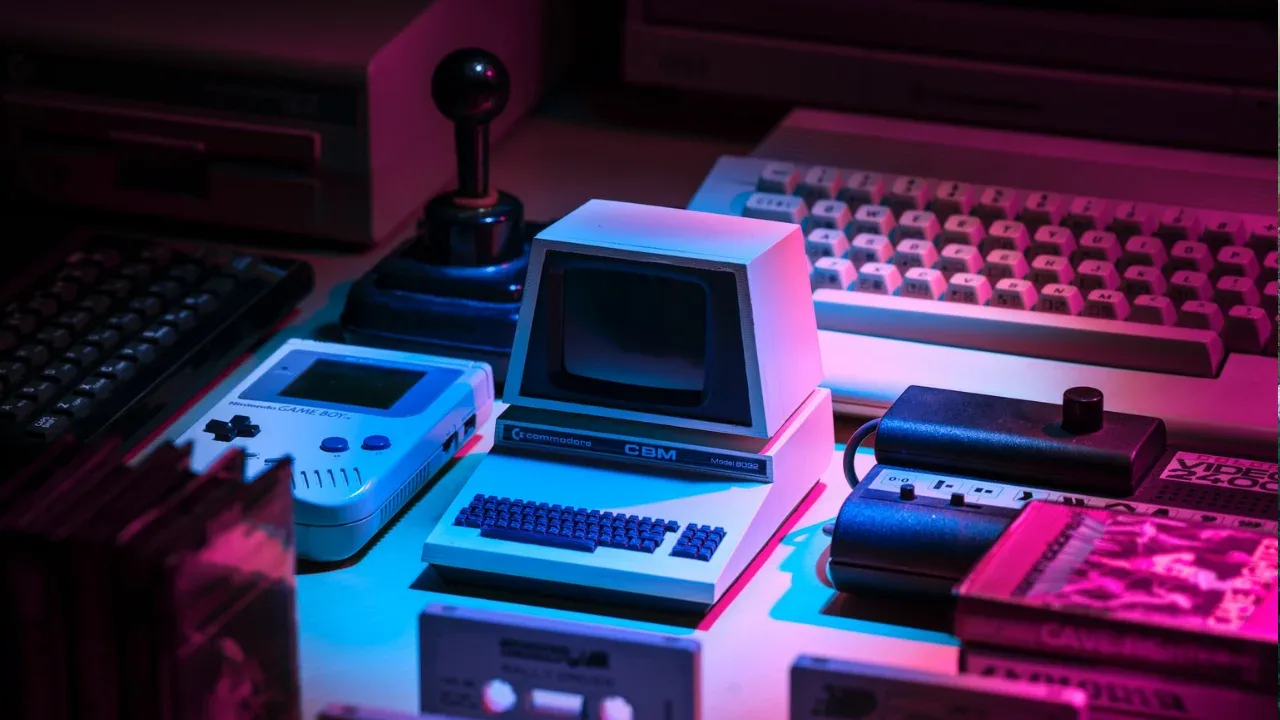
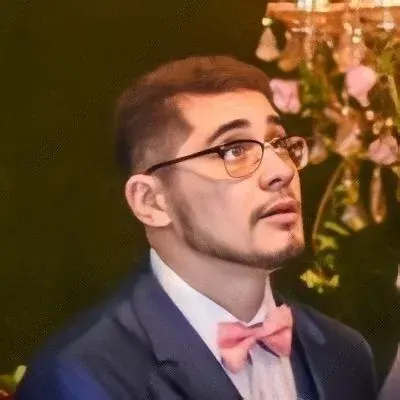
📝🦅 Using Mongoose without Defining a Schema: A Schema-Less Alternative 📝🦅
Are you tired of fitting your data into a predefined schema? Do you need a more flexible approach? Look no further! In this blog post, we'll explore how to use Mongoose without defining a schema and tackle the common issues you may encounter along the way. Get ready to revolutionize your Node.js development with Mongoose!
Understanding the Context
Before we dive into the solution, it's crucial to understand the context. In previous versions of Mongoose, there was an option to use it without defining a schema. Let's take a look at an example from the past:
var collection = mongoose.noSchema(db, "User");
This simple snippet allowed developers to work with dynamic and schema-less models in Mongoose. However, in the current version of Mongoose, this "noSchema" function has been removed, leaving users searching for an alternative solution.
The Need for Schema-less Models
Why would you want to use Mongoose without defining a schema? One common scenario is when your data structures change frequently and don't conform to a fixed schema. In such cases, the traditional schema-based approach can become cumbersome and limit the dynamic nature of your data model.
Whether you're working on a content management system, a social media platform, or any other application with constantly evolving data structures, having a schema-less alternative can greatly enhance your development experience.
Introducing Mongoose Schema-less Models
Fortunately, there is still a way to use Mongoose without defining a schema. Although the old "noSchema" function is gone, Mongoose provides a powerful feature for handling schema-less models: the Schema.Types.Mixed
data type.
Let's explore how you can leverage this feature to build flexible models. Consider the following example:
const mongoose = require('mongoose');
const dynamicSchema = new mongoose.Schema({},
{ strict: false }
);
const DynamicModel = mongoose.model('Dynamic', dynamicSchema);
🔍 Explanation:
First, we import the Mongoose library.
We create a new
dynamicSchema
by invokingmongoose.Schema
with an empty object as the schema definition. This empty object signifies that we are not enforcing any specific schema.The second argument of
mongoose.Schema
is an options object. By setting thestrict
flag tofalse
, we allow the schema-less model to accept any key-value pairs without throwing any validation errors.We create the "Dynamic" model using
mongoose.model
, passing in the desired model name ('Dynamic') and thedynamicSchema
.
🔧 Easy Solutions to Common Problems:
Adding Data: To add data to our schema-less model, we can simply create a new instance and set any arbitrary key-value pairs. For example:
const dynamicInstance = new DynamicModel();
dynamicInstance.firstName = 'John';
dynamicInstance.lastName = 'Doe';
dynamicInstance.save();
Querying Data: Querying becomes straightforward with schema-less models as you can search for documents based on any key-value pair. For instance:
DynamicModel.find({ age: { $gte: 18 } })
.then((results) => {
console.log(results);
})
.catch((error) => {
console.error(error);
});
💡 Pro Tip: If you want to take advantage of indexing, you can explicitly specify individual fields as indexable using the index: true
flag.
Engage and Share Your Experience!
Using Mongoose without defining a schema opens up a world of possibilities for working with dynamic data structures. Start experimenting with schema-less models in your Node.js projects today and share your experiences!
💌 If you encountered any challenges or have your own tips and tricks, please let us know in the comments section below. We love hearing from our readers!
🚀 Call-to-Action: Dive into the world of schema-less models and Mongoose. Check out our latest tutorial on schema-less modelling with Mongoose [link to tutorial]. Don't forget to subscribe for more interesting content and join our vibrant community of developers. Together, let's unlock new horizons in data flexibility!
Remember, in the world of Mongoose, schemas are no longer prisoners. Embrace the schema-less revolution!
🔗📚🔗