How do I perform the SQL Join equivalent in MongoDB?
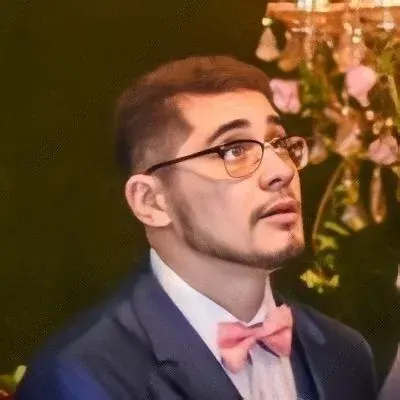
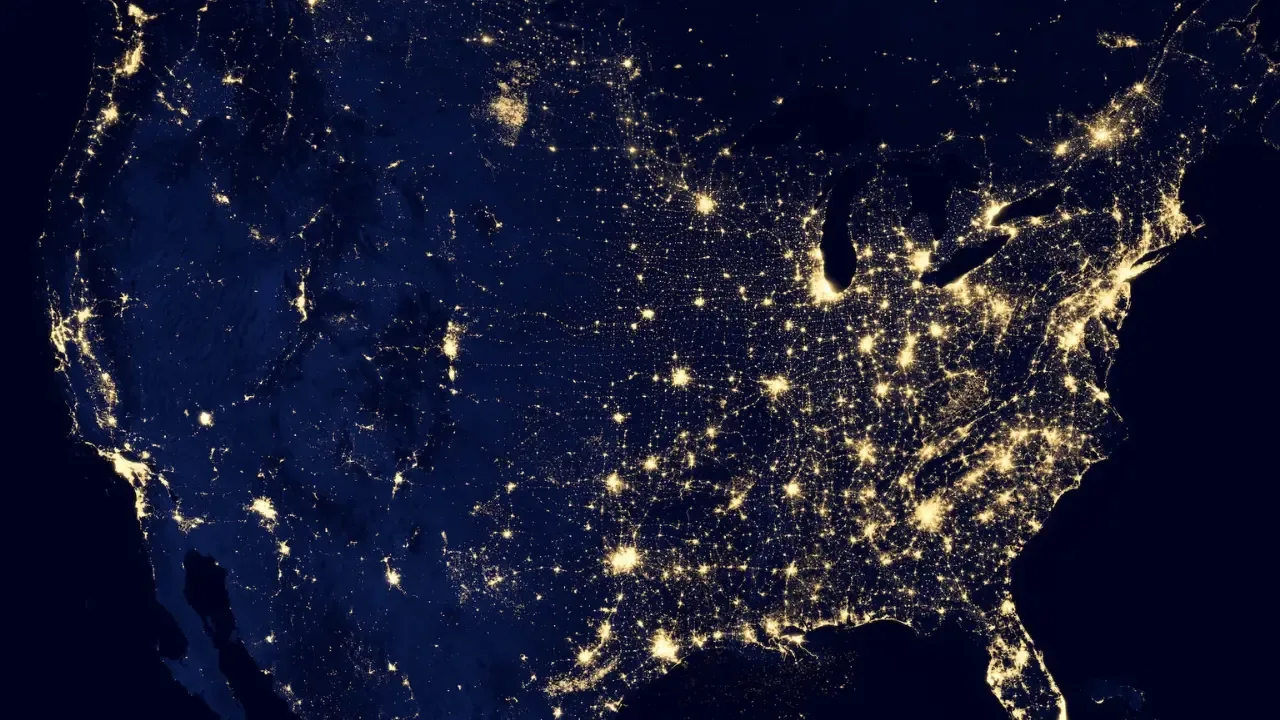
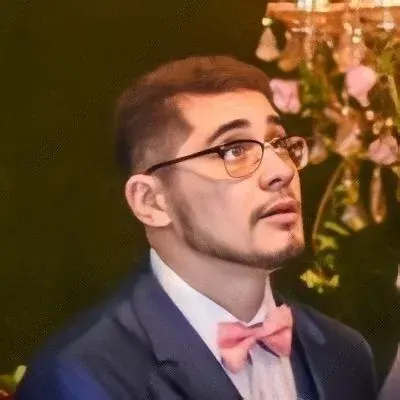
📝 How to Perform the SQL Join Equivalent in MongoDB: A Simplified Guide 🧩
Are you struggling to perform the SQL Join equivalent in MongoDB? Look no further! In this blog post, we will walk you through a simple and efficient solution to this common challenge. 💪
🔍 Understanding the Problem: Let's start with an example to give you a clear understanding of the problem at hand. Imagine you have two collections: "users" and "comments." You want to retrieve all the comments with pid=444 and also fetch the corresponding user information for each comment.
Here's an example of the collections:
comments:
{ uid:12345, pid:444, comment="blah" }
{ uid:12345, pid:888, comment="asdf" }
{ uid:99999, pid:444, comment="qwer" }
users:
{ uid:12345, name:"john" }
{ uid:99999, name:"mia" }
🤔 The Challenge:
The question is whether there's a way to extract all comments with a certain field (e.g., ...find({pid:444})
) along with the associated user information in one go. The current approach involves fetching the comments matching the criteria, identifying the uids within the result set, retrieving the corresponding user objects, and then merging the data. However, this feels cumbersome and inefficient. There must be a better way! 🤷♂️
🎉 The Solution: Fortunately, MongoDB provides a powerful feature called "aggregation" that can help us solve this problem with ease. The aggregation framework allows us to perform complex data operations, including joining data from multiple collections.
To achieve the desired result, we can utilize MongoDB's $lookup
operator. This operator performs a left outer join between two collections and retrieves the matching documents based on a specified condition.
Here's an example of how to use the $lookup
operator to solve our problem:
db.comments.aggregate([
{
$match: { pid: 444 } // Filter comments where pid is 444
},
{
$lookup: {
from: "users", // Specify the collection to join
localField: "uid", // Field from comments collection
foreignField: "uid", // Field from users collection
as: "user" // Name of the output array
}
}
])
🚀 Explanation:
We start by using the
$match
stage to filter the comments collection based on the pid field. This ensures that we only retrieve comments where pid equals 444.Next, we use the
$lookup
stage to join the comments collection with the users collection. We specify the collection name using thefrom
keyword.The
localField
parameter indicates the field in the comments collection that we want to match with theforeignField
in the users collection. In this case, we match theuid
field.The
as
parameter sets the name of the output array that will contain the user information associated with each comment. We chose "user" as the array name.Voila! 🎉 The output of the aggregation pipeline will contain all the comments with pid=444 along with their respective user information, neatly presented in an array.
💡 Pro Tip:
To make the output more manageable, you can further refine the aggregation pipeline by projecting only the necessary fields from both the comments and users collections. This can be done using the $project
stage.
🔔 Call-to-Action: We hope this guide has helped you solve the challenge of performing SQL Join equivalent in MongoDB. Now you can join data from multiple collections effortlessly, leveraging the power of the aggregation framework.
If you have any questions or want to share your experience, please leave a comment below. Join our community of tech enthusiasts who love to explore the latest technologies and simplify complex problems. 💬👥
Happy coding! 💻✨