How do I partially update an object in MongoDB so the new object will overlay / merge with the existing one
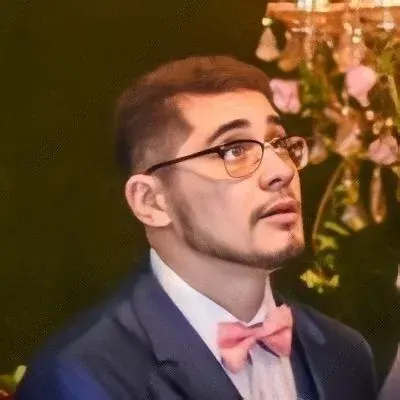
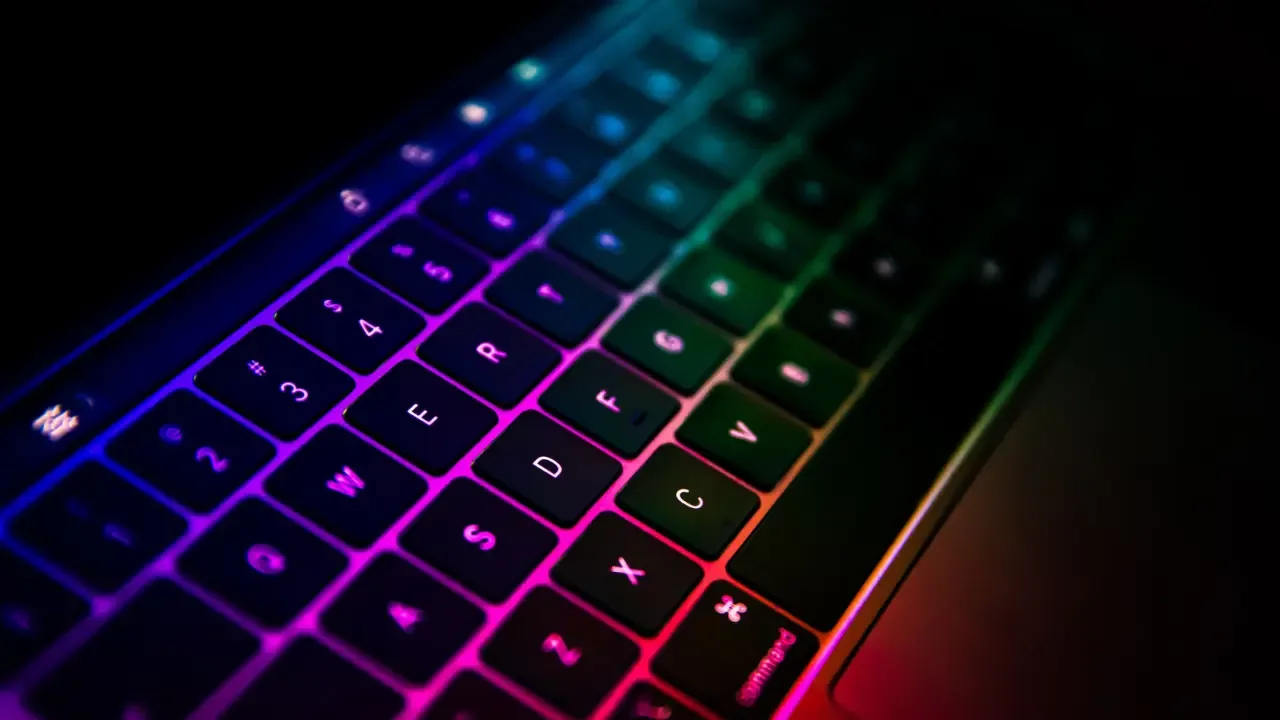
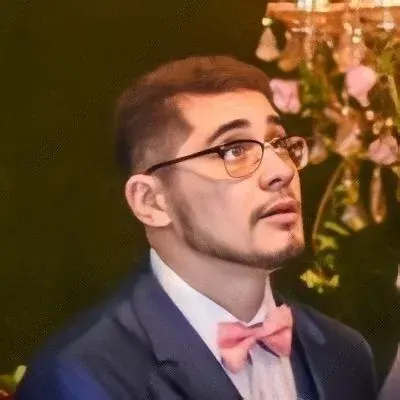
How to Partially Update an Object in MongoDB: Overlay / Merge with Existing Data
Have you ever encountered a situation where you need to update only certain fields of an object in MongoDB without losing the existing data? Look no further! In this article, we'll tackle this common issue head-on and provide you with easy solutions. So grab a cup of ☕ and let's dive in!
The Challenge: Updating Specific Fields Without Overwriting the Rest
Consider the following scenario: you have a document saved in MongoDB with the following structure:
{
_id: ...,
some_key: {
param1: "val1",
param2: "val2",
param3: "val3"
}
}
Now, let's say you have an object with new information on param2
and param3
from the outside world that you want to save:
var new_info = {
param2: "val2_new",
param3: "val3_new"
};
The challenge lies in updating only the new fields (param2
and param3
) while retaining the existing data (param1
). If you perform a regular update using the $set
operator like this:
db.collection.update({ _id: ... }, { $set: { some_key: new_info } });
MongoDB will replace the entire some_key
object, resulting in the loss of param1
. 🙀
The Solution: Merging or Overlaying New Fields
To successfully update only the new fields without overwriting the existing ones, you can use the $set
operator along with the $setOnInsert
operator. Follow these steps:
Create an update object that includes both
$set
and$setOnInsert
operators:var updateObj = { $set: { "some_key.param2": new_info.param2, "some_key.param3": new_info.param3 }, $setOnInsert: { "some_key.param1": "val1" } };
Here, we use the dot notation to specify the individual fields within the object that we want to update or insert.
Perform the update by using the
update()
method:db.collection.update({ _id: ... }, updateObj);
This approach ensures that only the specified fields (param2
and param3
) are updated, while the rest of the fields (like param1
) are retained. 💡
Example in Java
Since you mentioned you're using the Java client, let's provide a concrete example using the Java driver. Here's how you can achieve partial updating with overlay/merge in Java:
MongoCollection<Document> collection = database.getCollection("your_collection");
Document updateObj = new Document("$set",
new Document("some_key.param2", new_info.get("param2"))
.append("some_key.param3", new_info.get("param3")))
.append("$setOnInsert",
new Document("some_key.param1", "val1"));
collection.updateOne(eq("_id", yourId), updateObj);
Ensure that you have imported the necessary classes from the com.mongodb.client
package.
Conclusion
Updating specific fields in MongoDB without losing the rest of the document is made possible by utilizing the $set
and $setOnInsert
operators. By following the steps outlined in this article, you can easily overlay or merge new fields with the existing data.
Next time you find yourself in a similar situation, remember this handy guide and save yourself from the headache of manual field identification. 😎
Have you encountered any other MongoDB dilemmas? Let us know in the comments below! And don't forget to share this article with your fellow MongoDB enthusiasts. Happy coding! 👩💻👨💻
*[MongoDB]: A NoSQL database management system *[Java]: A popular programming language for building enterprise applications *[API]: Application Programming Interface