Get names of all keys in the collection
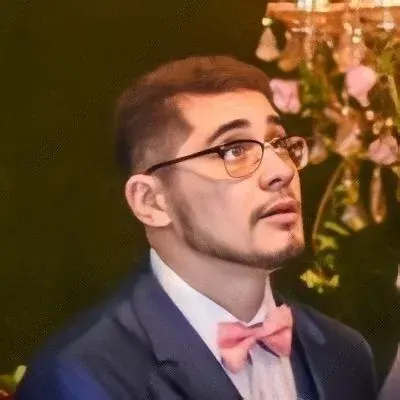
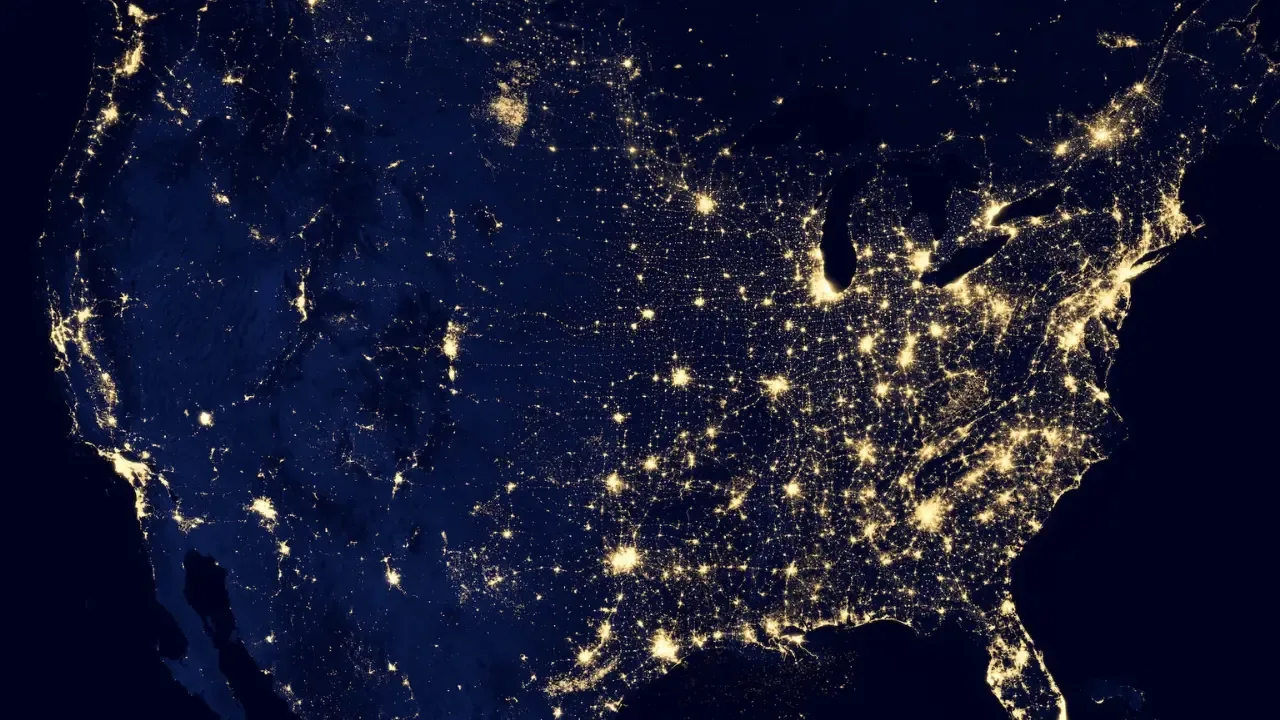
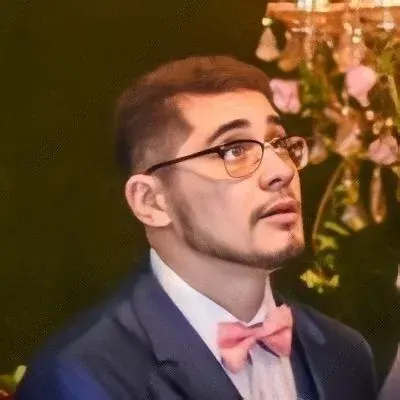
π Unleashing the Power of MongoDB Keys π
Oh, keys, those little hidden gems in a MongoDB collection ποΈ We have all been there, desperately trying to find a way to retrieve the names of all the keys in a collection. Whether you're a beginner or an experienced developer, this can be quite the head-scratcher. But fear not! π¦ΈββοΈ I'm here to guide you through this quest with easy solutions that will have you unleashing the power of MongoDB keys in no time. Let's dive in! π»
The Quest Begins
Imagine you have a MongoDB collection filled with documents like this:
db.things.insert( { type : ['dog', 'cat'] } );
db.things.insert( { egg : ['cat'] } );
db.things.insert( { type : [] } );
db.things.insert( { hello : [] } );
And your goal is to retrieve the unique keys within this collection:
type, egg, hello
Solution 1: Aggregation Magic β¨
One of the most powerful tools at your disposal is the MongoDB aggregation framework π§ββοΈ It allows you to perform complex operations on your data, including extracting keys from documents. Let me show you how:
db.things.aggregate([
{ $project: { keys: { $objectToArray: "$$ROOT" } } },
{ $unwind: "$keys" },
{ $group: { _id: null, keys: { $addToSet: "$keys.k" } } },
{ $project: { _id: 0 } }
])
What just happened here? Let me break it down for you:
We use the
$project
stage to convert each document into an array of key-value pairs using$objectToArray
.The
$unwind
stage flattens the resulting array, ensuring each key-value pair is treated as a separate document.With the
$group
stage, we group all the documents together, extract the keys using$addToSet
, and voilΓ ! π We have our unique keys.Finally, the
$project
stage removes the_id
field for cleaner results.
Solution 2: Code Mastery π»
If you prefer a more hands-on approach, fear not, for there is another way! You can accomplish the same result using a few lines of JavaScript code:
const keys = new Set();
db.things.find().forEach(doc => {
Object.keys(doc).forEach(key => keys.add(key));
});
keys
π Let's examine this in detail:
We create a
Set
object calledkeys
to store our unique keys. ASet
guarantees uniqueness, just what we need!The
forEach
method is used to iterate over each document in the collection.For each document, we extract its keys using
Object.keys()
and add them to ourkeys
set.Finally, we get our unique keys by accessing the
keys
set.
It's Your Turn! π
Now that you have learned two powerful ways to retrieve the names of all keys in a MongoDB collection, it's time to apply your newfound knowledge πͺ Give it a try and see how it can benefit your project!
Remember, MongoDB keys are like a treasure map πΊοΈ, leading you to valuable insights about your data. Dive in, experiment, and let the keys unlock a whole new world of possibilities!
If you have any questions or suggestions, don't hesitate to leave a comment below π I'm here to help you navigate the MongoDB universe!
Happy coding! π»β¨