Find document with array that contains a specific value
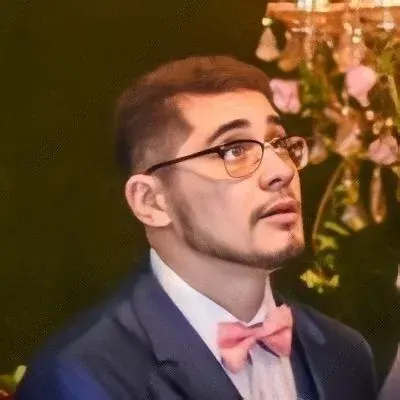
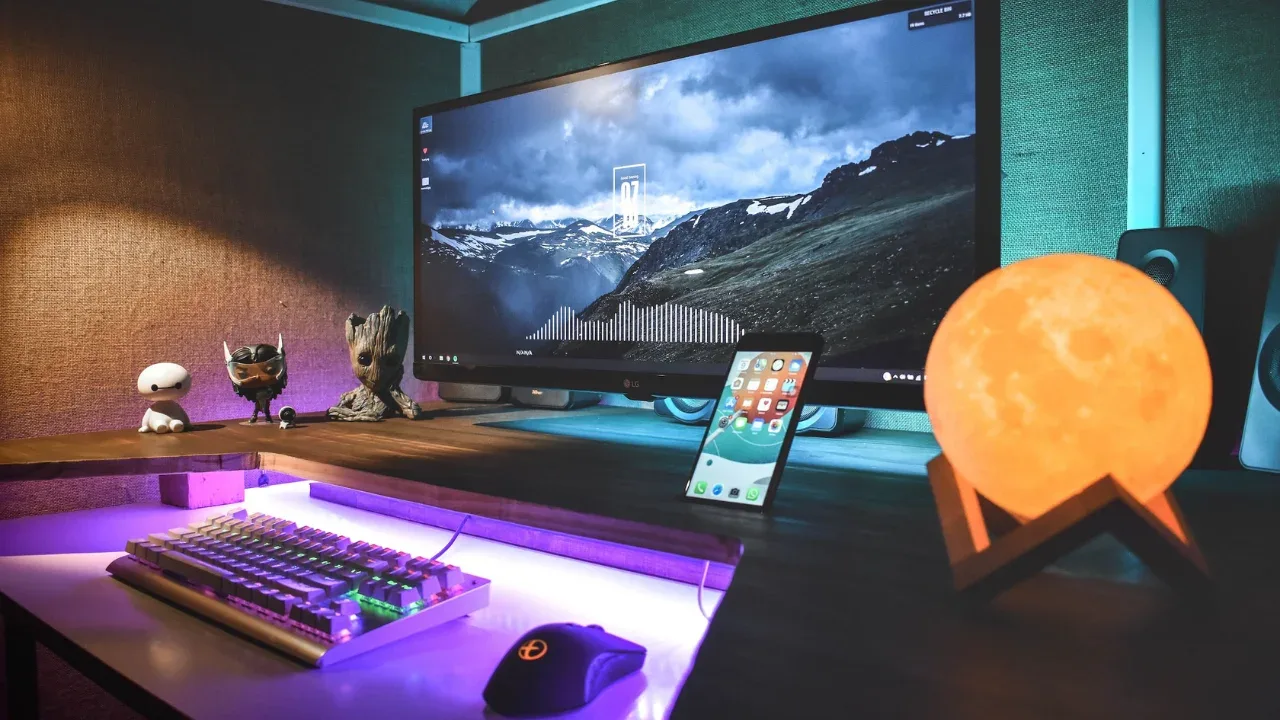
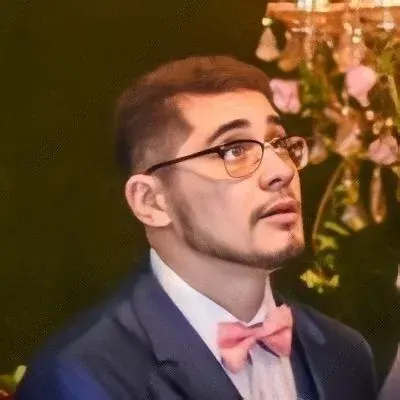
How to Find Documents with an Array that Contains a Specific Value π£
Are you struggling to find documents in your MongoDB database that have an array containing a specific value? Look no further! In this blog post, we'll explore a common issue faced by many developers and provide you with easy solutions to tackle this problem using Mongoose.
The Scenario π½οΈ
Let's say you have a MongoDB schema for a person
collection with the following structure:
person = {
name: String,
favoriteFoods: Array
}
The favoriteFoods
field is an array populated with strings representing the person's favorite foods.
Now, you want to find all the persons who have "sushi" as their favorite food. How can you accomplish this efficiently using Mongoose?
The Expected Solution π‘
At first, you might expect to use the $contains
operator in MongoDB. However, there's a catch β this operator doesn't exist! So, we need to find an alternative approach to solve this problem.
Solution: Using $in Operator π―
Fortunately, MongoDB provides the $in
operator that allows us to determine if an array contains any of the specified values. In our case, we can use it to find documents that have "sushi" in their favoriteFoods
array.
Here's how you can do it using Mongoose:
PersonModel.find({ favoriteFoods: { $in: ["sushi"] } }, function(error, persons) {
if (error) {
console.error(error);
} else {
console.log(persons);
}
});
By passing { favoriteFoods: { $in: ["sushi"] } }
as the query object to find()
, Mongoose will search for documents where the favoriteFoods
array contains "sushi". The resulting documents will then be returned, allowing you to work with the data as needed.
Conclusion π
Finding documents in MongoDB with an array that contains a specific value doesn't have to be a headache. By using the $in
operator in Mongoose, you can easily filter and retrieve the desired documents without any fuss.
Whether you're building a restaurant review app or simply exploring food preferences, this solution will come in handy. So go ahead, try it out, and elevate your MongoDB querying game!
Got any other MongoDB questions or want to share your success stories? Feel free to leave a comment below or reach out on social media. We'd love to hear from you! π
Keep coding and bon appΓ©tit! ππ½οΈ