Delete a key from a MongoDB document using Mongoose
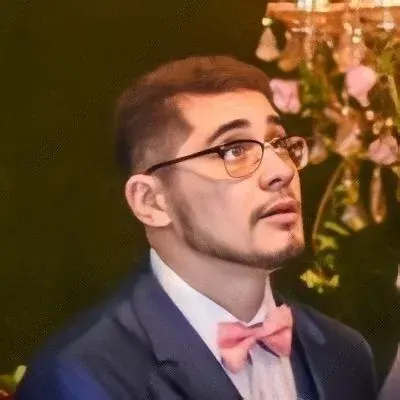
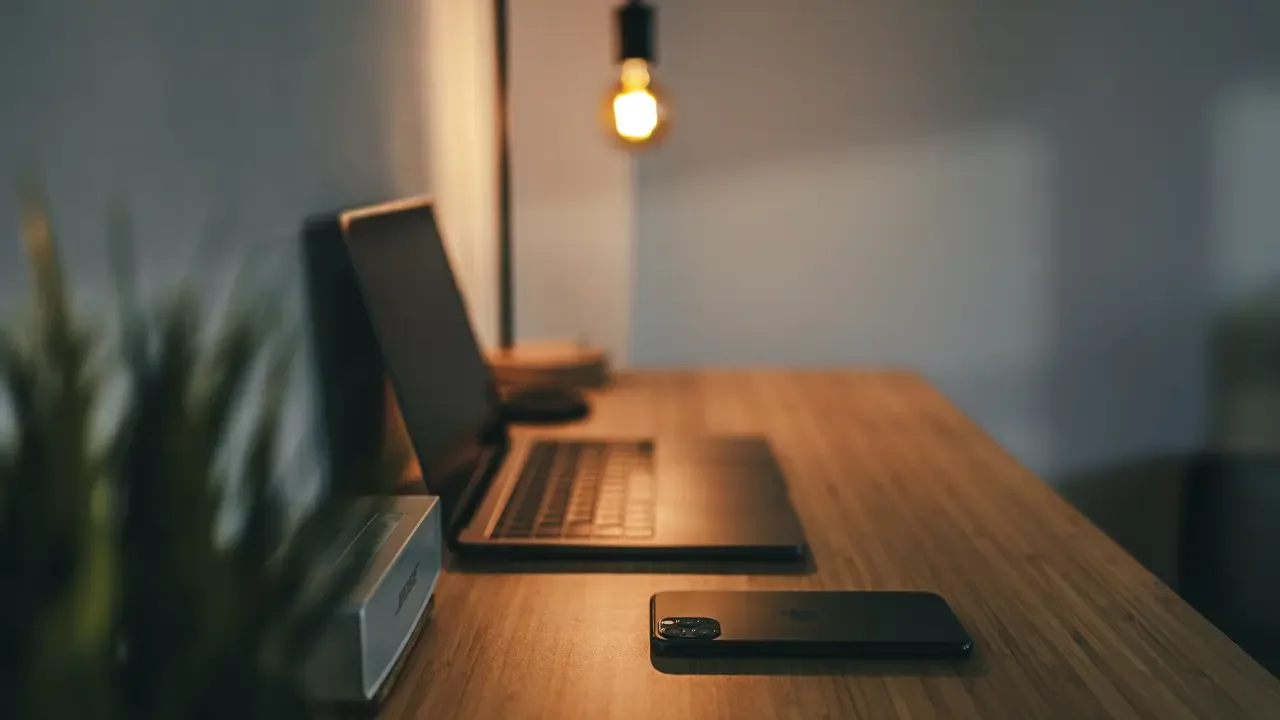
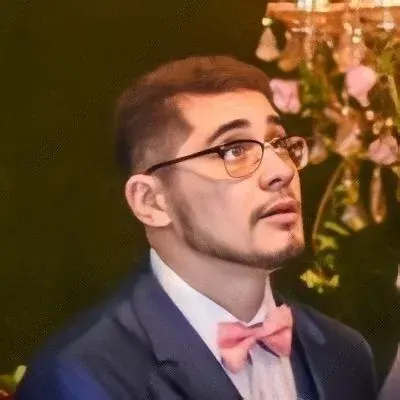
Deleting a Key from a MongoDB Document using Mongoose
Are you struggling to remove a key from a document in MongoDB using Mongoose? You're not alone! This common issue often puzzles developers, but fear not, we've got you covered. In this guide, we will explore easy solutions to completely remove a key from a MongoDB document using Mongoose.
The Problem
Let's take a look at the code snippet you provided:
User.findOne({}, function(err, user) {
// Correctly sets the key to null... but it's still present in the document
user.key_to_delete = null;
// Doesn't seem to have any effect
delete user.key_to_delete;
user.save();
});
In the above code, you attempted to set the key_to_delete
to null
and use the delete
operator to remove it from the document. However, you noticed that even though the value was set to null
, the key itself was still present in the document.
This happens because Mongoose doesn't automatically remove keys when the value is set to null
or when the delete
operator is used.
Solution 1: Using unset
Fortunately, Mongoose provides a handy method called unset
that allows us to remove a key from a document:
User.findOne({}, function(err, user) {
user.unset('key_to_delete');
user.save();
});
By using the unset
method, we directly tell Mongoose to remove the specified key. After calling user.save()
, the document will no longer contain the key_to_delete
.
Solution 2: Using deleteOne
Method
Another approach to remove a key from a MongoDB document is to use the deleteOne
method provided by Mongoose.
User.updateOne({}, { $unset: { key_to_delete: 1 } }, function(err) {
// Handle error and provide appropriate feedback
});
In this solution, we use the updateOne
method and the $unset
operator to remove the key_to_delete
from the document. Make sure to handle any errors that may occur during the operation.
Conclusion
Removing a key from a MongoDB document using Mongoose may initially seem tricky, but with the right knowledge, it becomes a breeze. Hopefully, this guide helped you understand the common issues and provided you with easy and effective solutions.
Now it's your turn! Give these solutions a try in your project and let us know how it goes. If you have any further questions or tips to share, feel free to leave a comment below. Happy coding! 💻🚀
Note: Don't forget to link back to your blog or encourage readers to leave comments, share the post, or follow your social media accounts. This call-to-action will help drive reader engagement and broaden your blog's reach.