Sort a list of objects in Flutter (Dart) by property value
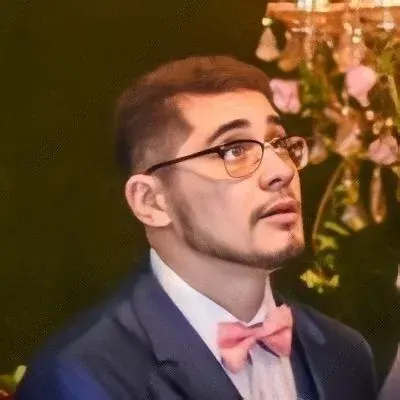
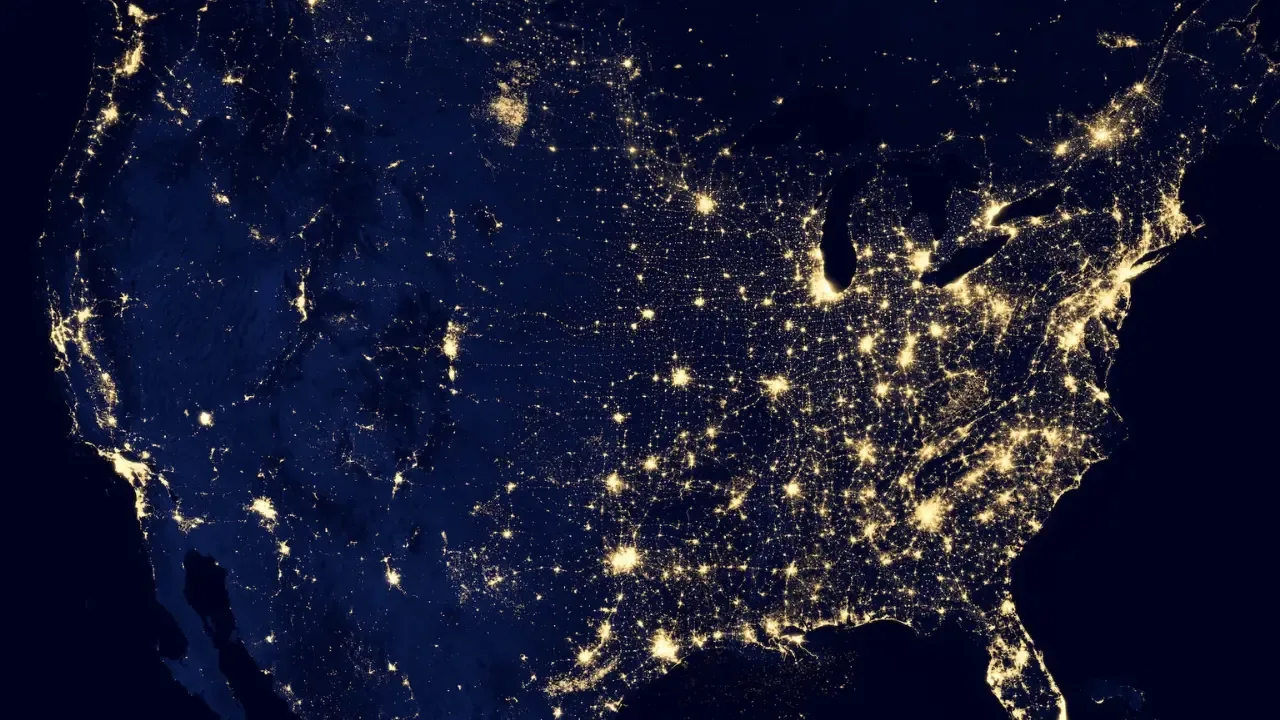
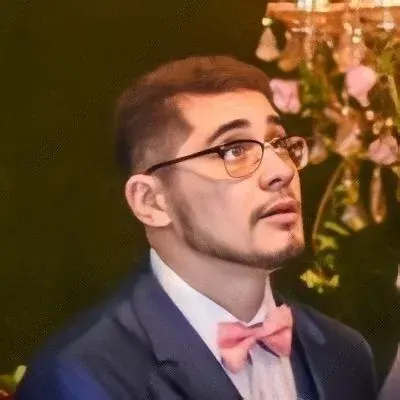
How to Sort a List of Objects in Flutter (Dart) by Property Value ๐๐ข
Sorting a list of objects is a common task in any programming language, including Flutter with Dart. However, sorting by a specific property value can be a bit tricky. ๐ค
In this guide, we'll explore a problem that many developers face: how to sort a list of objects by the alphabetical order of one of its properties. ๐
The Problem and Common Issues ๐งโ
Imagine you have a list of objects, and each object has multiple properties. You want to sort this list based on one particular property, not its object name but the actual value the property holds. This can be challenging, especially if you're new to Flutter and Dart.
The common issues that arise when trying to solve this problem include:
Lack of understanding: Understanding how sorting works in Dart and Flutter can be confusing.
Undefined ordering logic: Deciding how to order the objects can be tricky, especially when working with different data types. Should it be case-insensitive? Should it ignore special characters?
Efficiency concerns: Finding an efficient sorting algorithm, especially for large lists, is crucial to ensure good performance in your app.
Easy Solutions and Examples ๐ก๐งฉ
Let's dive into some easy solutions to sort a list of objects in Flutter (Dart) by a specific property value:
Solution 1: Using the sort
method
The simplest approach is to use Dart's built-in sort
method. This method takes a comparator function as an argument, allowing you to define the sorting logic. Here's an example:
myObjects.sort((a, b) => a.property.compareTo(b.property));
This code sorts the myObjects
list in ascending order based on the property
value. To sort in descending order, swap a
and b
in the comparator function.
Solution 2: Implementing the Comparable
interface
If you want more control over the sorting logic and a cleaner syntax, you can make your objects implement the Comparable
interface. This approach is particularly useful when you have complex sorting requirements.
Here's an example:
class MyObject implements Comparable<MyObject> {
final String property;
MyObject(this.property);
@override
int compareTo(MyObject other) {
return property.compareTo(other.property);
}
}
// Usage:
List<MyObject> myObjects = [
MyObject('Banana'),
MyObject('Apple'),
MyObject('Orange'),
];
myObjects.sort();
In this example, the MyObject
class implements the compareTo
method from the Comparable
interface. The sort
method then uses this method internally to sort the list.
Wrap Up and Engage! ๐๐
You've learned how to sort a list of objects in Flutter (Dart) by property value using easy and effective solutions. ๐๐
Next time you face the challenge of sorting a list of objects alphabetically based on one of their properties, you can confidently implement these approaches.
Do you have other Flutter or Dart-related questions? Let me know in the comments below! ๐๐