How do I prompt for Yes/No/Cancel input in a Linux shell script?
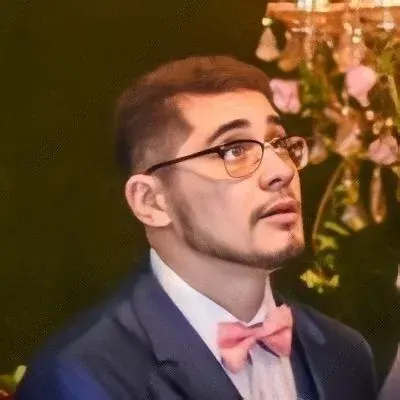
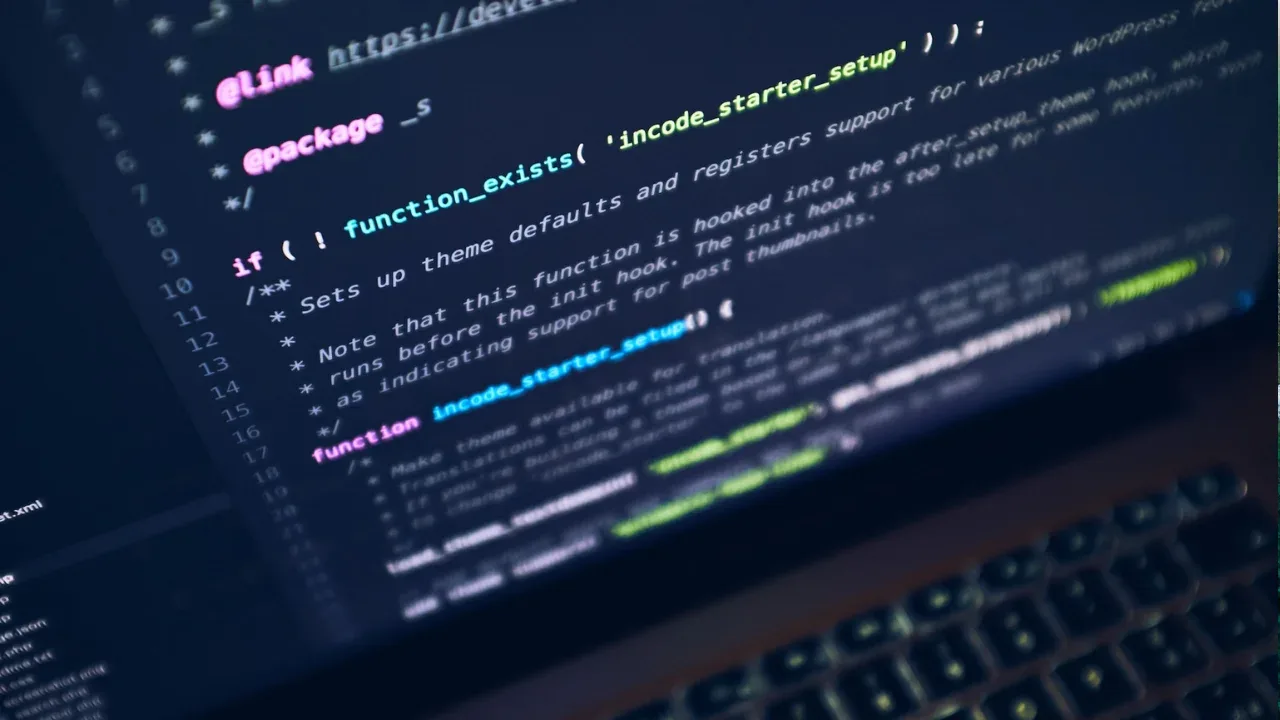
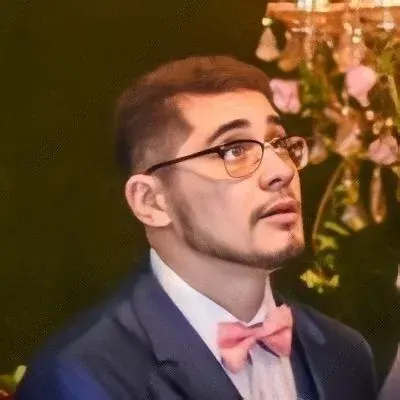
How to Prompt for Yes/No/Cancel Input in a Linux Shell Script 🤔💻
Have you ever found yourself needing to ask the user for a simple yes, no, or cancel input in a Linux shell script? It's a common situation when you want to create an interactive script or automate certain tasks. In this blog post, we'll explore different methods to achieve this goal and provide you with easy solutions. Let's dive in! 🚀
The Challenge: Pausing Input and Prompting for Choices
To start, let's understand the challenge at hand. You want to pause the execution of your shell script momentarily and prompt the user with a standard "yes," "no," or "cancel" type question. The goal is to obtain the user's input and act accordingly in your script.
Solution #1: Using the read
Command
The simplest way to achieve this is by using the read
command. Here's how you can prompt the user and obtain their input in a Linux shell script:
echo "Do you want to proceed? (Y/N/C)"
read choice
case $choice in
y|Y)
echo "User chose 'Yes'";;
n|N)
echo "User chose 'No'";;
c|C)
echo "User chose 'Cancel'";;
*)
echo "Invalid choice";;
esac
Let's break it down:
The
echo
command is used to display the question to the user.The
read
command waits for the user to input their choice and stores it in thechoice
variable.The
case
statement checks the value ofchoice
and executes the corresponding code block based on the user's input.
This method allows you to handle multiple user choices and take different actions accordingly.
Solution #2: Utilizing the select
Command
Another option that provides a more interactive experience is to use the select
command. It creates a simple menu system for the user to choose from. Here's an example:
echo "Select your choice:"
select choice in "Yes" "No" "Cancel" "Quit"; do
case $choice in
Yes)
echo "User chose 'Yes'"
break;;
No)
echo "User chose 'No'"
break;;
Cancel)
echo "User chose 'Cancel'"
break;;
Quit)
echo "Quitting..."
exit;;
*)
echo "Invalid choice";;
esac
done
Here's what's happening:
The
echo
command displays the prompt to the user.The
select
statement creates a menu based on the options provided.The
case
statement determines the action to execute based on the user's choice.The
break
statement exits the loop after the user makes their selection, ensuring the script doesn't keep asking for input.
Feel free to extend the menu options or modify the code to fit your specific needs.
Engage with the Community! 🤝
We've covered two simple solutions to prompt for Yes/No/Cancel input in a Linux shell script. Now it's your turn! 🎉
Have you encountered this challenge before? How did you solve it? Do you have any alternative methods? Let us know by leaving a comment below. Sharing your experiences and insights helps others in the community. Together, we can create more efficient and user-friendly shell scripts! 💪💡
Happy scripting! 💻✨