Extract file basename without path and extension in bash
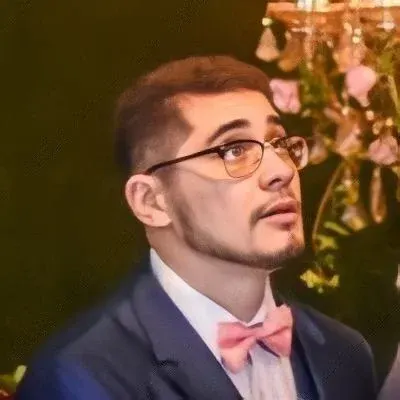

Extracting File Basename without Path and Extension in Bash
So you have some file names with paths and extensions, and you just want to extract the base name of the file without the path and extension, right? Well, you've come to the right place! In this blog post, we'll discuss common issues and provide easy solutions to help you achieve this task in Bash.
The Problem
Let's start by understanding the problem at hand. You have file names like these:
/the/path/foo.txt
bar.txt
And you want to extract:
foo
bar
While the script you provided might seem like the right approach, it unfortunately doesn't work as expected. Here's the script you mentioned:
#!/bin/bash
fullfile=$1
fname=$(basename $fullfile)
fbname=${fname%.*}
echo $fbname
The script uses the basename
command to strip away the path from the file name stored in the fullfile
variable. It then uses parameter expansion with %.*
to remove the extension from the file name and stores the result in the fbname
variable. Lastly, it echoes the fbname
.
What's Wrong with the Script?
The problem lies in how basename
and parameter expansion are being used. When the file name doesn't have a path, basename
won't remove anything, resulting in the original file name being stored in fname
. Consequently, the parameter expansion %.*
won't remove anything either, giving us a file name with the extension intact.
The Right Way to Do It
To rectify the issue, we need to make a small adjustment to the script. Here's the revised version:
#!/bin/bash
fullfile=$1
fname=$(basename "$fullfile")
fbname=$(echo "$fname" | cut -d. -f1)
echo "$fbname"
Instead of relying solely on basename
and parameter expansion, we introduce the cut
command. cut
is a powerful tool in Bash that can extract specific parts of a line based on a delimiter. In our case, we use .
as the delimiter and extract the first field (-f1
) of the file name.
By enclosing the variables within double quotes, we ensure that the script works correctly even if the file name contains spaces or special characters.
Wrapping Up
Extracting the base name of a file without the path and extension may seem like a trivial task at first, but it can be tricky in Bash. By understanding the shortcomings of the initial approach and adjusting our script accordingly, we can successfully accomplish this task.
Now that you have a working solution, go ahead and try it out! If you encounter any issues or have any further questions, feel free to leave a comment below. Sharing your experiences and feedback will not only help us learn from each other, but also engage and assist fellow readers.
Happy scripting! 💻🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
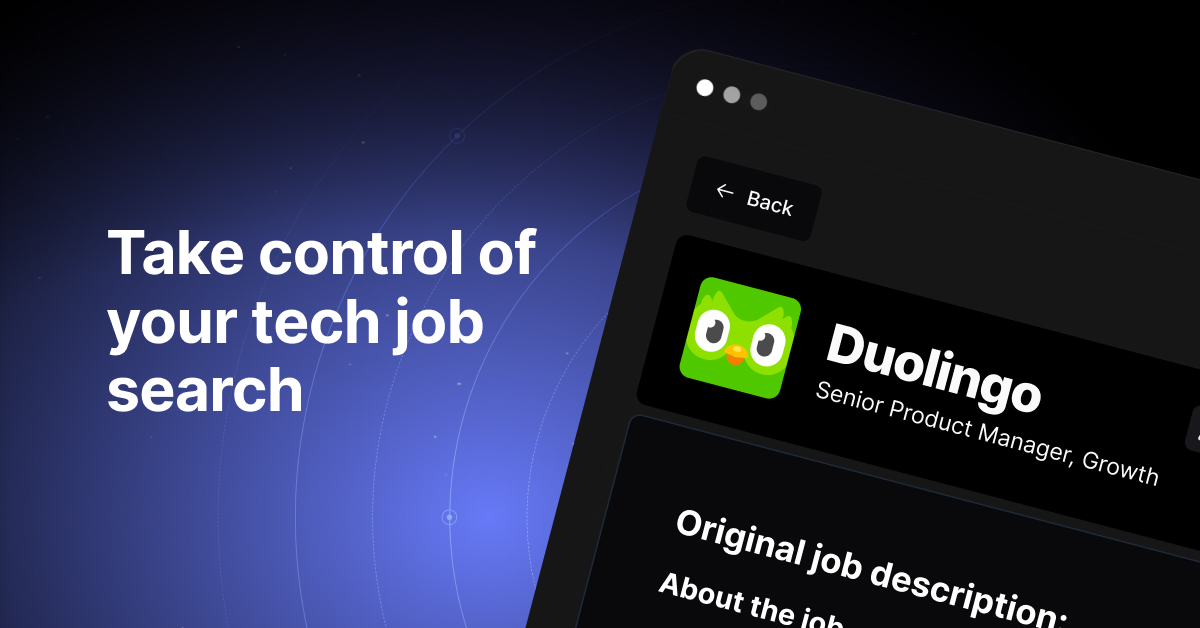