Laravel save / update many to many relationship
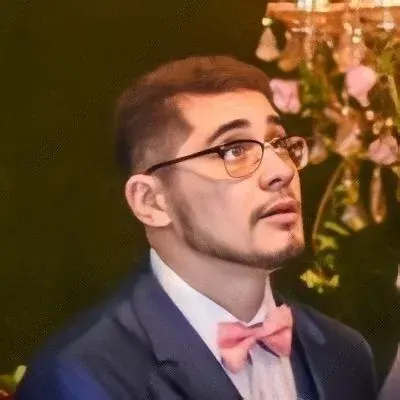
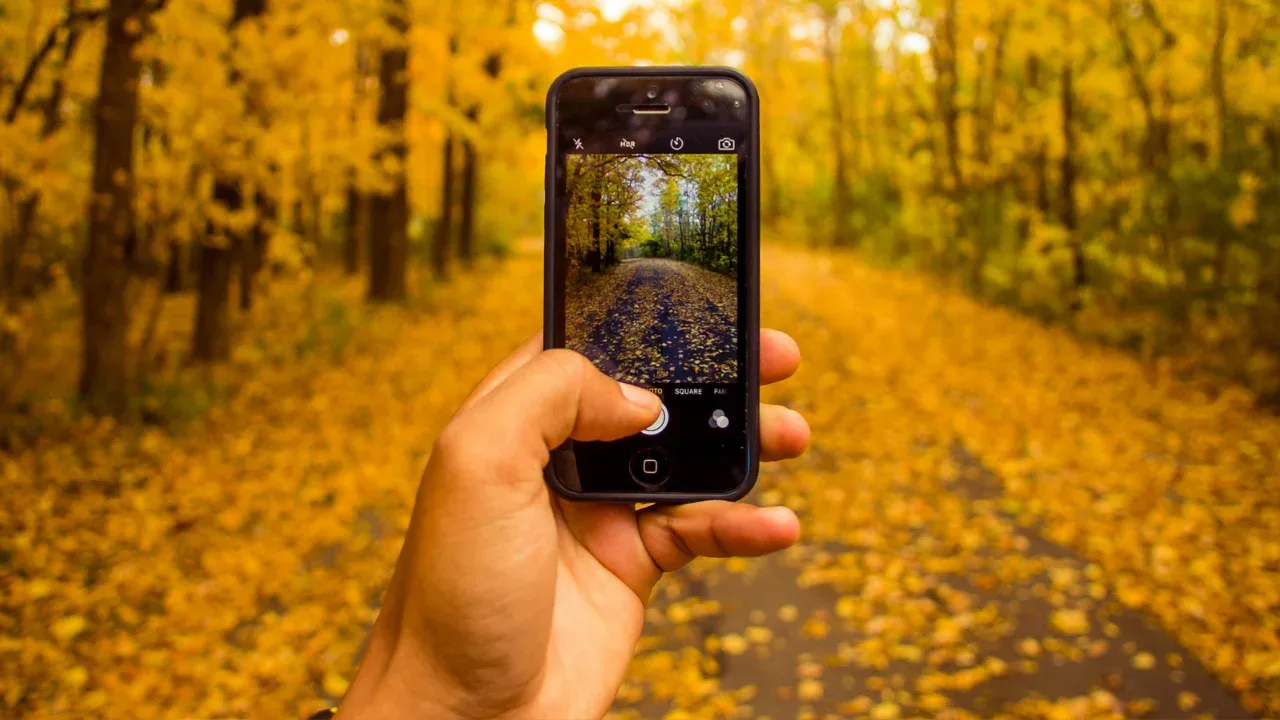
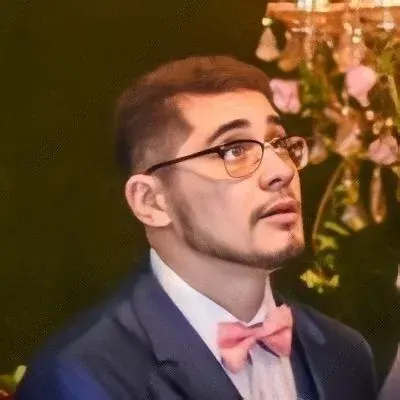
Laravel Save/Update Many to Many Relationship: A Complete Guide
Introduction
Are you struggling with saving and updating many-to-many relationships in Laravel? You're not alone! This can be a tricky task, especially when you need to handle the addition and removal of related entities dynamically. In this guide, we will walk you through a step-by-step process to help you solve this problem effortlessly. Let's dive in!
The Challenge
The specific challenge mentioned by a user is that they have a "tasks" table and a "users" table, with a many-to-many relationship between them. The user wants to be able to assign multiple users to a specific task using an HTML multiple select input in an update form. The user also wants to be able to dynamically add or remove users through the same form.
Step 1: Define the Relationship
To start, let's define the relationship between the "User" and "Task" models. In the "User" model, add the following code:
public function tasks()
{
return $this->belongsToMany(Task::class, 'user_tasks');
}
In the "Task" model, add the following code:
public function taskParticipants()
{
return $this->belongsToMany(User::class, 'user_tasks');
}
Step 2: Update the Controller
Next, let's update the "TaskController" to handle the saving and updating of the many-to-many relationship. In the "update" method of the controller, add the following code:
public function update($task_id)
{
if (request()->has('taskParticipants'))
{
foreach(request()->get('taskParticipants') as $worker)
{
$task2 = $task->taskParticipants->toArray();
$task2 = array_add($task2, $task_id, $worker);
$task->taskParticipants()->sync([$task2]);
}
}
}
Step 3: Table Structure
Make sure your table structure matches the model and controller code. Here's an example of the table structure:
tasks
id | title | deadline
user_tasks
id | task_id | user_id
Conclusion
You've made it! Now you have a clear understanding of how to save and update many-to-many relationships in Laravel. By following these three simple steps, you can easily handle dynamic addition and removal of related entities in your application. Feel free to experiment and explore different ways to customize this solution based on your specific requirements.
If you found this guide helpful, don't forget to share it with your fellow developers who might be facing the same challenge. Together, we can simplify complex problems and empower the Laravel community!
Have any questions or suggestions? Leave a comment below and let's start a conversation. Happy coding! 👨💻💪