Laravel - Route::resource vs Route::controller
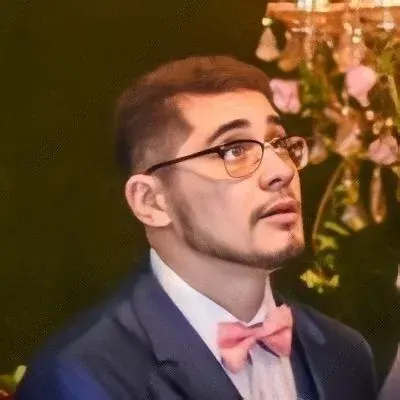
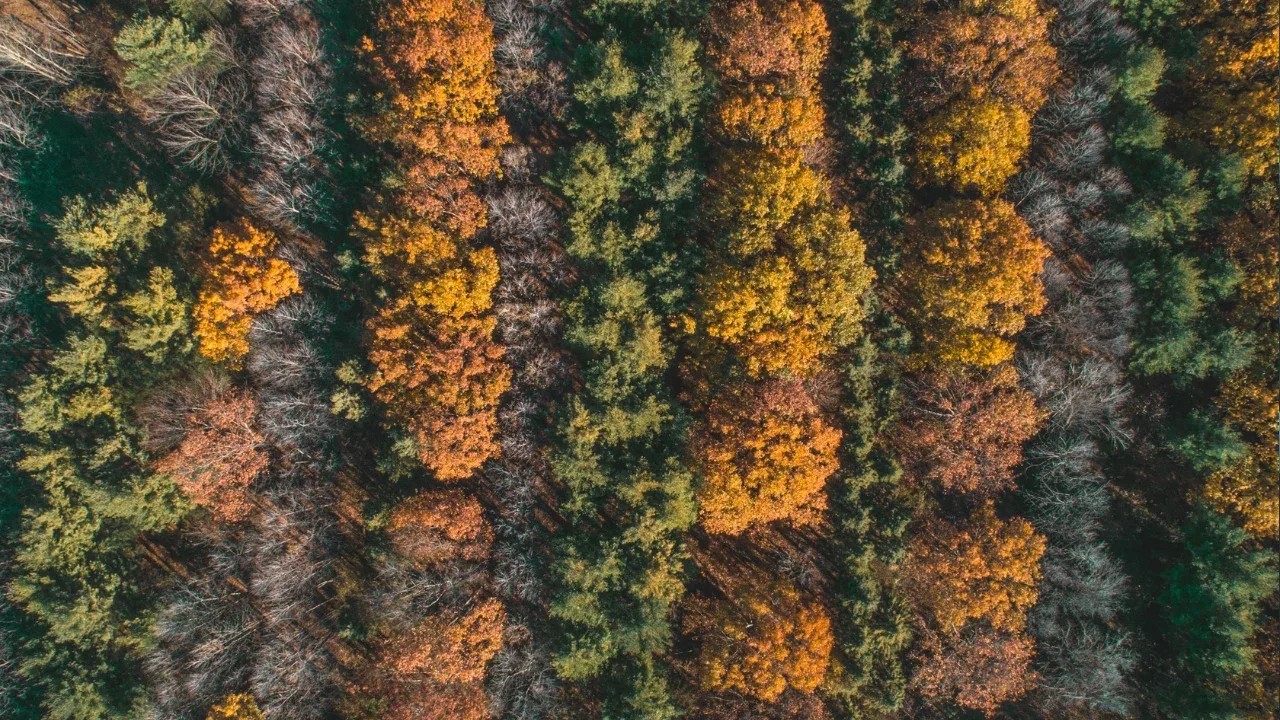
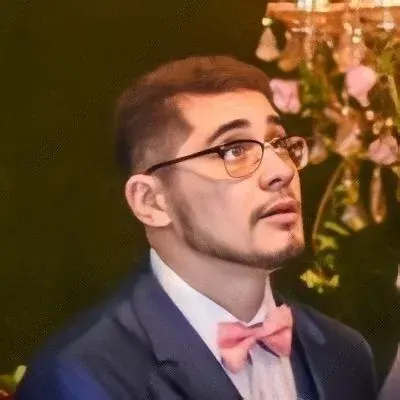
Laravel - Route::resource vs Route::controller: Demystifying the Siblings 👪
So, you stumbled upon the fascinating world of Laravel and now you find yourself scratching your head, trying to understand the difference between Route::resource
and Route::controller
. Don't worry, my tech-savvy friend, I've got your back! 😎
The Mystery Unveiled 🎉
To put it simply, both Route::resource
and Route::controller
are used to define routes in Laravel. However, their purposes and functionalities are slightly different. Let's dive deeper to clear up the confusion.
1. Route::resource: CRUD at its Finest 💥
The Route::resource
method is like the superhero of Laravel routes when dealing with CRUD (Create, Read, Update, Delete) operations. It automatically generates a set of RESTful routes for your specified resource (e.g., posts, comments, users) using standard naming conventions.
To use Route::resource
, you'll specify the resource's URI segment and the corresponding controller:
Route::resource('posts', 'PostController');
This single line of code creates routes for all the necessary actions, such as index
, create
, store
, show
, edit
, update
, and destroy
. How convenient is that? 🤩
2. Route::controller: A Customized Powerhouse 💪
Now, let's talk about its sibling, the Route::controller
method. Unlike Route::resource
, Route::controller
allows you to specify only the needed actions in a more customized manner. It binds a URI segment to a specific controller, without the need to create separate routes for each action.
To unleash the power of Route::controller
, you'll define the URI segment and the associated controller, followed by the methods you want to include:
Route::controller('posts', 'PostController');
Here, you have full control over which methods are available within the specified controller. This flexibility comes in handy when you don't want all the predefined CRUD actions but only a subset of them. 💥
Choosing the Right Path 🛤️
Now that we understand the differences, you might be wondering which approach is considered a good practice. Well, the answer, as often is in tech, is... it depends! 🤷♂️
Use
Route::resource
when you need all (or the majority of) the CRUD actions for your resource. It provides a standardized and consistent way of defining routes, making your codebase more maintainable.Opt for
Route::controller
when you want to have more control and specify only specific actions for your routes. This approach suits scenarios where you have unique requirements and need fine-grained control over your application.
Remember, there is no one-size-fits-all answer, and it ultimately depends on your specific use case and preference.
Easy Solutions Ahead! 🚀
Now that we've shed some light on this topic, let's simplify it even further with some quick solutions to common issues you might encounter when working with these routes.
Issue: "I want to customize the URI segment for my resource routes."
Solution: You can specify a different URI segment by passing an array as the second argument to Route::resource
:
Route::resource('articles', 'ArticleController', ['parameters' => [
'articles' => 'blog_posts',
]]);
Issue: "I only need a subset of CRUD actions. How can I achieve that?"
Solution: With Route::controller
, you can specify only the methods you need by using the only
or except
methods. For example:
Route::controller('posts', 'PostController', [
'only' => ['index', 'show']
]);
// OR
Route::controller('posts', 'PostController', [
'except' => ['create', 'store', 'update', 'destroy']
]);
📣 Take Action and Share Your Thoughts! 🗣️
Now that the fog has lifted, it's your turn to take action! Choose the right route method for your project and experiment with its incredible capabilities.
Don't forget to leave a comment below, sharing your experience with Route::resource
and Route::controller
. Your insights can help fellow developers make informed decisions and spark engaging discussions! Let the Laravel journey begin! 🚀
Keep coding and stay awesome! ✌️