Laravel Redirect Back with() Message
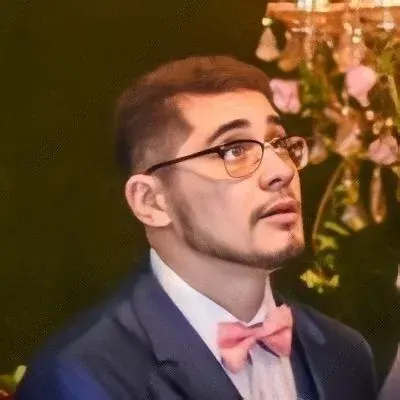
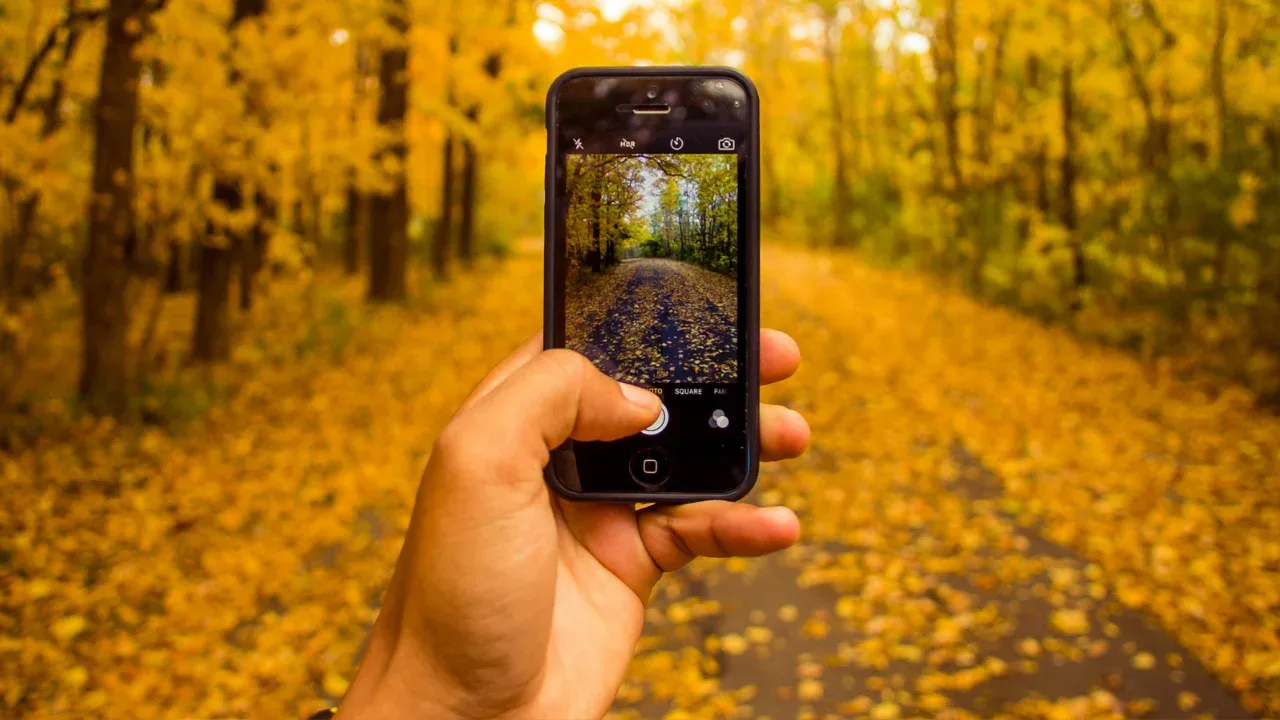
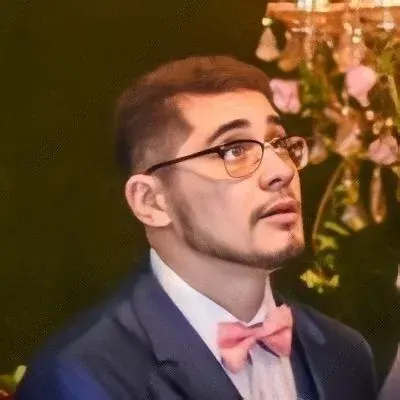
Laravel Redirect Back with() Message: A Simple Guide 😎
So you're trying to redirect to the previous page with a message in Laravel? Great choice! Laravel offers a convenient way to do this using the Redirect::back()->with()
method. However, it seems like you're facing some issues with rendering the message. Don't worry, I've got your back! 🤝
The Problem 💥
Based on the code snippet you shared, it seems like you're using the App::fatal()
function to handle fatal errors and redirect back to the previous page. Here's the code for reference:
App::fatal(function($exception)
{
return Redirect::back()->with('msg', 'The Message');
});
In your view, you're trying to retrieve the message using Sessions::get('msg')
. However, you mentioned that nothing is getting rendered. Let's dig into the solution and see if we can get things back on track. 💪
The Solution 🚀
The issue here lies in the way you're trying to access the message in your view. Instead of using Sessions::get('msg')
, you should be using the session()
helper function to retrieve the flashed message. Here's the corrected code:
{{ session('msg') }}
This will retrieve the 'msg'
value from the session flash data and render it in your view.
If you're still facing issues, make sure that you have the web
middleware applied to your routes. This middleware is responsible for handling sessions in Laravel. You can add it in your routes/web.php
file like this:
Route::group(['middleware' => 'web'], function () {
// Your routes here
});
Now, when a fatal error occurs, the user will be redirected back to the previous page, and the message will be rendered successfully. 🎉
Take it a Step Further! 🚀
Now that you've fixed the issue at hand, why not enhance the user experience even more? Instead of using a generic message like 'The Message'
, you can customize the redirect message to provide more context to your users.
For example, if the fatal error was due to an expired session, you could use something like:
return Redirect::back()->with('msg', 'Your session has expired. Please log in again.');
This will provide a more user-friendly message, making it easier for your users to understand what went wrong.
Conclusion 🎉
Redirecting back to the previous page with a message is a powerful feature in Laravel, allowing you to provide seamless feedback to your users. By ensuring that you use the session()
helper function to access the flashed messages, you can easily render the message in your views.
Remember, always customize your redirect messages to provide useful information to your users. And don't forget to apply the web
middleware to your routes!
Now that you've mastered this technique, go ahead and implement it in your own projects. Happy redirecting! 😄🚀
Got any questions or suggestions? Feel free to leave a comment below and let's discuss! 👇