Laravel redirect back to original destination after login
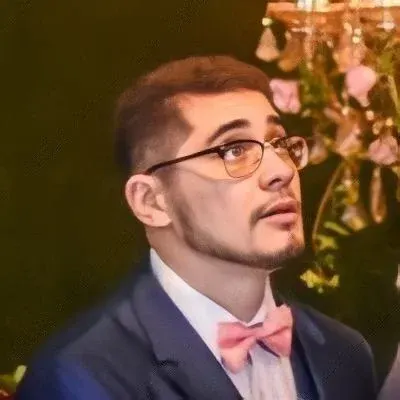
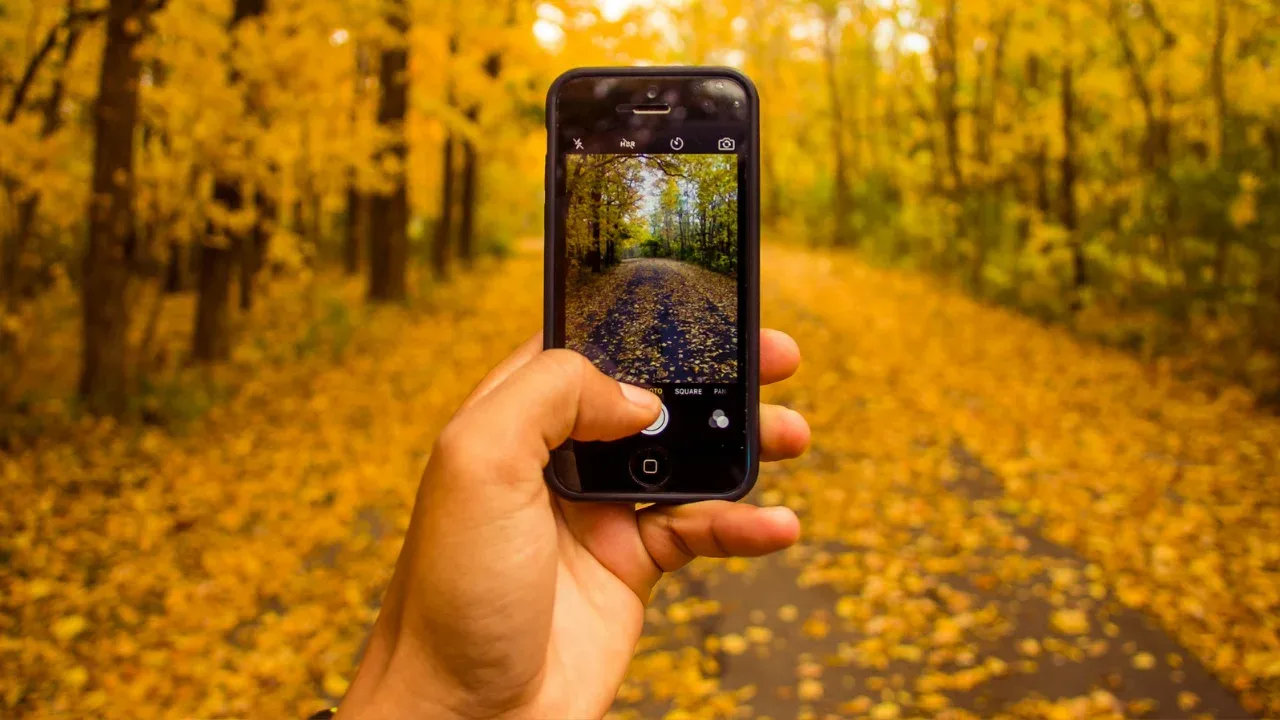
Redirecting Back to the Original Destination with Laravel
š·
So you're building a web application with Laravel and you encounter this common scenario: a user clicks on a link or tries to access a protected page, Laravel's auth
filter kicks in and redirects them to a login page. After successfully logging in, you want to send them back to the original page they were trying to access. But how do you know what that page was? š¤
Laravel does provide a handy solution for this situation, ensuring a smooth and seamless user experience. Let's explore some easy ways to achieve this!
1. Using the redirect()->intended()
method
One of the ways Laravel handles redirection after login is by utilizing the redirect()->intended()
method. This method knows where the user was trying to go before being redirected to the login page.
Here's an example of how you can use it in your login controller:
public function login(Request $request)
{
// Perform your login logic here
// Redirect the user back to their intended destination
return redirect()->intended();
}
This method will automatically redirect the user to their intended destination if it exists. If not, it will redirect them to a default location, specified in your RedirectIfAuthenticated
middleware.
2. Customizing the default redirection location
By default, Laravel will redirect authenticated users to the /home
route. However, you can customize this behavior according to your application's requirements. To do so, you can modify the redirectTo
property in your LoginController
:
protected $redirectTo = '/dashboard';
In this example, authenticated users will be redirected to the /dashboard
route instead of the default /home
route.
3. Manually storing and retrieving the intended destination
If you prefer a more manual approach, you can store and retrieve the intended destination yourself. Laravel provides the session
helper to simplify this process.
Here's how you can store the intended destination before redirecting to the login page:
public function index(Request $request)
{
if (!Auth::check()) {
session()->put('url.intended', $request->url());
}
// Continue with your regular logic
}
After the user successfully logs in, you can retrieve the intended destination like this:
public function login(Request $request)
{
// Perform your login logic here
// Get the intended destination from the session
$intended = session()->pull('url.intended', '/');
// Redirect the user back to the intended destination
return redirect($intended);
}
By using the session()->pull()
method, we not only retrieve the intended destination but also remove it from the session, preventing it from being used on subsequent requests.
In conclusion
Redirecting back to the original destination after login in Laravel doesn't have to be a challenging task. With the provided methods, you can easily achieve a seamless user experience. Whether you choose to use Laravel's built-in redirect()->intended()
method or maintain the intended destination manually, the choice is yours.
Now it's your turn! Have you encountered this situation before? How did you handle it? Share your thoughts and experiences in the comments below and let's learn from each other! š
š Don't forget to subscribe to our newsletter for more helpful Laravel tips and tricks straight to your inbox! āļø
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
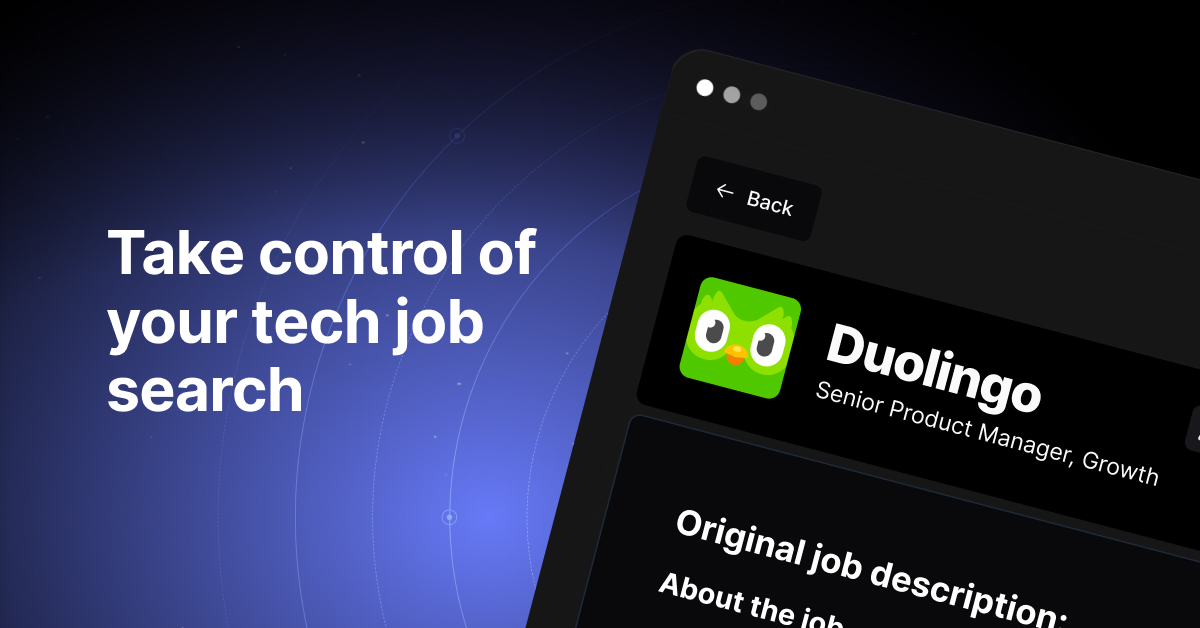