Laravel - Eloquent "Has", "With", "WhereHas" - What do they mean?
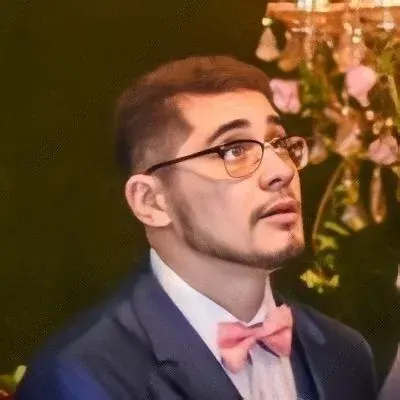
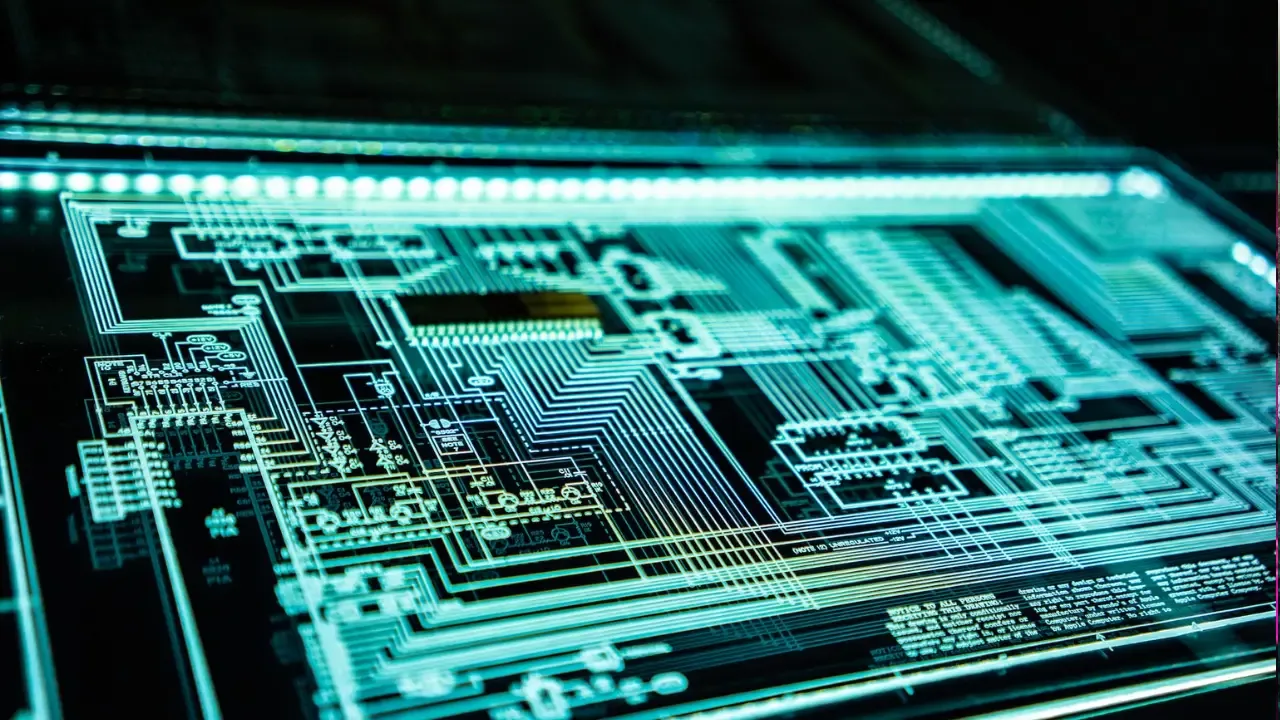
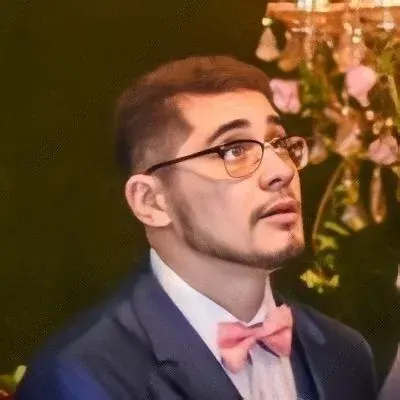
🌟 Understanding Laravel Eloquent "Has", "With", and "WhereHas" 🌟
If you've delved deep into Laravel development, chances are you've come across the powerful Eloquent ORM and its handy relationships feature. Among the several methods provided by Eloquent to define and work with relationships, "has", "with", and "whereHas" are three commonly used methods that can sometimes cause confusion. 🤔
📋 Let's shed some light on the differences between these methods and understand when to use each one of them through a practical example.
🚀 The Scenario: A Blogging Application 🚀
Imagine you're building a blogging application, and you have two models: User
and Post
. Each User
can have multiple Post
instances associated with them. Now let's look at how "has", "with", and "whereHas" can be used to query these relationships.
1️⃣ The "has" Method
The has
method is used to retrieve records that have a specific relationship. In our example, let's say we want to retrieve all the users who have at least one post. We can use the "has" method like this:
$users = User::has('posts')->get();
This will fetch all the users who have at least one post associated with them. 🙌
2️⃣ The "with" Method
The with
method is used to eager load relationships while retrieving records. This means that we can retrieve all the users along with their associated posts. Here's how we can achieve this:
$users = User::with('posts')->get();
Now, each user retrieved will have their associated posts as part of the result. This helps in reducing the number of database queries when accessing the user's posts later in the application. 🎉
3️⃣ The "whereHas" Method
The whereHas
method allows us to put constraints on the relationship. Let's say we want to retrieve all the users who have posts containing the word "Laravel" in their title. We can accomplish this as follows:
$users = User::whereHas('posts', function ($query) {
$query->where('title', 'like', '%Laravel%');
})->get();
Now, we'll get all the users who have at least one post with "Laravel" in the title. This method is useful when we want to filter the parent records based on their relationship's constraints. 😎
📞 Take Action: Share Your Thoughts! 📞
Now that you have a clear understanding of the differences between "has", "with", and "whereHas" in Laravel Eloquent, it's time to put this knowledge into practice and experiment with it in your own projects. Let us know in the comments section below how these methods have helped you in your application development! 💬
If you found this guide helpful, consider sharing it with your fellow developers who might benefit from this valuable information. Happy coding! 🚀✨