Laravel Eloquent - Get one Row
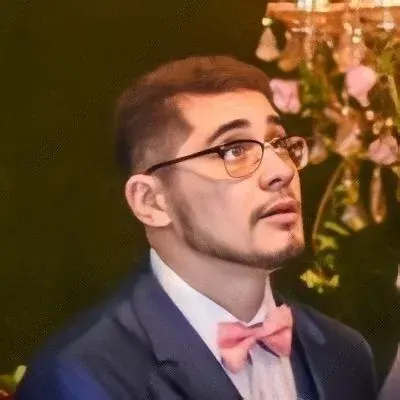
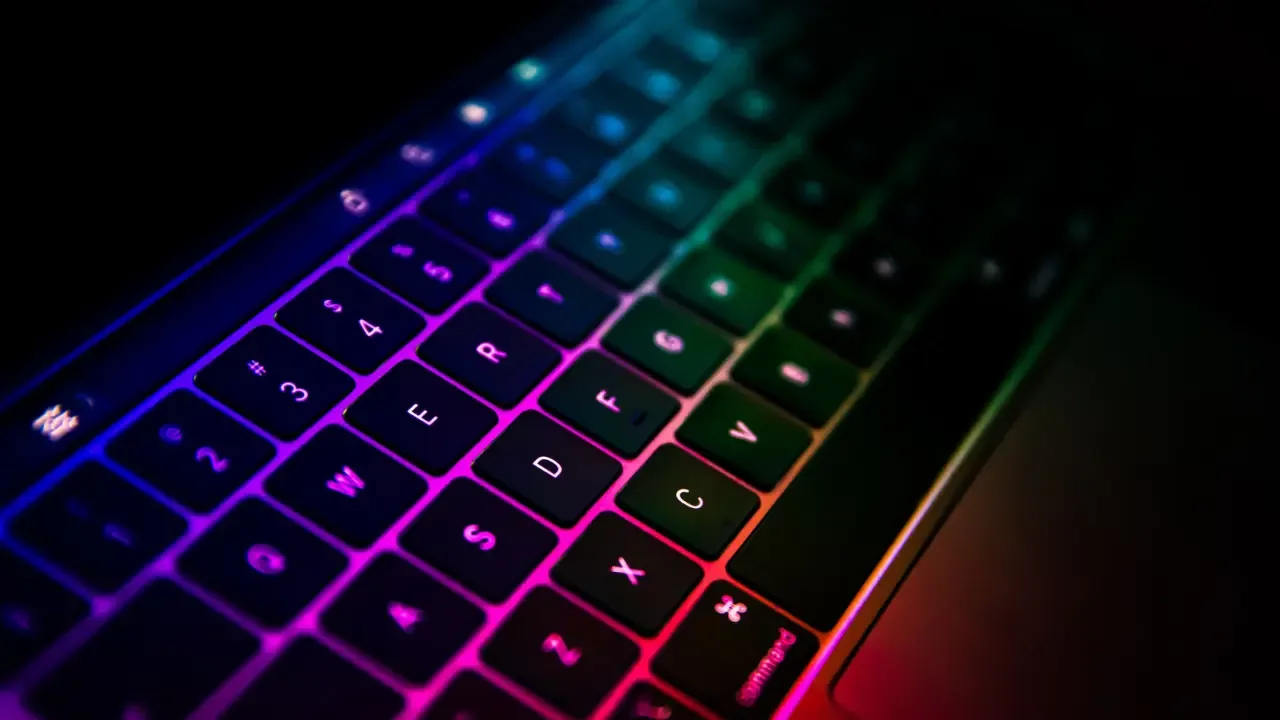
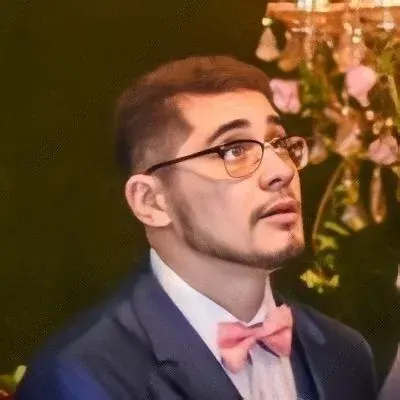
š Title: Laravel Eloquent - Getting that One Special Row!
š Hey there tech enthusiasts! Have you ever found yourself in a dilemma while trying to fetch just one row using Laravel Eloquent? š¤ Don't worry, you're not alone! It's a common issue that many developers face. Today, we're here to help you out and guide you through the process of obtaining that one special row effectively and efficiently. Let's dive in! šŖ
š Understanding the Problem
So, you're trying to fetch a user by their email using User::whereEmail($email)->get()
, but it's returning an array instead of a single row. š« Fret not! The reason for this behavior is that the get()
method returns a collection of results, even if it's just one row. Collections are powerful, but in this case, we need to access a single row directly.
š ļø Finding the Solution
To retrieve just one row, you can make use of the first()
method instead of get()
:
$user = User::where('email', $email)->first();
By using first()
, you'll obtain the desired user as a simple object instead of an array. Now, you can access the user's name directly using $user->first_name
. No more need for $user[0]['first_name']
! š
š Alternative Approaches
While first()
is the most straightforward way to get a single row, there are a couple of additional approaches you can try out.
Using
find()
: If you have a unique identifier for the user, such as their ID, you can directly fetch the user using thefind()
method:
$user = User::find($userId);
Utilizing the
whereEmail()
Method + Chainfirst()
:
In case you prefer to keep the whereEmail()
method, you can chain first()
to it, like so:
$user = User::whereEmail($email)->first();
ā Remember the Caveats
Keep in mind the following points when working with Eloquent queries:
When using
first()
, if no matching record is found,null
will be returned. Ensure you handle this properly in your code to prevent any unexpected errors.If you want to retrieve multiple rows matching your criteria, feel free to stick with
get()
and work with the resulting collection.
š Call-to-Action
Now that you know how to fetch that elusive single row, go ahead and try it out in your own Laravel projects! Share your experience or any other Laravel Eloquent challenges you've faced in the comments below. Let's grow and learn together! šš¬
āļø That's all for today, folks! We hope this guide helped you solve the issue of fetching one row using Laravel Eloquent. Stay tuned for more exciting tech tips, tutorials, and problem-solving discussions. Until next time, happy coding! šš©āš»šØāš»