How to Get the Current URL Inside @if Statement (Blade) in Laravel 4?
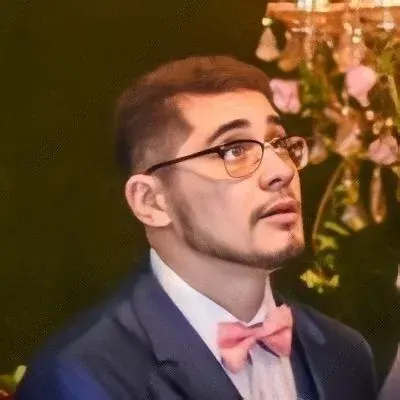
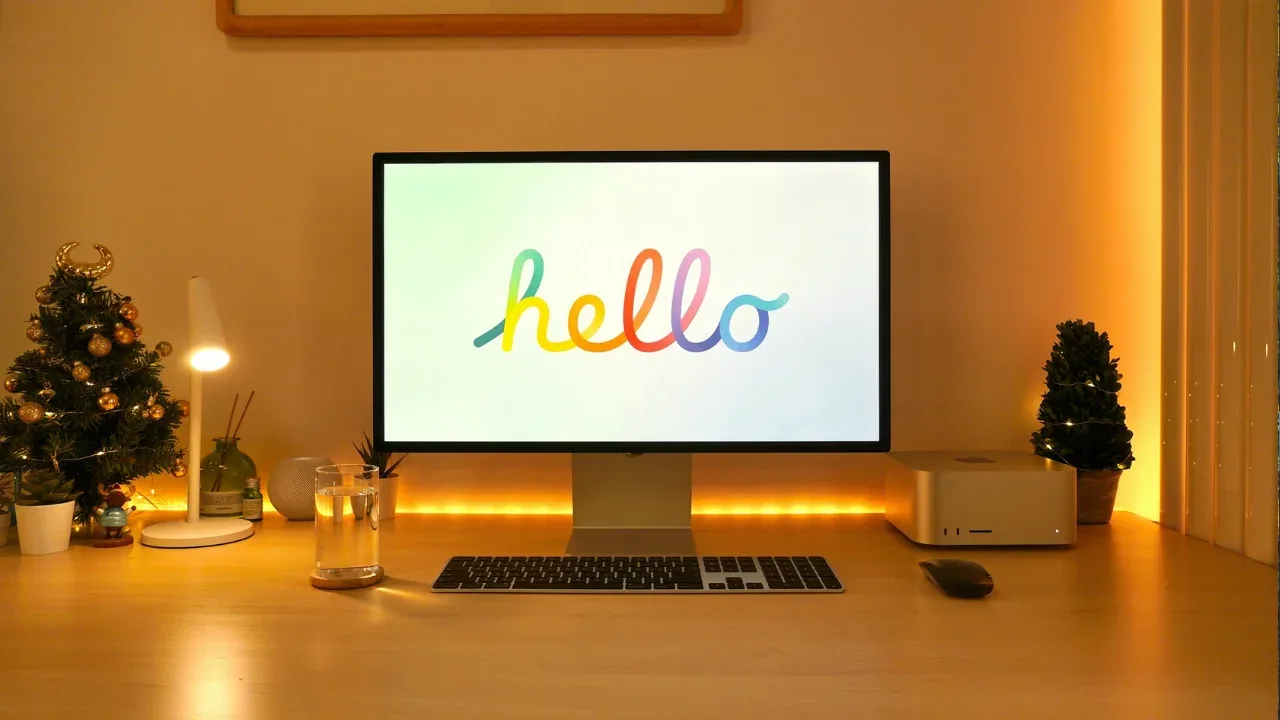
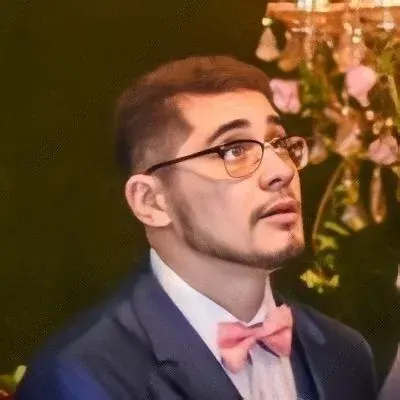
🌟 Supercharge Your Laravel 4 Views: How to Get the Current URL Inside @if Statement (Blade)
So, you're trying to level up your Laravel 4 views and include the current URL inside an @if
statement using Laravel's Blade templating engine? You've come to the right place! I've got your back on this one, buddy! 🙌
The Common Issue
Let's address the problem at hand. You want to access the current URL within an @if
condition in your Laravel 4 view. But guess what? It's not as straightforward as you initially thought. Simply using URL::current()
won't work inside an @if
Blade statement. 😬
The Easy Solution
Don't sweat it! I've got a nifty solution for you that will make accessing the current URL within an @if
statement a piece of 🍰.
To achieve this, you can use the Request
facade provided by Laravel. Here's how you can do it:
First, make sure to import the
Request
facade at the top of your view file:
use Illuminate\Support\Facades\Request;
Now, you can easily access the current URL using the
url()
method provided by theRequest
facade:
{{ Request::url() }}
To use it within an
@if
statement, you can assign the current URL to a variable and then reference the variable in the condition:
@php
$currentUrl = Request::url();
@endphp
@if ($currentUrl === 'your-desired-url')
// Do something awesome here! ✨
@endif
And there you have it! You can now access the current URL inside an @if
statement in your Laravel 4 view. Talk about a win! 🎉
Pro Tip: Using Blade Directives
If you find yourself using this logic frequently, you can level up even more by creating a custom Blade directive. How cool is that? 😎
For example, you can define a directive like @ifCurrentUrl()
in your app/Providers/AppServiceProvider.php
file:
use Illuminate\Support\Facades\Request;
use Illuminate\Support\ServiceProvider;
class AppServiceProvider extends ServiceProvider
{
public function boot()
{
Blade::if('ifCurrentUrl', function ($url) {
return Request::url() === $url;
});
}
}
Now, you can use this custom directive directly in your views:
@ifCurrentUrl('your-desired-url')
// Do something even more awesome here! 🚀
@endifCurrentUrl
Your Turn to Shine! ✨
Now that you've got the solution, it's time for you to put it into action and unlock some serious Laravel 4 magic in your views. Don't forget to customize the URL comparison condition to match your exact needs.
If you have any questions, suggestions, or just want to share your success, feel free to drop a comment below. I'd be thrilled to hear from you! Let's dive deeper into the Laravel world together. 🎉
Happy coding! 💻