How to change value of a request parameter in laravel
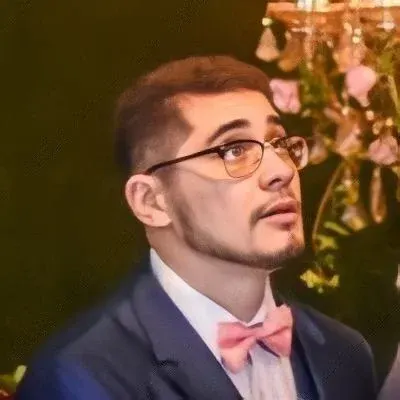
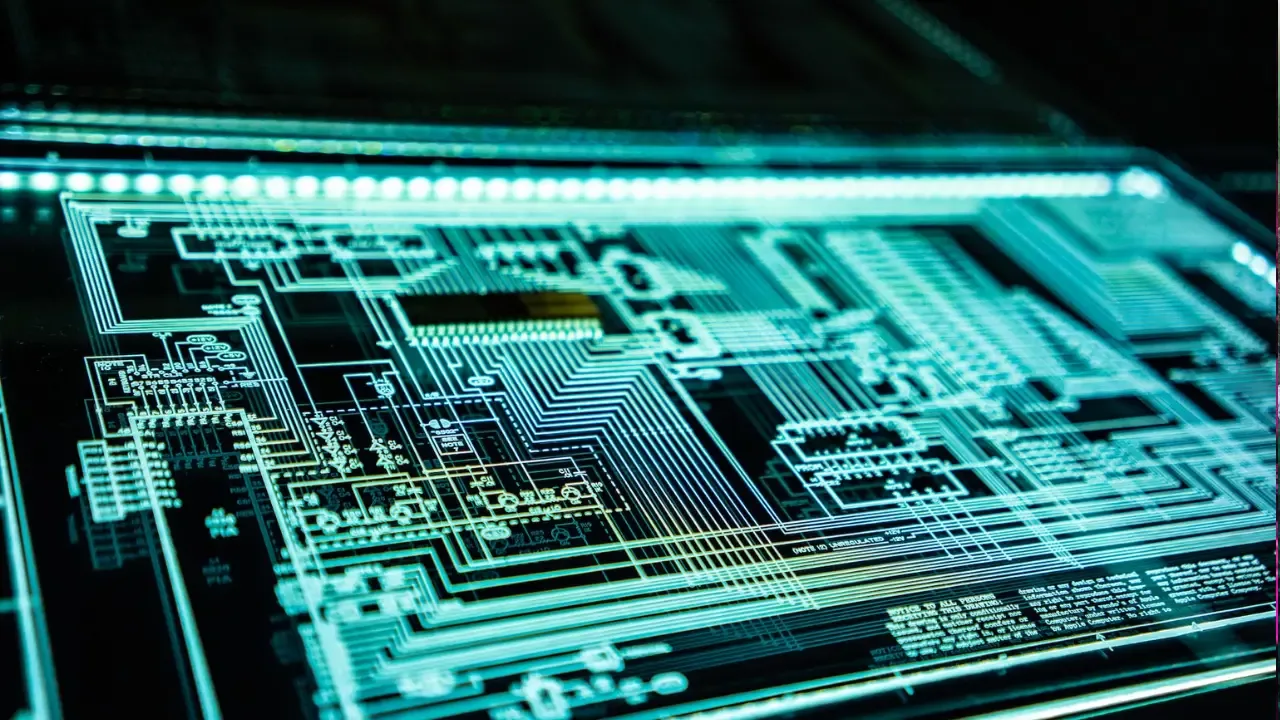
🔥📝 How to Change the Value of a Request Parameter in Laravel 🔄📥
Changing the value of a request parameter in Laravel might seem like a simple task, but it can sometimes leave developers scratching their heads. 🤔 Whether you're facing common issues or dealing with a specific problem, this guide will provide you with easy solutions and help you get back on track. So, without further ado, let's dive right into it! 💪
The Request Parameter Dilemma 😕
Consider the following code snippet:
$request->name = "My Value!";
In this example, you might expect that assigning a new value to the "name" parameter using the $request
object would work. However, sadly, that's not the case. 😟
Another approach that developers often try is:
$request->offsetSet('name', 'My Value!');
But, quite disappointingly, this doesn't work either. 😩
Easy Solution 💡
To change the value of a request parameter in Laravel, you need to understand that the $request
object is an instance of the Illuminate\Http\Request
class. Therefore, direct assignment or using the offsetSet
method won't work as expected. But don't worry – there's a simple solution! 🎉
To update the value of a request parameter, you need to utilize the request()
helper function and the merge()
method. Here's how you can do that:
$request->merge(['name' => 'My Value!']);
This one-liner does the trick! Now, you can successfully change the value of the "name" parameter. 🎉
Example Implementation ✨
Let's put this newfound knowledge into use with a practical example. Suppose you have a form containing a "name" input field, and you want to change its value based on user input. Here's how you can achieve that in your Laravel controller:
public function update(Request $request)
{
// Get the user input
$newName = $request->input('newName');
// Change the value of the "name" parameter
$request->merge(['name' => $newName]);
// Continue with your logic...
}
In this example, the user's input is retrieved using the input()
method, and then the value of the "name" parameter is changed using the merge()
method. You can then proceed with your desired logic based on the updated parameter value. 🚀
Your Turn! 🙌
Now that you've learned how to change the value of a request parameter in Laravel, why not implement this solution in your own project? As you dive into the code, you might encounter other challenges and find unique solutions. Share your experiences by leaving a comment below! 💬
Remember, knowledge is power 💪. Stay curious, keep coding, and embrace the joy of Laravel development! Happy coding! 😄✨🎉
Have any questions, comments, or suggestions? Feel free to contact us or connect with us on Twitter. We'd love to hear from you! 😊
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
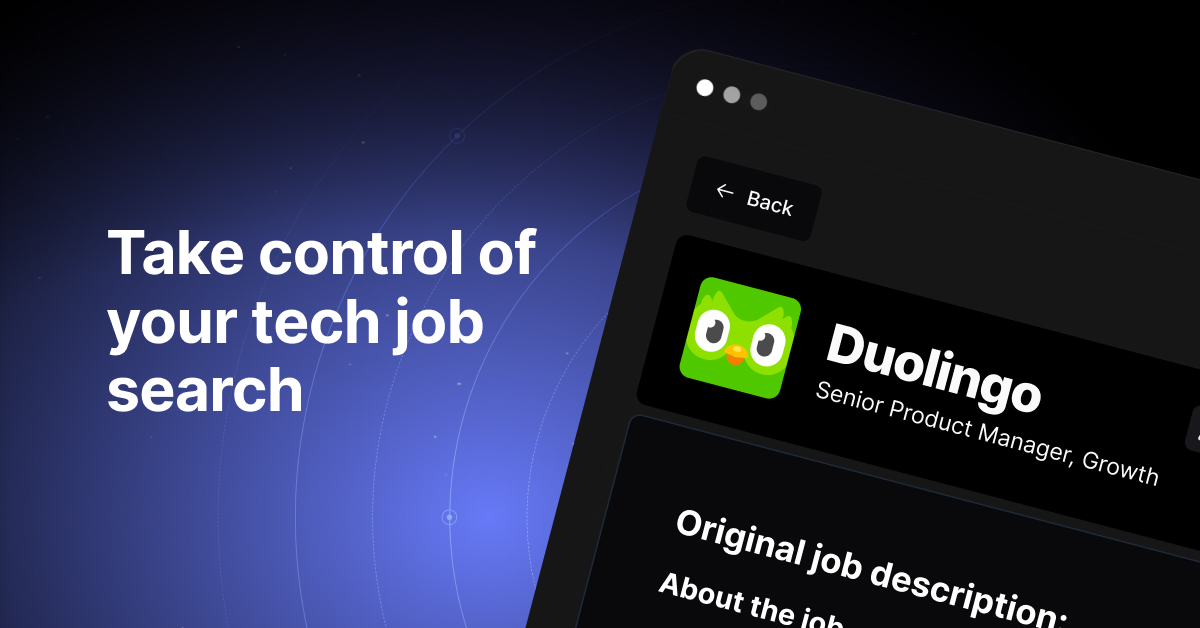