How do I pass a variable to the layout using Laravel" Blade templating?
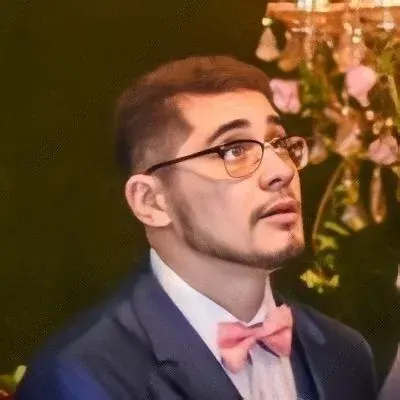
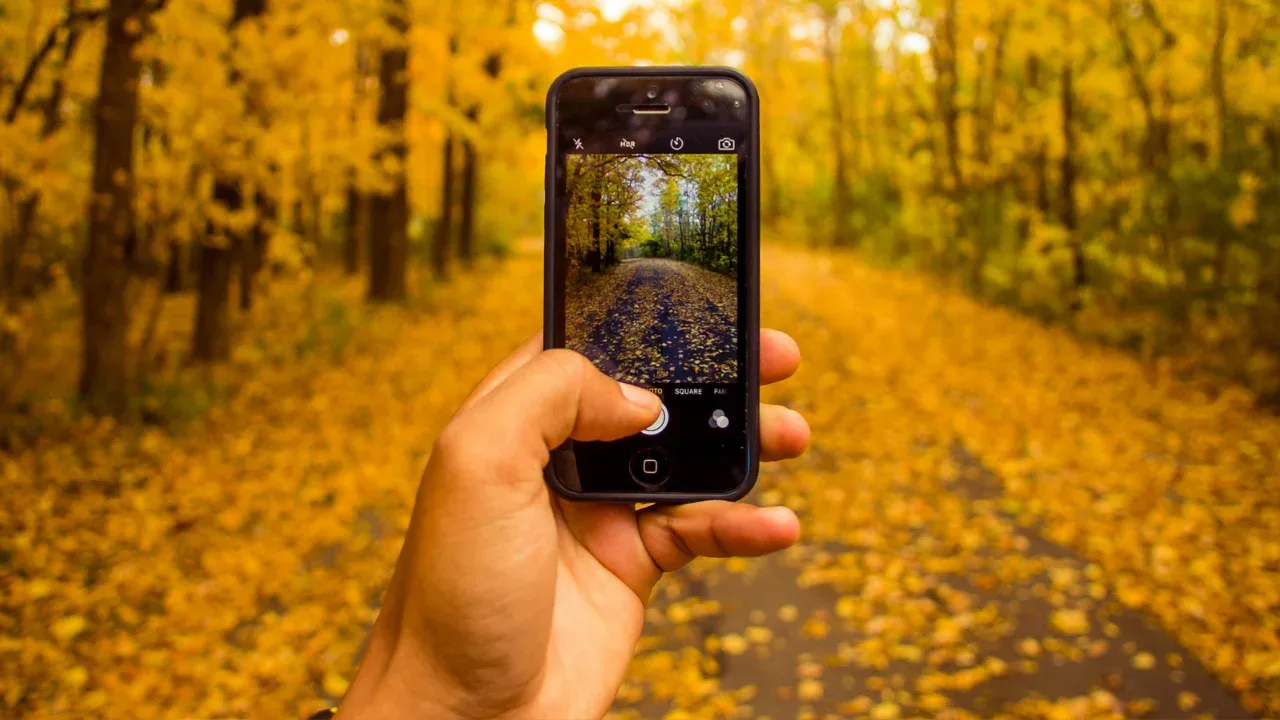
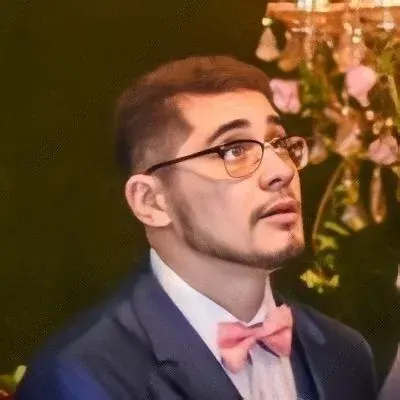
How to Pass a Variable to the Layout Using Laravel's Blade Templating? 🖥️
So, you're working with Laravel and you want to pass a variable to the layout using Blade templating. You've come to the right place! This guide will walk you through the steps and provide you with easy solutions to this common issue. Let's dive in! 🏊♂️
Understanding the Issue 🤔
In Laravel 4, the controller utilizes a Blade layout to structure the application's views. The layout contains the common elements shared across multiple views, such as the <title>
tag. The challenge lies in passing a variable to the layout itself, rather than just to the subviews.
Solution 1: Using the View Composer 🎵
One way to tackle this problem is by using a View Composer. A View Composer allows you to share data with multiple views or, in this case, the layout. Here's how you can do it:
Create a new service provider using the Artisan command:
php artisan make:provider ViewComposerServiceProvider
.Open the newly created
ViewComposerServiceProvider.php
file located in theapp/Providers
directory.In the
boot
method of the service provider, use theview
method to bind a closure to the specific view you want to pass the variable to. In this case, the layout.public function boot() { view()->composer('layouts.master', function ($view) { $view->with('title', 'Your Variable Value'); }); }
Register the service provider by adding it to the
providers
array in yourconfig/app.php
file:'providers' => [ // Other service providers... App\Providers\ViewComposerServiceProvider::class, ],
And that's it! The variable title
will now be available in your layout (layouts.master
) and can be accessed using {{ $title }}
.
Solution 2: Using View Share 🌐
Another way to pass a variable to the layout is by using the View::share
method provided by Laravel. Here's what you need to do:
Open the
app/Providers/AppServiceProvider.php
file.In the
boot
method of theAppServiceProvider
, use theView::share
method to share the desired variable with all views:public function boot() { View::share('title', 'Your Variable Value'); }
And you're done! The variable
title
will now be accessible in your layout and all other views.
Conclusion and CTA 📝
Passing a variable to the layout using Laravel's Blade templating might seem like a complex task at first, but with the right solutions, it can be easily accomplished. Remember, you can use either the View Composer approach or the View Share method.
Start using these techniques in your Laravel projects today and say goodbye to struggling with passing variables to your layout. Happy coding! 💻
Do you have any other Laravel-related questions or faced any difficulties before? Let us know in the comments below! We would love to hear from you and help you out in your coding journey. Keep exploring and keep learning! 🔍🚀