Eloquent - where not equal to
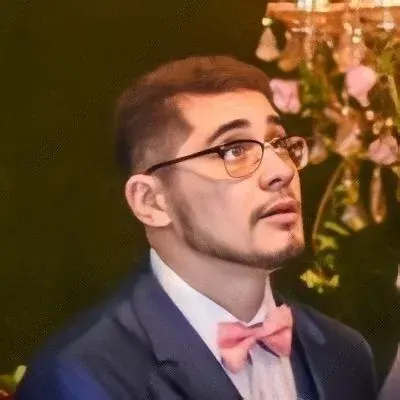
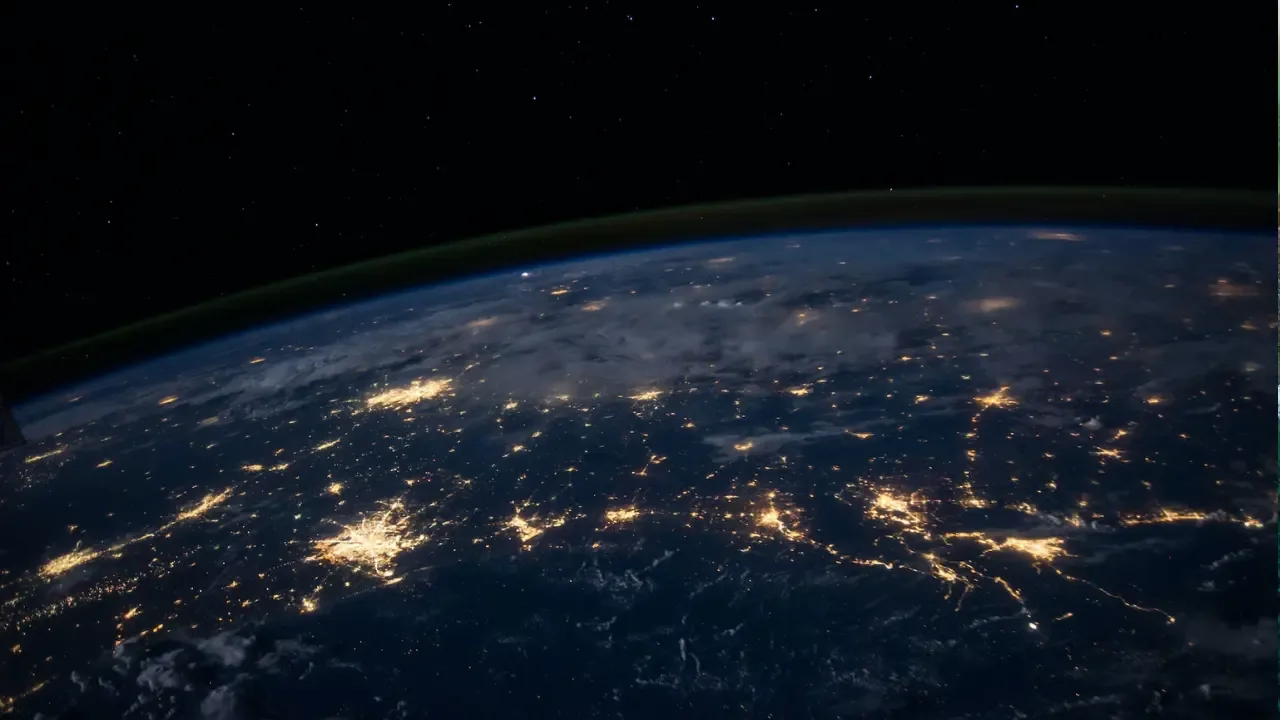
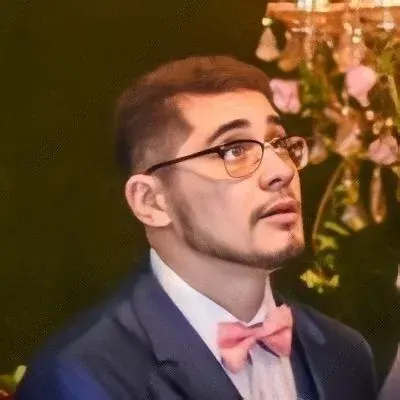
š Title: Solving the Not Equal to Problem in Eloquent for Laravel
š Hey there Laravel lovers! š Are you faced with a perplexing problem where querying records except for a specific value in Eloquent just returns a blank array? Don't worry, I've got your back! In this blog post, we'll explore how to tackle the "Not Equal to" problem in Eloquent for Laravel and provide you with easy and effective solutions. Let's dive in! šŖ
The Problem
So here's the scenario: you're running the latest version of Laravel, and you've tried a few queries to retrieve records while excluding a specific value. Your attempts may have looked something like this:
Code::where('to_be_used_by_user_id', '<>', 2)->get();
Code::whereNotIn('to_be_used_by_user_id', [2])->get();
Code::where('to_be_used_by_user_id', 'NOT IN', 2)->get();
But to your dismay, instead of getting all the records except for user_id = 2
, you end up with an empty array. š± So what's the deal? How do we tackle this issue?
The Solution
To overcome this problem, we need to understand how Laravel handles the "Not Equal to" comparison in Eloquent queries. First of all, let me clarify that the <>
or !=
operator works well for most cases, but in some instances, it may not give you the desired results, especially when handling null values.
To solve this problem, we can use the whereNotNull
method in conjunction with the where
method. Here's an example that demonstrates this approach:
Code::where('to_be_used_by_user_id', '<>', 2)
->orWhereNull('to_be_used_by_user_id')
->get();
In this query, we use orWhereNull
to include records with NULL
values for the to_be_used_by_user_id
column, in addition to those that are not equal to 2.
Alternatively, you can also utilize the whereColumn
method to compare two columns. Here's an example:
Code::whereColumn('to_be_used_by_user_id', '<>', 'user_id')
->get();
In this case, we directly compare the to_be_used_by_user_id
column with the user_id
column.
Digging Deeper (Optional)
To understand why your previous attempts didn't work as expected, let's take a closer look at your Code model:
<?php namespace App;
use Illuminate\Database\Eloquent\Model;
class Code extends Model
{
protected $fillable = ['value', 'registration_id', 'generated_for_user_id', 'to_be_used_by_user_id', 'code_type_id', 'is_used'];
public function code_type()
{
return $this->belongsTo('App\CodeType');
}
}
Based on the defined $fillable
array, it seems that the to_be_used_by_user_id
column is not guarded. Hence, when you use the Code::where('to_be_used_by_user_id', '<>', 2)->get()
query, it actually fetches all records in the table.
To tackle this, ensure that you have set appropriate restrictions for mass assignment by either using the $guarded
property or defining the $fillable
array accordingly.
Time to Take Action! š
Now that you have a clear understanding of how to deal with the "Not Equal to" problem in Eloquent for Laravel, it's time to put this knowledge into action! Start by modifying your queries using the solutions provided and see the magic happen. Don't forget to share your experience and results with us!
If you still encounter any issues or have any questions, feel free to drop a comment below, and our community of Laravel enthusiasts will be glad to assist you. Let's continue to dive deeper into Laravel and conquer its challenges together! šŖš
Remember, sharing is caring! If you found this blog post helpful, don't forget to share it with your fellow Laravel developers. Spread the knowledge and let's make the Laravel world a better place, one query at a time. Happy coding! šš
š Did you find this blog post helpful? Give us feedback or share your thoughts in the comments below. We'd love to hear from you! š
š£ Subscribe to our newsletter to stay updated with the latest Laravel tips, tutorials, and best practices. Let's take your Laravel skills to the next level!
š Want more Laravel goodness? Check out these related articles:
š» Join our community on Slack, where Laravel enthusiasts share ideas, seek help, and celebrate success stories. Don't miss out on the fun!
š© Got a burning question or need support? Contact our support team, and we'll get back to you in no time.
š Thank you for choosing our blog post as your guide! Keep rocking with Laravel and stay tuned for more awesome content. Happy coding!