Eloquent Collection: Counting and Detect Empty
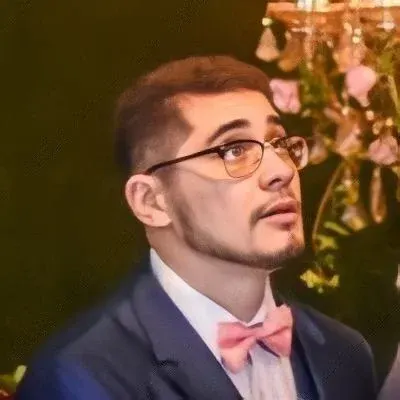
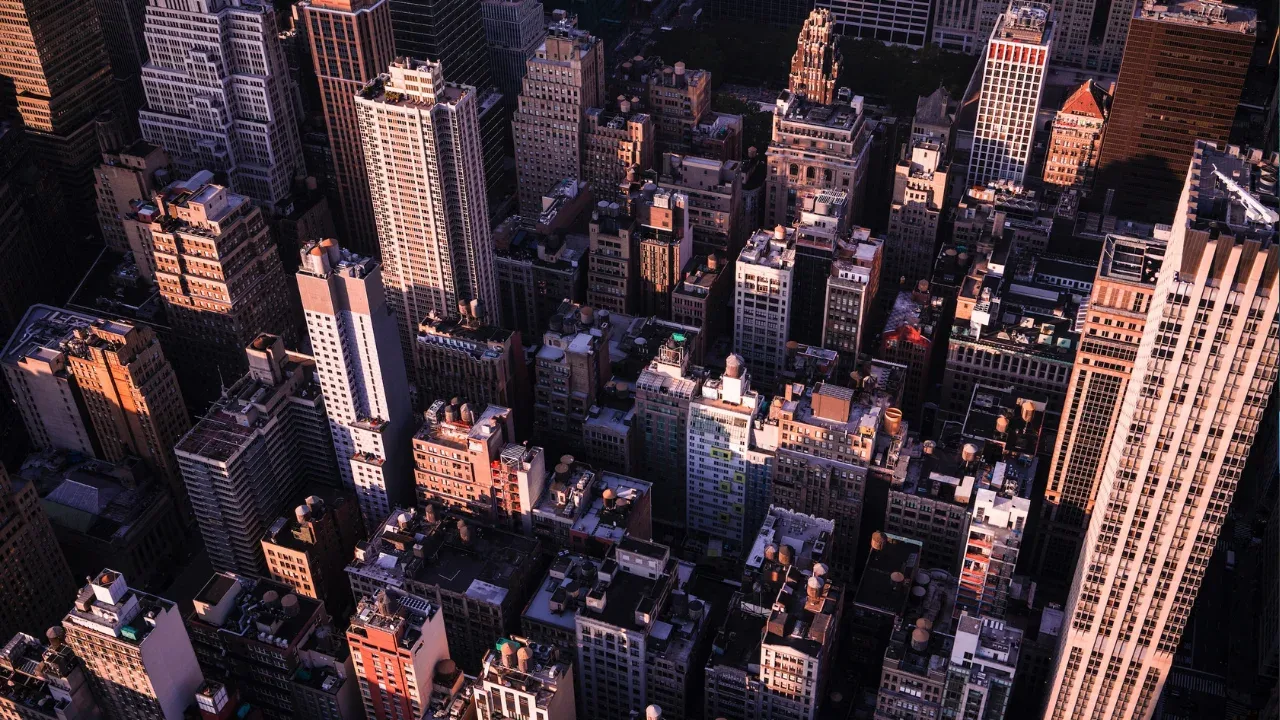
💡 Simplifying Eloquent Collection: Counting and Empty Detection
Are you an aspiring Laravel developer who's struggling with counting and detecting empty Eloquent collections? Look no further! In this guide, we'll delve into the common issues you might encounter and provide you with easy, reliable solutions. By the end of this article, you'll be ready to tackle these problems head-on and optimize your Laravel code.
🤔 Common Issues
When dealing with Eloquent collections in Laravel, it's essential to know how to properly count elements and detect whether the collection is empty. The provided context mentions using !$result
to detect emptiness and count($result)
to count elements.
However, these techniques may not cover all cases and can lead to unexpected results. Let's explore more robust solutions that Laravel recommends.
🛠️ Easy Solutions
Empty Detection
Instead of using !$result
to check if an Eloquent collection is empty, Laravel provides a dedicated method called isEmpty()
. It returns true
if the collection contains no elements, making your code more explicit and self-explanatory.
if ($result->isEmpty()) {
// Collection is empty
} else {
// Collection is not empty
}
With this simple change, you can ensure that your code behaves as expected, even in edge cases.
Counting Elements
To count the number of elements in an Eloquent collection, you might be tempted to use count($result)
as mentioned. While this approach can work, Laravel suggests using the count()
method directly on the collection. This method provides better performance, especially with large datasets.
$elementCount = $result->count();
By invoking the count()
method on the collection directly, you'll get accurate results without any unnecessary overhead.
👣 Step-by-Step Guide
Now that you know the best practices, let's summarize the steps to count elements and detect emptiness in your Eloquent collections:
Retrieve your Eloquent collection using the query builder:
$result = Model::where(...)->get();
Check if the collection is empty using the
isEmpty()
method:
if ($result->isEmpty()) {
// Collection is empty
} else {
// Collection is not empty
}
Count the elements in the collection using the
count()
method:
$elementCount = $result->count();
Utilizing these steps, you can improve the reliability and performance of your Laravel code, ensuring seamless user experiences.
💬 Join the Conversation!
We hope this guide has helped you overcome the challenges of counting and detecting empty Eloquent collections. Now, we'd love to hear from you!
Have you encountered any difficulties related to Eloquent collections? How did you solve them? Share your experiences and insights in the comments below! Let's build a supportive community and help each other become Laravel pros! 💪
Don't forget to subscribe to our newsletter to receive more helpful Laravel tips and tricks right in your inbox. Stay tuned for future articles that'll tackle more exciting challenges!
Happy coding! 😄🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
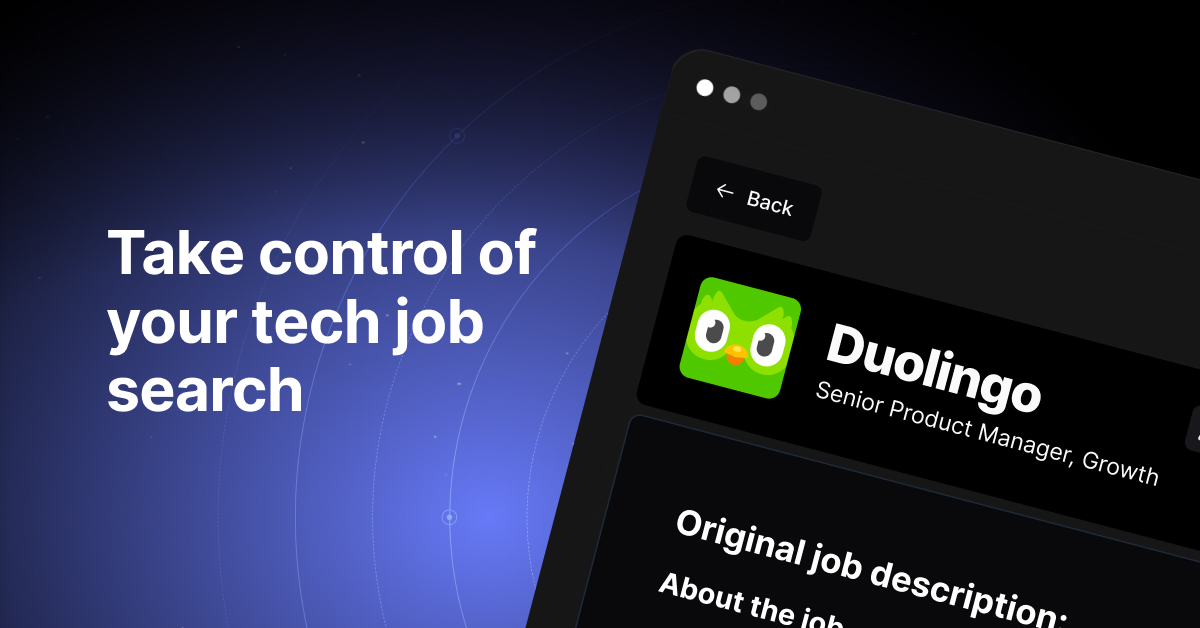