Clone an Eloquent object including all relationships?
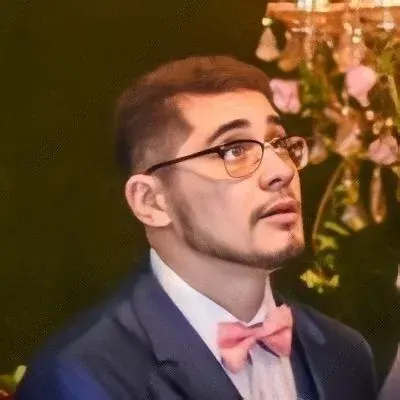
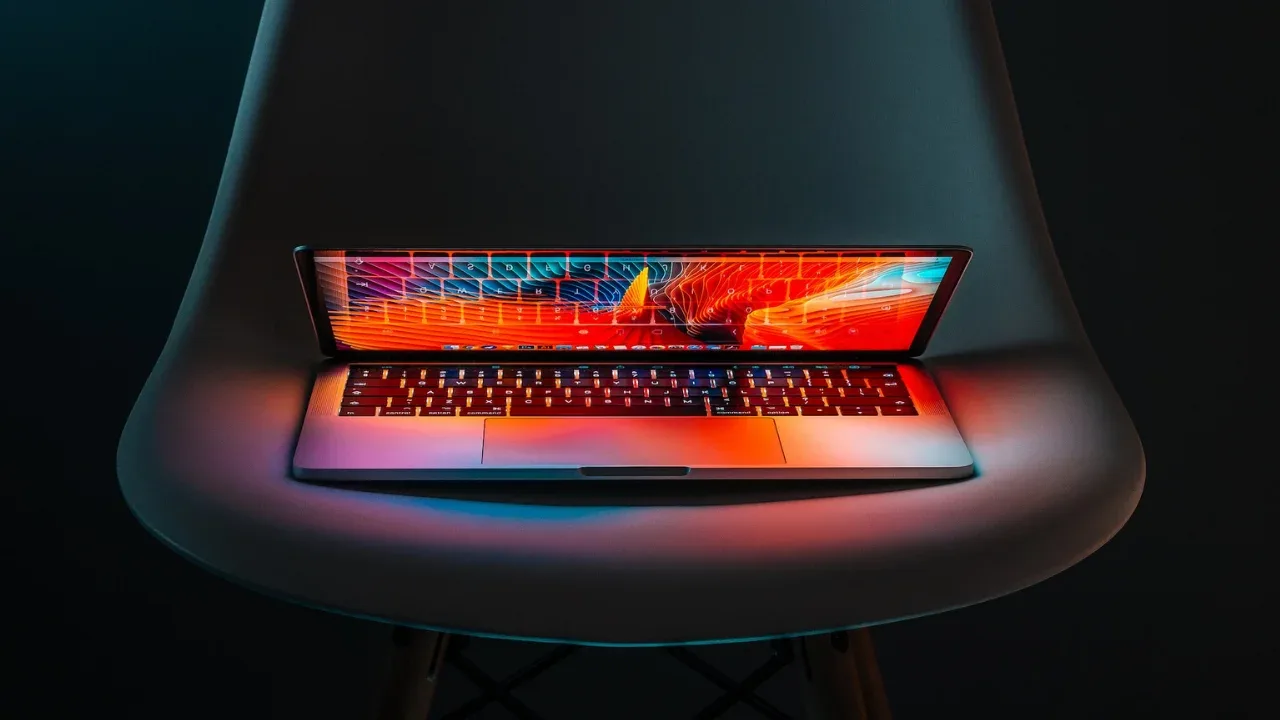
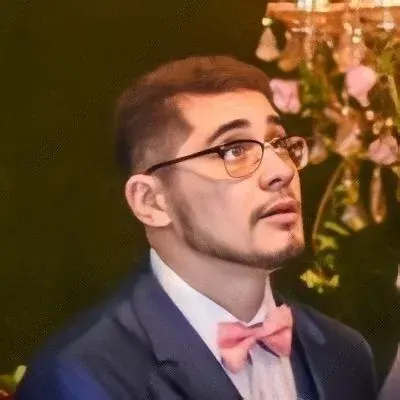
Clone an Eloquent object including all relationships?
š Are you tired of manually copying all the relationships of an Eloquent object? š Don't worry, we've got you covered! In this post, we'll explore an easy solution to clone an Eloquent object, including all its relationships. š¤©
The Problem
š¤ So, you have an Eloquent object and want to create a copy of it, preserving all its relationships intact. Let's say you have the following tables:
users ( id, name, email )
roles ( id, name )
user_roles ( user_id, role_id )
In addition to creating a new row in the users
table, with all columns except the id
being the same, you also want to create a new row in the user_roles
table, assigning the same role to the new user. š®
The Solution
š Thankfully, Laravel provides a straightforward solution for cloning Eloquent objects. Here's how you can achieve it:
Assuming you have a User model with a roles
relationship defined:
class User extends Eloquent {
public function roles() {
return $this->hasMany('Role', 'user_roles');
}
}
Now, let's say you have an existing user that you want to clone:
$user = User::find(1);
$new_user = $user->replicate();
š Voila! The replicate()
method creates a new instance of the User model, retaining all attributes of the original user, except for the id
. It also automatically copies all the relationships tied to the original user. šÆāāļø
Let's Test It!
To verify if the clone includes all the relationships, you can check the roles of the new user:
$roles = $new_user->roles;
And there you have it! The $roles
variable should contain all the roles associated with the original user. š
Conclusion
Cloning an Eloquent object, including all its relationships, is a piece of cake with Laravel. š° The replicate()
method makes it easy to create a new instance with the same attributes and relationship data. š
So, next time you find yourself needing to clone an Eloquent object, just remember to use the replicate()
method and save yourself the hassle of manually copying all the relationships. š¤©
Now it's your turn! Have you encountered any challenges when cloning Eloquent objects? How did you solve them? Share your experiences in the comments below! š¬āØ