Best Practices for Laravel 4 Helpers and Basic Functions?
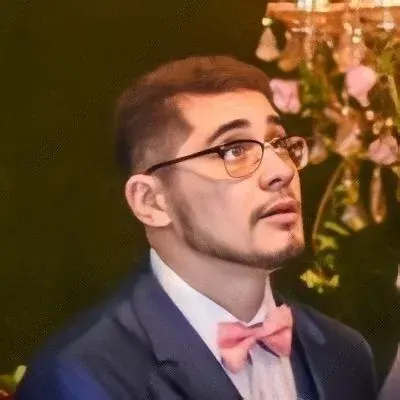
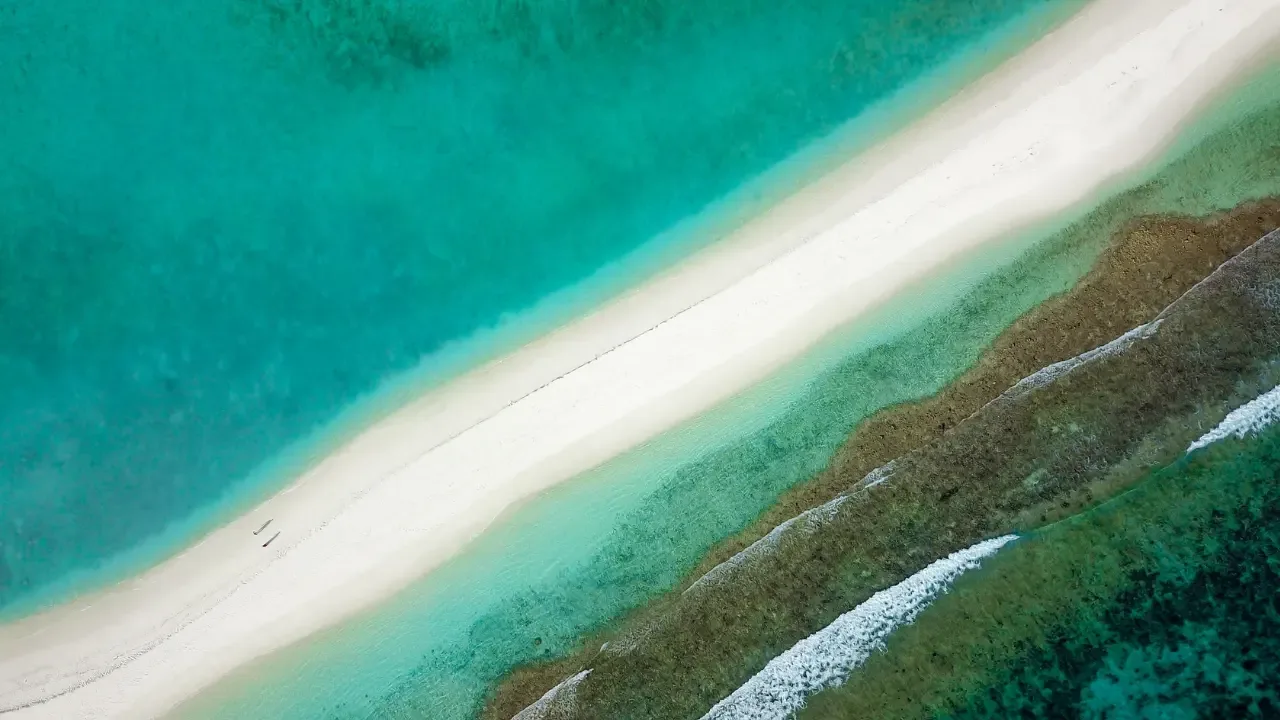
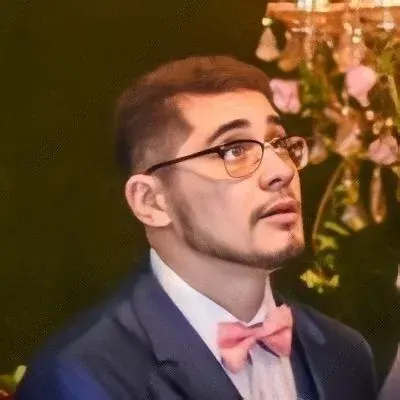
Best Practices for Laravel 4 Helpers and Basic Functions
Are you struggling to find the best place to put a global function in Laravel 4? 🤔 Do you want to make your functions easily accessible in Blade templates? We've got you covered! In this blog post, we will discuss the best practices for creating helpers and basic functions in Laravel 4 and provide easy solutions to overcome common issues. So, let's dive in and level up your Laravel game! 💪
The Problem: Where to put global functions?
Before we jump into the solutions, let's understand the problem. You might have a simple function, like date formatting, that needs to be accessible throughout your Laravel application. But where should you put it? 🤷♂️
Option 1: Facades - Too Modular?
One option you might have come across is using facades. However, facades can sometimes be considered too modular for simple functions. They require you to create a separate class, which might feel like overkill for a basic functionality.
Option 2: Library folder - Overkill?
Another suggestion you might have stumbled upon is creating a library folder and storing your functions as classes there. While this approach gives you a dedicated place for your functions, it might still seem like too much for a simple function. Plus, accessing those functions in Blade templates can be a hassle.
Now that we understand the challenges, let's explore the best practices and easy solutions to make your functions readily available everywhere!
The Best Practices: Helper functions and Blade templates
Solution 1: Helper Functions to the Rescue!
One of the best practices in Laravel 4 is to create helper functions for common functionalities. These functions can be accessed globally and make your life easier. Here's how you can create a helper function:
Create a new file, let's call it
helpers.php
, in yourapp
directory.Define your helper function(s) in this file, ensuring they are globally accessible. For example, let's create a
formatDate()
function for date formatting:function formatDate($date) { // Your date formatting logic here }
Next, you need to autoload this file. Open your
composer.json
file and add the following lines under theautoload
section:"files": [ "app/helpers.php" ]
Finally, run
composer dump-autoload
command from the terminal to update your autoload files.
Voila! 🎉 Your helper function is now globally available. You can call formatDate()
from anywhere in your Laravel application.
Solution 2: Blade Directives for Even Easier Access!
While helper functions are great, accessing them in Blade templates can still be a bit cumbersome. But fear not - Laravel provides an elegant solution called Blade directives. With Blade directives, you can directly use your helper functions in your views. Here's how:
Open your
app/start.php
file.Scroll down to the
App::missing
section, or create it if it doesn't exist.Add the following code to create a Blade directive for our
formatDate()
function:Blade::directive('formatDate', function ($expression) { return "<?php echo formatDate($expression); ?>"; });
Save the file and you're good to go! You can now use the
@formatDate
directive in your Blade templates:<p>The current date is: @formatDate(date('Y-m-d'))</p>
Conclusion
Creating helper functions and leveraging Blade directives are the best practices for making your functions readily available in Laravel 4. By following these easy solutions, you can avoid the complexities of facades or creating separate library folders, and still have clean and easily accessible code.
Now that you have learned the best practices, it's time to level up your Laravel game! 🚀 Implement these techniques in your projects and share your experiences in the comments below. We would love to hear from you. Happy coding! 💻✨