A JOIN With Additional Conditions Using Query Builder or Eloquent
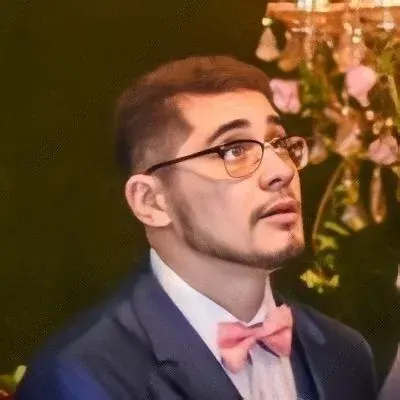
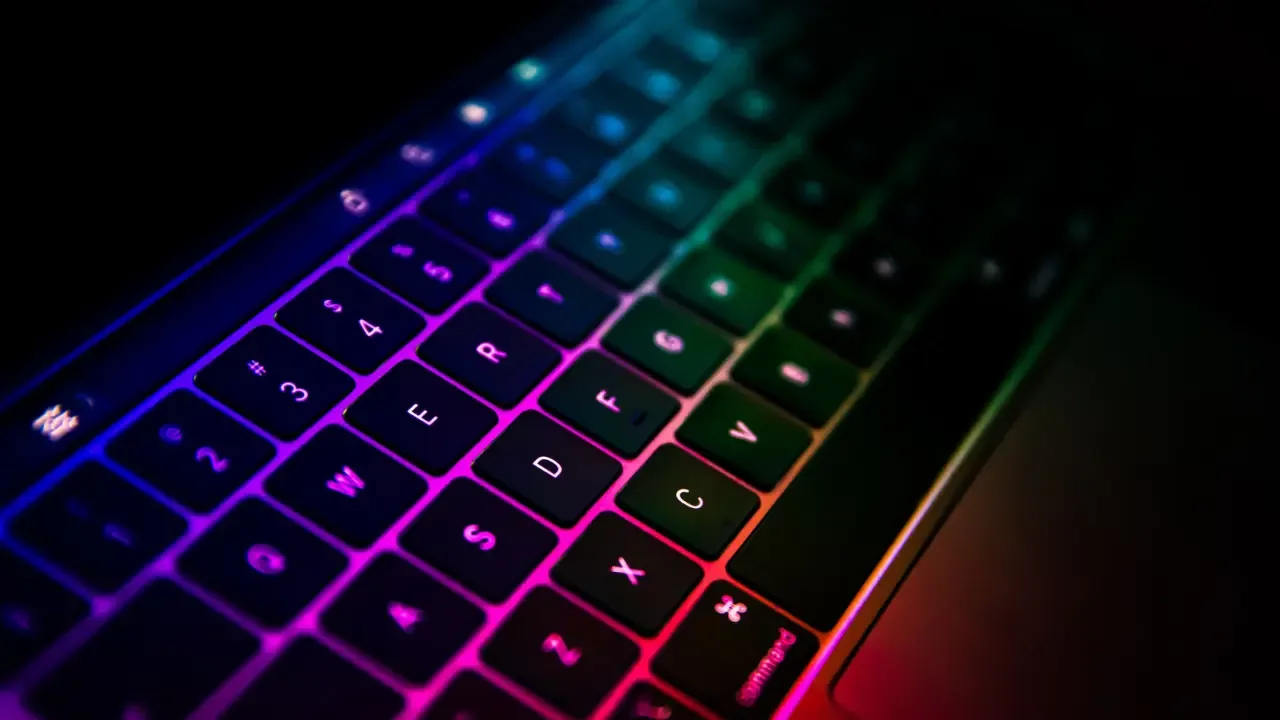
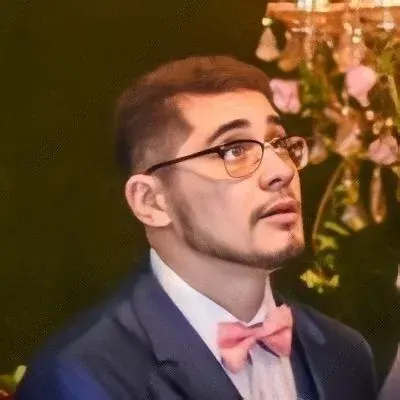
🔗 A JOIN With Additional Conditions Using Query Builder or Eloquent 🔗
Are you struggling to add additional conditions to a JOIN query using Laravel Query Builder? 😫 Don't worry, you're not alone! Many developers face this challenge when working with complex queries that require specific conditions. But fear not, because I'm here to guide you through it! 🚀
Let's start by understanding the problem at hand. You have a query that involves a JOIN, and you want to add additional conditions to it. In your current code, you are using the DB::select()
method to execute a raw SQL query. This works, but it leaves you vulnerable to SQL injection if not handled properly. Hence, you're seeking a safer solution that still gives you the desired query structure. 🤔
You've already attempted to use the Laravel Query Builder to achieve this, but the generated query and results didn't match your expectations. Let's analyze your code and identify the issues together. 💡
$results = DB::table('rooms')
->distinct()
->leftJoin('bookings', function ($join) {
$join->on('rooms.id', '=', 'bookings.room_type_id');
})
->whereBetween('arrival', array('2012-05-01', '2012-05-10'))
->whereBetween('departure', array('2012-05-01', '2012-05-10'))
->where('bookings.room_type_id', '=', null)
->get();
The generated query by Laravel is as follows:
select distinct * from `room_type_info`
left join `bookings`
on `room_type_info`.`id` = `bookings`.`room_type_id`
where `arrival` between ? and ?
and `departure` between ? and ?
and `bookings`.`room_type_id` is null
Now, you've noticed that the output doesn't have the desired structure, especially the conditions under the JOIN scope. 😣
So, to answer your first question: Yes, it is possible to add additional conditions under the JOIN using Laravel Query Builder! You're on the right track, and to get the desired output, you need to make a small adjustment to your code. 🛠️
To achieve this, you can use the on
method directly to add the additional conditions under the JOIN. Here's the modified code:
$results = DB::table('rooms')
->distinct()
->leftJoin('bookings', function ($join) {
$join->on('rooms.id', '=', 'bookings.room_type_id')
->whereBetween('bookings.arrival', ['2012-05-01', '2012-05-10'])
->orWhereBetween('bookings.departure', ['2012-05-01', '2012-05-10']);
})
->whereNull('bookings.room_type_id')
->limit(20)
->get();
🎉 Ta-da! This will give you the desired structure for your JOIN query while incorporating the additional conditions. Notice how we chained the whereBetween
and orWhereBetween
methods within the leftJoin
closure. This way, the conditions are applied specifically to the JOIN clause. Also, we simplified the where('bookings.room_type_id', '=', null)
condition by using the whereNull
method for readability.
But wait, there's more! 🙌
You also raised the question of whether it's better to use Eloquent or stick with DB::select
. Well, the decision ultimately depends on your specific use case and preferences.
If you're working with simple queries and don't need to deal with complex relationships, Eloquent ORM (Object-Relational Mapping) might be your best bet. Eloquent allows you to work with database records as objects, making your code more expressive and readable. It offers powerful features like model relationships, automatic query generation, and a query builder.
On the other hand, if you're dealing with more complex queries, need fine-grained control over the SQL, or want to leverage specific database features, using DB::select
or Query Builder might be the way to go. Query Builder offers a fluent interface for building SQL queries, giving you more flexibility in customizing your queries.
In conclusion:
For simple queries and ease of use, consider Eloquent ORM.
For complex queries or specific SQL requirements, stick with
DB::select
or Query Builder.
Now that you have the knowledge to solve this JOIN query challenge, go ahead and apply it in your code. 🤓 Remember, practice makes perfect!
If you have any further questions or need additional help, feel free to leave a comment below. Let's code together and conquer those complicated queries! 💪💻
🔐 Call-To-Action:
If you found this blog post helpful, don't forget to share it with your fellow developers who might be struggling with JOIN queries. Knowledge is meant to be shared, and helping others is what makes our coding community thrive! 😊✨
Leave a comment below and let me know if this solution worked for you, or if you have any other questions related to Laravel or web development in general. I'm here to help!
Happy coding! 🎉🚀