What is deserialize and serialize in JSON?
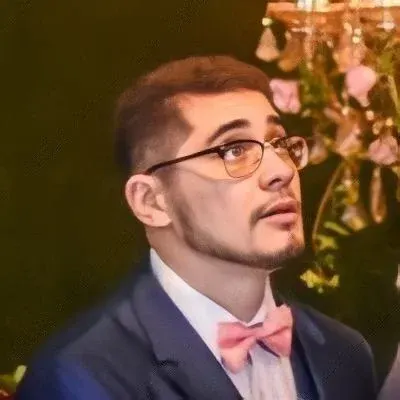

π Blog Post: What is deserialize and serialize in JSON? ππ
Welcome back to our tech blog! Today, we are here to demystify the terms "deserialize" and "serialize" in the context of JSON. Don't worry if you're scratching your head right now β we've got you covered! π‘π€
First things first, let's break down these terms:
π What is Deserialization in JSON? Deserialization refers to the process of taking data in its serialized form and converting it back into its original format. In simpler terms, it's like transforming a complex series of characters (serialized data) into a usable object that we can work with. πβ‘οΈπ€
For example, imagine you have a JSON string that represents a user object:
{
"name": "John Doe",
"age": 25,
"email": "johndoe@example.com"
}
By deserializing this JSON string, we can convert it into a user object with properties like name
, age
, and email
. This object can then be easily manipulated and used within our code. π₯π»π
π‘ Quick Tip: Deserialization is all about transforming JSON data into a usable object.
π What is Serialization in JSON? On the flip side, serialization refers to the process of converting an object into a serialized string representation (often in JSON format). Essentially, we are taking an object and converting it into a format that can be easily stored, transmitted, or persisted. πβ¬ οΈππ»
Continuing with our user object example, let's say we have an object like this:
const user = {
name: "John Doe",
age: 25,
email: "johndoe@example.com"
};
By serializing this object using JSON, we can convert it into a JSON string:
{
"name": "John Doe",
"age": 25,
"email": "johndoe@example.com"
}
This serialized JSON string can then be sent over a network, stored in a database, or used in various other ways. Ultimately, serialization allows us to convert objects into a portable format. π¬πΆποΈπ‘
π‘ Quick Tip: Serialization helps convert an object or data structure into a JSON string for storage or transmission.
π§ Common Issues and Solutions in Deserialization and Serialization: Now that we understand what deserialization and serialization mean, let's address some common issues and provide easy solutions for them:
Issue 1: Incorrect JSON Structure If you encounter errors while deserializing or serializing JSON, the most common cause is an incorrect JSON structure. Make sure the keys and values are properly formatted and separated by colons and commas.
Solution: Validate your JSON using online tools or libraries like JSONLint
or JSON.Net
to ensure the structure is correct.
Issue 2: Missing or Extra Data Another challenge when working with JSON deserialization is missing or extra data. This occurs when the JSON structure doesn't match the object structure during deserialization.
Solution: Ensure that your JSON properties match the object's properties exactly. Consider using attributes or annotations (available in most programming languages) to map JSON properties to object properties accurately.
Issue 3: Circular References In certain cases, objects may have circular references, which can lead to infinite loops while serializing or deserializing JSON.
Solution: Use libraries or frameworks that support circular reference handling, like Jackson
for Java or Newtonsoft.Json
for .NET. Alternatively, you can exclude specific properties from serialization using attributes or configuration options.
π£ Call-to-Action: Understanding deserialization and serialization in JSON is crucial for working with APIs, databases, and more! If you found this blog post helpful, share it with your fellow developers and let us know your thoughts in the comments below. We'd love to hear about your experiences and any additional tips you might have! π²ππ¬
That brings us to the end of this guide. We hope that this post has shed some light on the concepts of deserialization and serialization in JSON and provided easy solutions to common challenges. Until next time, happy coding! π»π
π Link to more tech-related articles: TechBlog.com
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
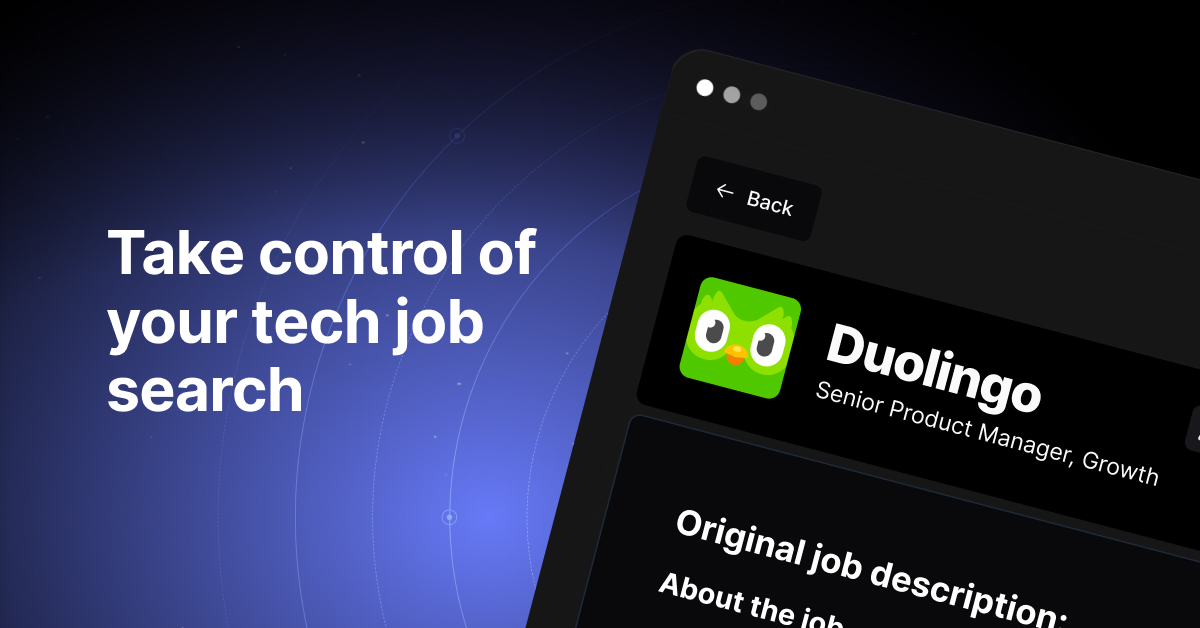