Reading in a JSON File Using Swift
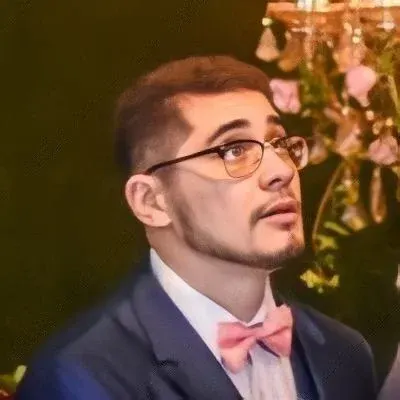
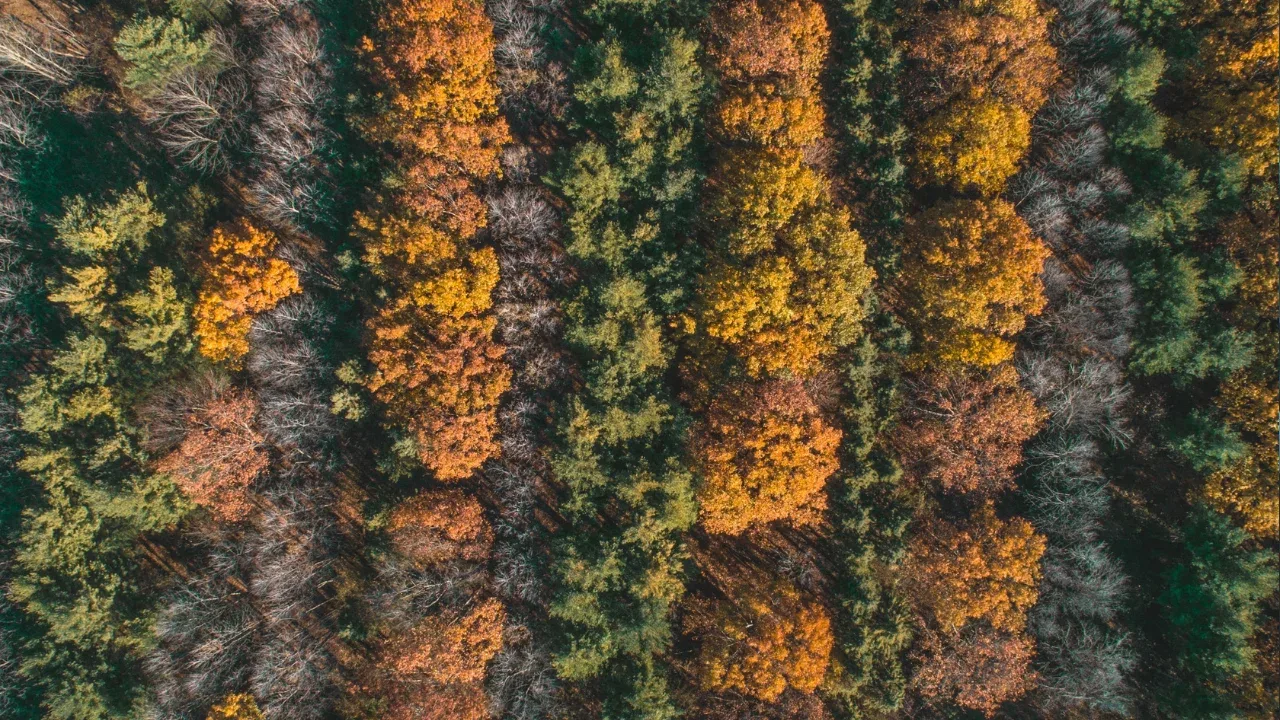
Reading in a JSON File Using Swift: A Quick Guide 📚✨
So you've been struggling with reading a JSON file into Swift, huh? Don't worry, we've all been there! But fret not, my friend, for I am here to guide you in the right direction. 🤓
The Problem 🧐
Our fellow coder Krivvenz has faced the same dilemma. After spending days searching and trying different methods, they still haven't been able to read their JSON file into Swift. The file, named test.json
, contains a list of people with their respective details.
The Solution 💡
Well, Krivvenz, fear not because Swift has got your back! Here's how you can deserialize the JSON file and put it in an accessible Swift object:
Make sure you have your
test.json
file in the Documents directory.let file = "test.json" let dirs = NSSearchPathForDirectoriesInDomains(.documentDirectory, .allDomainsMask, true) guard let dir = dirs.first else { print("Oops! Directory not found.") return } let path = URL(fileURLWithPath: dir).appendingPathComponent(file)
Read the contents of the file as
Data
.do { let jsonData = try Data(contentsOf: path) } catch { print("Oops! Error reading JSON file.") return }
Deserialize the JSON data into a Swift object using
JSONDecoder
.let jsonDecoder = JSONDecoder() guard let jsonObject = try? jsonDecoder.decode([String: [Person]].self, from: jsonData) else { print("Oops! Error deserializing JSON data.") return }
Access your deserialized object!
guard let people = jsonObject["person"] else { print("Oops! Error accessing the people array.") return } // Now you can play around with the people array as you please! 🎉 for person in people { print(person.name) print(person.age) print(person.employed) }
Conclusion 🎉
Voila! You've done it! You successfully read your JSON file into Swift and put it into an accessible object. 🙌🏼 Now you can play around with the data and do whatever you desire.
Remember, don't hesitate to seek help when you're stuck, just like Krivvenz did. And if you ever find yourself searching high and low for answers, don't forget that Stack Overflow is your best friend! 🥳
If you still have any questions or need further guidance, feel free to drop a comment below or share your thoughts. Happy coding! 😄👩💻👨💻
Keep explorin', keep codin'! 👩💻💪🌟✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
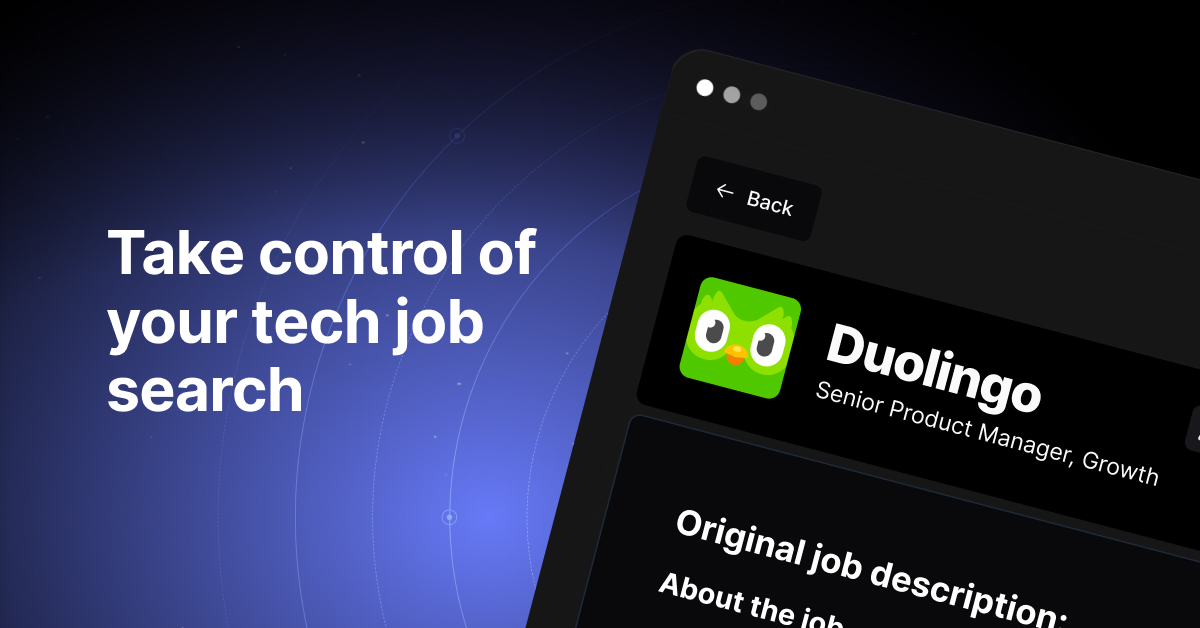