.NET NewtonSoft JSON deserialize map to a different property name
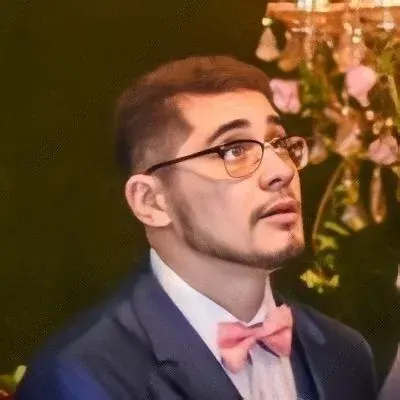
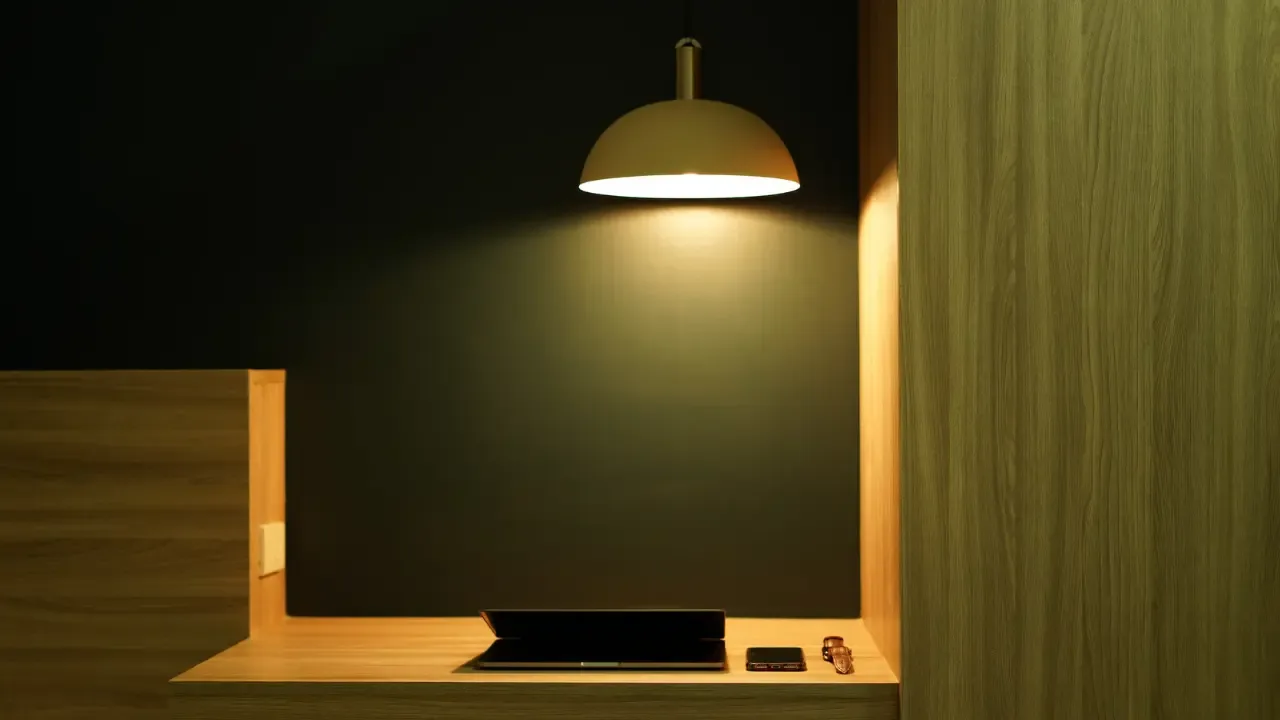
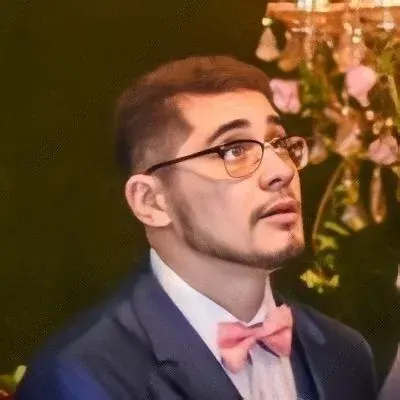
💡 Solving the Problem of Renaming JSON Properties in .NET NewtonSoft
When working with JSON data in .NET, we often encounter situations where we want to map the property names in the JSON to different names in our .NET classes. This can be especially useful when the JSON data is being provided by an external party and the property names don't match our desired naming conventions. In this blog post, we'll explore how to use .NET NewtonSoft to deserialize JSON data and map property names to different names in our .NET classes.
📜 The Problem: Renaming Property Names in JSON
In our specific scenario, we have a JSON string that represents a list of teams and their attributes. The JSON looks like this:
{
"team":[
{
"v1":"",
"attributes":{
"eighty_min_score":"",
"home_or_away":"home",
"score":"22",
"team_id":"500"
}
},
{
"v1":"",
"attributes":{
"eighty_min_score":"",
"home_or_away":"away",
"score":"30",
"team_id":"600"
}
}
]
}
We have defined our .NET classes to deserialize this JSON as follows:
public class Attributes
{
public string eighty_min_score { get; set; }
public string home_or_away { get; set; }
public string score { get; set; }
public string team_id { get; set; }
}
public class Team
{
public string v1 { get; set; }
public Attributes attributes { get; set; }
}
public class RootObject
{
public List<Team> team { get; set; }
}
The problem is that we don't like the names of the classes and fields in our .NET code. We would prefer to have the Attributes
class be named TeamScore
, and we want to remove the underscores from the field names and give them proper names.
🛠️ The Solution: Renaming Property Names in .NET NewtonSoft
To solve this problem, we can make use of the JsonProperty
attribute provided by .NET NewtonSoft. This attribute allows us to specify the name of the JSON property that should be mapped to a specific field or property in our .NET class.
Here's how we can modify our .NET classes to achieve the desired mapping:
public class TeamScore
{
[JsonProperty("eighty_min_score")]
public string EightyMinScore { get; set; }
[JsonProperty("home_or_away")]
public string HomeOrAway { get; set; }
[JsonProperty("score")]
public string Score { get; set; }
[JsonProperty("team_id")]
public string TeamId { get; set; }
}
public class Team
{
public string v1 { get; set; }
[JsonProperty("attributes")]
public TeamScore TeamScore { get; set; }
}
public class RootObject
{
[JsonProperty("team")]
public List<Team> Teams { get; set; }
}
In the modified code, we have renamed the Attributes
class to TeamScore
and adjusted the field names according to our preference using the JsonProperty
attribute. The JsonProperty
attribute specifies the name of the JSON property that should be mapped to the corresponding field or property in our .NET class.
🚀 Putting It All Together
With our updated .NET classes, we can now use .NET NewtonSoft to deserialize the JSON data and ensure that the property names are properly mapped:
string jsonText = "your JSON string here"; // Replace with your JSON string
RootObject root = JsonConvert.DeserializeObject<RootObject>(jsonText);
The JsonConvert.DeserializeObject
method from .NET NewtonSoft takes care of deserializing the JSON data into our .NET objects, correctly mapping the property names as specified in our modified classes.
📣 Conclusion and Call-to-Action
In this blog post, we've explored how to solve the problem of renaming property names in JSON data using .NET NewtonSoft. By making use of the JsonProperty
attribute, we can easily map JSON properties to different names in our .NET classes.
Next time you encounter a similar situation where the JSON property names don't match your desired naming convention, remember to use the JsonProperty
attribute to ensure proper mapping.
Do you have any other JSON-related questions or topics you'd like us to cover in future blog posts? Let us know in the comments section!