is there a require for json in node.js
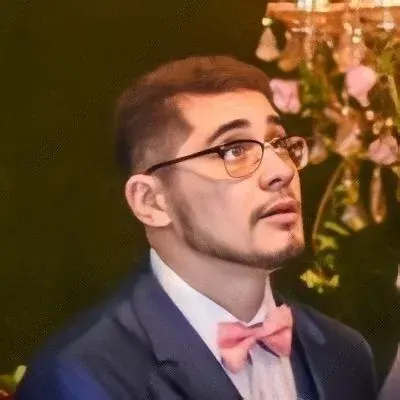
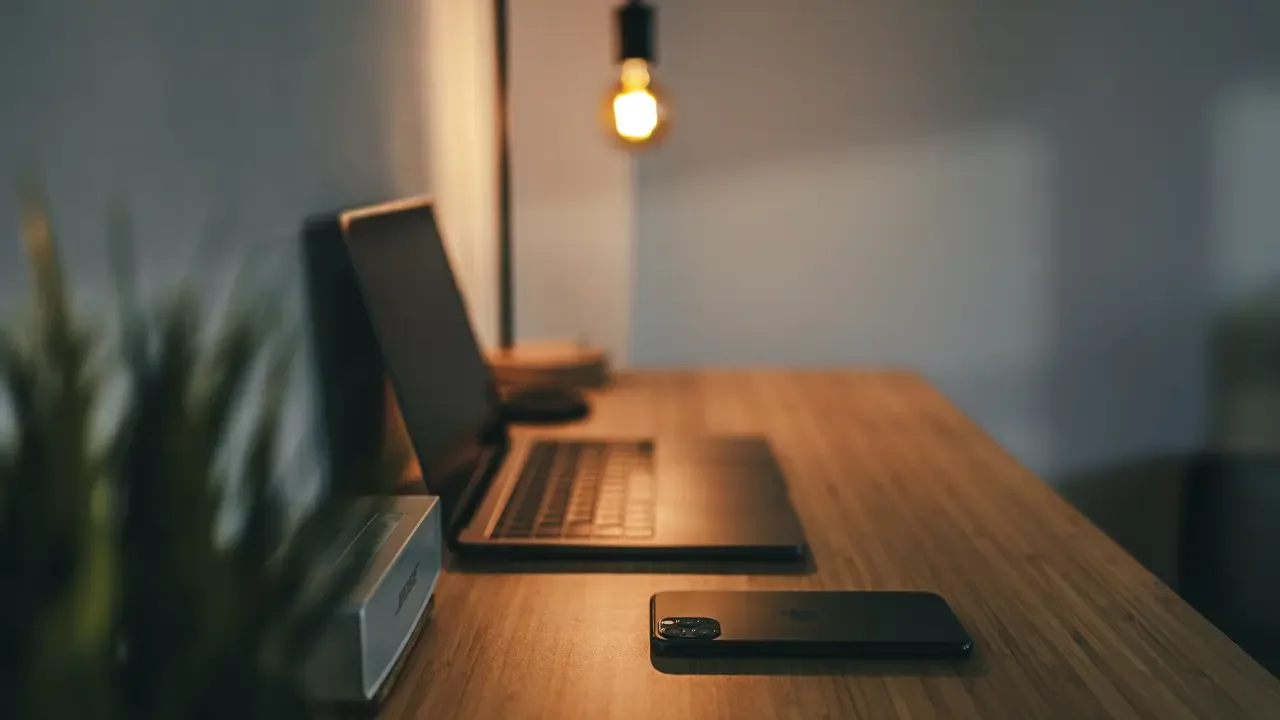
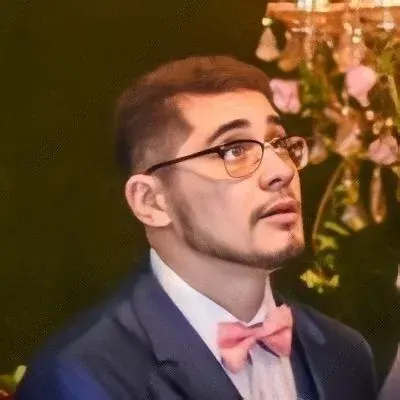
Is There a Require for JSON in Node.js? 🤔
If you've been working with Node.js and JavaScript, you might have come across the need to include JSON files in your code. While requiring another JavaScript file is as simple as using the require
function, you might be wondering if there's a similar approach to include JSON files. In this blog post, we'll address this common issue and provide you with easy solutions to include and work with JSON files in your Node.js projects. Let's get started! 💪
The "Ugly" Way: Using readFileSync and __dirname 🙃
Before we dive into more elegant solutions, let's quickly touch upon the approach mentioned in the question. You can currently use the readFileSync
function along with the __dirname
variable to read and access JSON files in the same directory as your JavaScript source file. While this solution works, it can be considered by some as less than ideal. 📁
The Require Magic: json() Function 🎩
But fear not! Node.js provides a nifty solution that allows you to require JSON files directly, making your code cleaner and more maintainable. You can take advantage of the built-in json
function to achieve this. Here's how:
Define the JSON file you want to include in a variable:
const data = require('./data.json');
🚀 That's it! You can now use the
data
object just like any other JavaScript object, accessing its properties and working with its data.
Example: Accessing JSON Data 📑
Let's walk through a simple example to solidify our understanding. Assume we have a JSON file called data.json
with the following contents:
{
"name": "John Doe",
"age": 25,
"occupation": "Web Developer"
}
In our JavaScript file, we can include and utilize this JSON file as follows:
const data = require('./data.json');
console.log(`Name: ${data.name}`);
console.log(`Age: ${data.age}`);
console.log(`Occupation: ${data.occupation}`);
Executing this code will output:
Name: John Doe
Age: 25
Occupation: Web Developer
It's that simple! 🎉
Better with ES Modules: import Syntax 🌟
Node.js has introduced support for ECMAScript (ES) Modules, enabling the use of the import
statement alongside the traditional require
. If you're using ES Modules in your project, you have an even cleaner solution.
To include a JSON file using ES Modules, follow these steps:
In your JavaScript file, import the JSON file like this:
import data from './data.json';
You can now use the
data
object as before.
Conclusion and Call-to-Action 🌈
In this blog post, we've addressed the common issue of including JSON files in Node.js and provided easy solutions to make your code more elegant and maintainable. Whether you choose to use the json
function with require
or the import
syntax with ES Modules, you can now effortlessly work with JSON data in your projects. 🙌
So go ahead and give it a try! 🚀 If you found this blog post helpful, why not share it with your fellow developers? Let them know about these powerful techniques for working with JSON in Node.js. And if you have any questions or suggestions, feel free to leave a comment below. Happy coding! 😊✨