How to convert a JSON string to a dictionary?
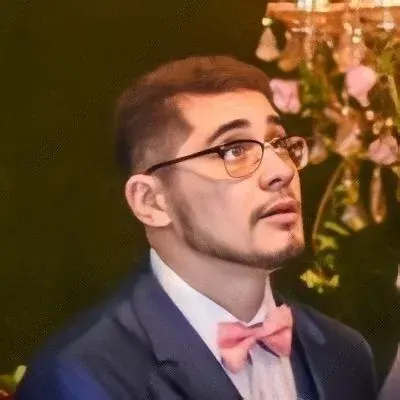
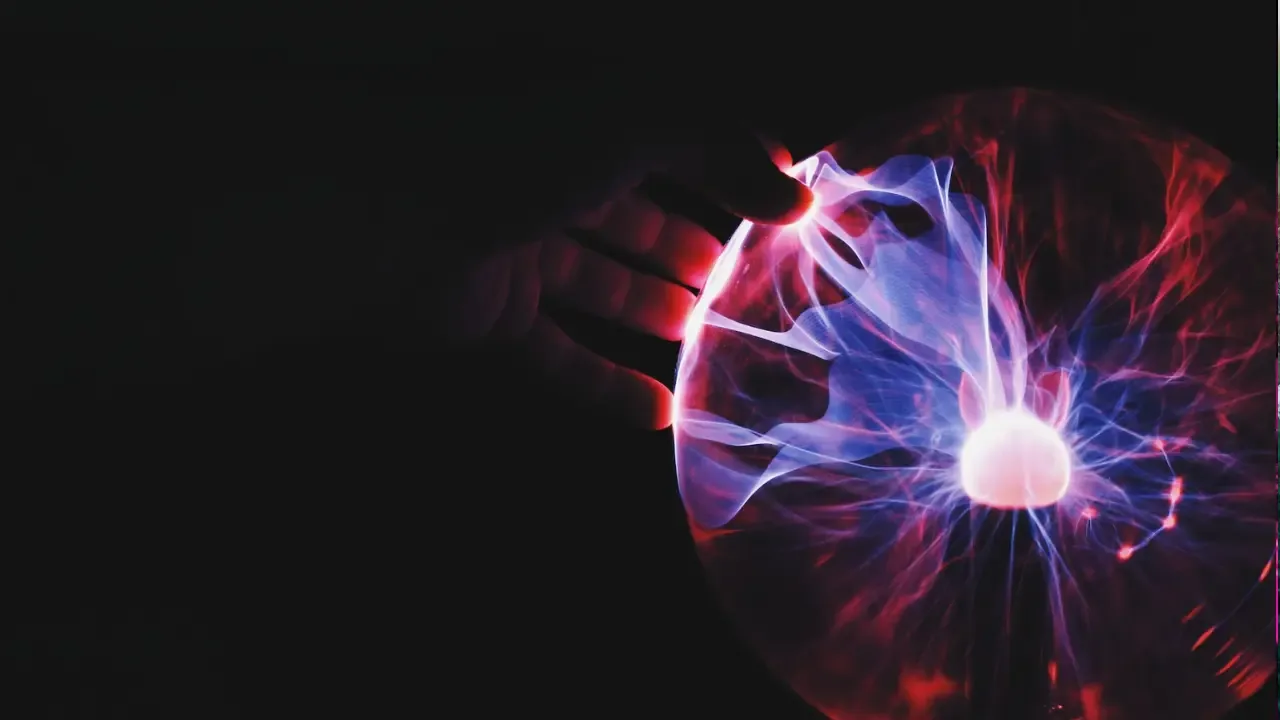
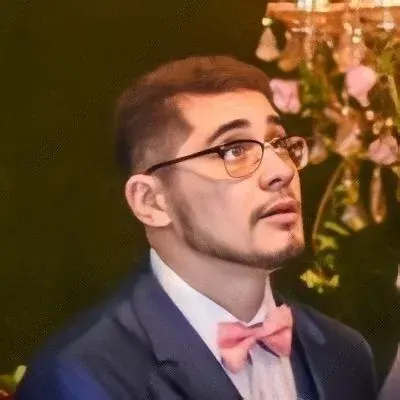
How to Convert a JSON String to a Dictionary: A Simple Guide
Are you struggling to convert a JSON string to a dictionary format in your Swift or Objective-C project? Don't worry, you're not alone! This is a common issue that developers face when dealing with JSON data. Luckily, there are easy solutions available to help you overcome this problem and get your code running smoothly. In this blog post, we'll walk you through the process step-by-step and provide you with some helpful examples. So, let's get started!
Understanding the Problem
When trying to convert a JSON string to a dictionary, you may encounter an error message that looks something like this:
Cannot convert expression's type (@lvalue NSData,options:IntegerLitralConvertible ...
This error occurs because the NSJSONSerialization.JSONObjectWithData
method expects the options
parameter to be of type NSJSONReadingOptions
rather than an integer literal. To fix this, you need to provide the correct options argument.
The Swift Solution
In Swift, you can convert a JSON string to a dictionary by making use of the NSJSONSerialization
class. Here's an example of how you can write a function to perform this conversion:
func convertStringToDictionary(text: String) -> [String: Any]? {
guard let data = text.data(using: .utf8) else {
return nil
}
do {
if let json = try JSONSerialization.jsonObject(with: data, options: []) as? [String: Any] {
return json
}
} catch {
print("Error converting JSON string to a dictionary: \(error)")
}
return nil
}
Here's how you can use this function:
let jsonString = "{\"name\":\"John\",\"age\":30}"
if let dictionary = convertStringToDictionary(text: jsonString) {
// Do something with the dictionary...
print(dictionary)
} else {
print("Failed to convert JSON string to a dictionary.")
}
The Objective-C Solution
If you're working with Objective-C, you can achieve the same result by using the NSJSONSerialization
class as well. Here's an example of an Objective-C function that converts a JSON string to a dictionary:
- (NSDictionary *)convertStringToDictionary:(NSString *)string {
NSError *error;
NSData *data = [string dataUsingEncoding:NSUTF8StringEncoding];
id jsonObject = [NSJSONSerialization JSONObjectWithData:data options:0 error:&error];
if ([jsonObject isKindOfClass:[NSDictionary class]]) {
return jsonObject;
}
NSLog(@"Error converting JSON string to a dictionary: %@", error);
return nil;
}
And here's how you can utilize this function:
NSString *jsonString = @"{\"name\":\"John\",\"age\":30}";
NSDictionary *dictionary = [self convertStringToDictionary:jsonString];
if (dictionary) {
// Do something with the dictionary...
NSLog(@"%@", dictionary);
} else {
NSLog(@"Failed to convert JSON string to a dictionary.");
}
Wrap Up and Engage!
By following these straightforward solutions, you should now be able to convert a JSON string to a dictionary in both Swift and Objective-C. If you have any questions or encounter any issues, don't hesitate to reach out for help. Happy coding!
Now, we want to hear from you! Have you ever struggled with converting a JSON string to a dictionary in your projects? What solution did you find most helpful? Share your experiences in the comments below and let's help each other out!