How do I send a JSON string in a POST request in Go
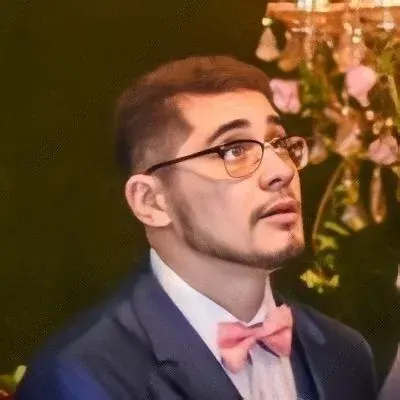
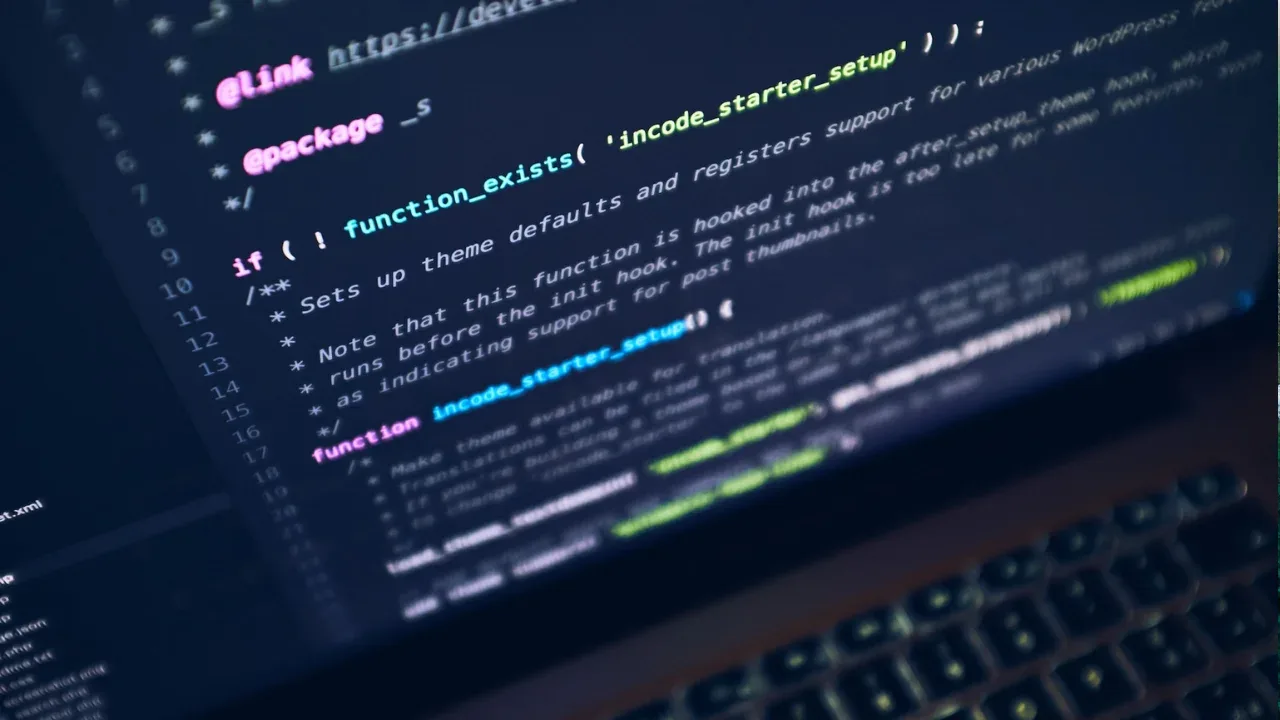
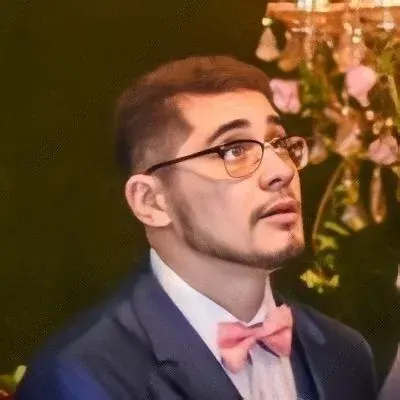
How to Send a JSON String in a POST Request in Go 🚀
So, you're trying to send a JSON string in a POST request in Go, but for some reason, it's not working. Don't worry, we've got you covered! In this blog post, we'll address common issues, provide easy solutions, and help you understand how to send a JSON string successfully. Let's dive in! 💪
The Problem 😫
From the context you provided, it seems like you're using the napping library to send your POST request. However, the code you shared is not properly sending the JSON string. You mentioned that the JSON string can be different in each call, and you can't use a struct
for this. So, how can you send a dynamic JSON string?
The Solution 💡
To send a dynamic JSON string in a POST request, we need to make a few adjustments to your code. Let's break it down step by step.
Step 1: Import the required packages
First, make sure you import the necessary packages for working with JSON and handling HTTP requests:
import (
"encoding/json"
"fmt"
"github.com/jmcvetta/napping"
"log"
"net/http"
)
Step 2: Prepare the JSON string
Since your JSON string can vary in each call, the best way to handle it is by using a map[string]interface{}
to represent the data dynamically. Here's an example of how you can do it:
var jsonData = map[string]interface{}{
"title": "Buy cheese and bread for breakfast.",
}
jsonBytes, err := json.Marshal(jsonData)
if err != nil {
log.Fatal(err)
}
jsonStr := string(jsonBytes)
In this example, we create a map jsonData
with the key-value pair representing your JSON data. Then, we marshal it into a byte slice using json.Marshal
. Finally, we convert the byte slice to a string for easier use later on.
Step 3: Send the POST request
Now that we have the JSON string prepared, let's modify the code to send the POST request correctly:
url := "http://restapi3.apiary.io/notes"
fmt.Println("URL:", url)
s := napping.Session{}
var headers http.Header
headers.Set("Content-Type", "application/json")
headers.Set("X-Custom-Header", "myvalue")
s.Header = headers
resp, err := s.Post(url, &jsonStr, nil, nil)
if err != nil {
log.Fatal(err)
}
fmt.Println("Response Status:", resp.Status())
fmt.Println("Response Headers:", resp.HttpResponse().Header)
fmt.Println("Response Body:", resp.RawText())
In this modified code, we set the Content-Type
header to application/json
to let the server know that we're sending JSON data. We also include the X-Custom-Header
value as you did in your code. We pass the &jsonStr
variable as the payload for the POST request.
Conclusion and Call-to-Action 🎉
You did it! Now you know how to send a dynamic JSON string in a POST request using Go. By following the steps mentioned above, you can ensure that your JSON string is sent correctly to the server.
If you found this blog post helpful, share it with your fellow developers who might be struggling with the same problem. And don't forget to subscribe to our newsletter for more useful tips and tricks on Go development. Happy coding! 💻🙌
Author: Your Name Website: Your Website Twitter: Your Twitter Handle LinkedIn: Your LinkedIn Profile