How do I consume the JSON POST data in an Express application
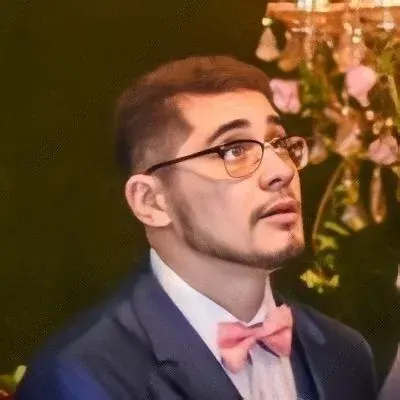
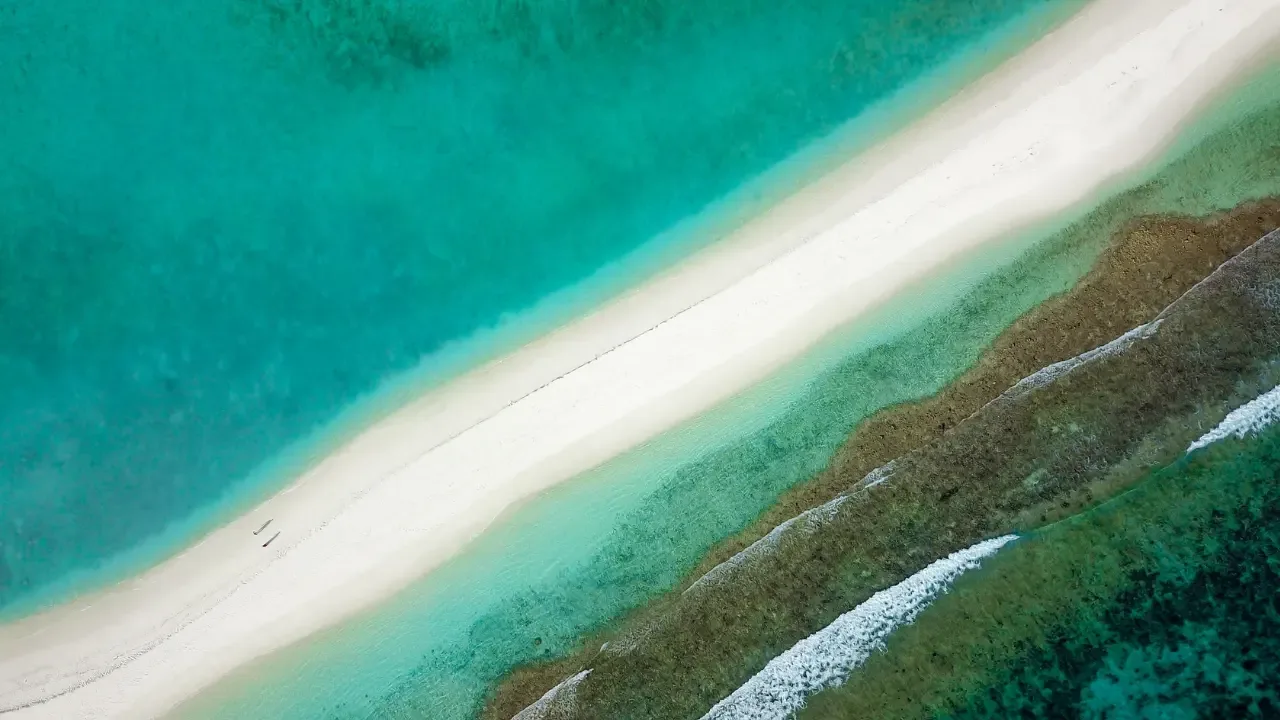
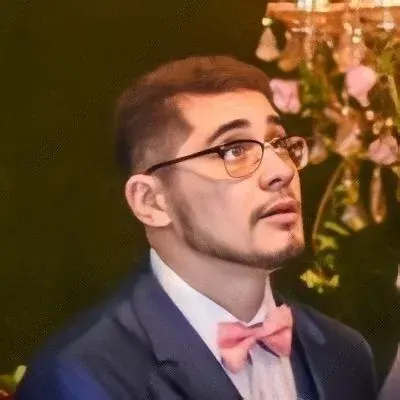
📝📲🚀 How to Consume JSON POST Data in an Express Application
Are you struggling to consume JSON POST data in your Express application? 🤔 Don't worry, we've got you covered! In this guide, we'll walk you through the common issues, provide easy solutions, and give you a compelling call-to-action to engage with our readers. Let's get started! 💪
Here is the context around the problem:
{
"id": 1,
"name": "foo"
},
{
"id": 2,
"name": "bar"
}
On the server side, you have the following code snippet:
app.post('/', function(request, response) {
console.log("Got response: " + response.statusCode);
response.on('data', function(chunk) {
queryResponse += chunk;
console.log('data');
});
response.on('end', function() {
console.log('end');
});
});
Now let's address the issue at hand. 🎯 It seems that you are trying to consume the POST data incorrectly. The events 'data' and 'end' that you are listening to on the response
object are only applicable when you are consuming data from the server's response, not the client's request. Let's fix that! 💡
To consume JSON POST data in an Express application, you need to follow these steps:
Install the necessary dependencies: Express and Body-parser.
Import the required modules into your application.
Configure Express to use the Body-parser middleware.
Access the JSON POST data in your Express route handler.
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
// Configure Body-parser middleware
app.use(bodyParser.json());
app.post('/', function(request, response) {
const jsonData = request.body;
console.log(jsonData);
// Do something with the JSON data
response.sendStatus(200); // Send a response back to the client
});
app.listen(3000, function() {
console.log('Server is running on port 3000.');
});
With the updated code, you can now access the JSON POST data using request.body
. No need to listen to 'data' and 'end' events anymore. 🙌
🔥 Here's your call-to-action: if you found this guide helpful or have any further questions, let us know in the comments below! We love engaging with our readers and helping them resolve their coding challenges. Plus, don't forget to share this post with your friends who might find it useful. Sharing is caring! 🤗
That's all for now! We hope this guide has made consuming JSON POST data in an Express application a breeze for you. Happy coding! 💻🎉