How can I pretty-print JSON using Go?
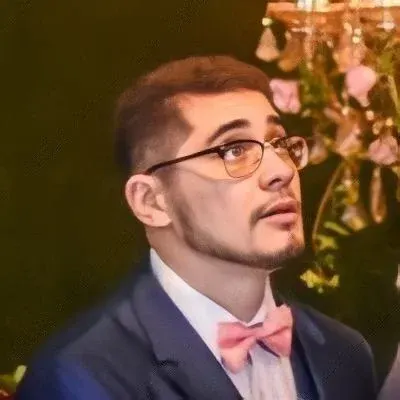
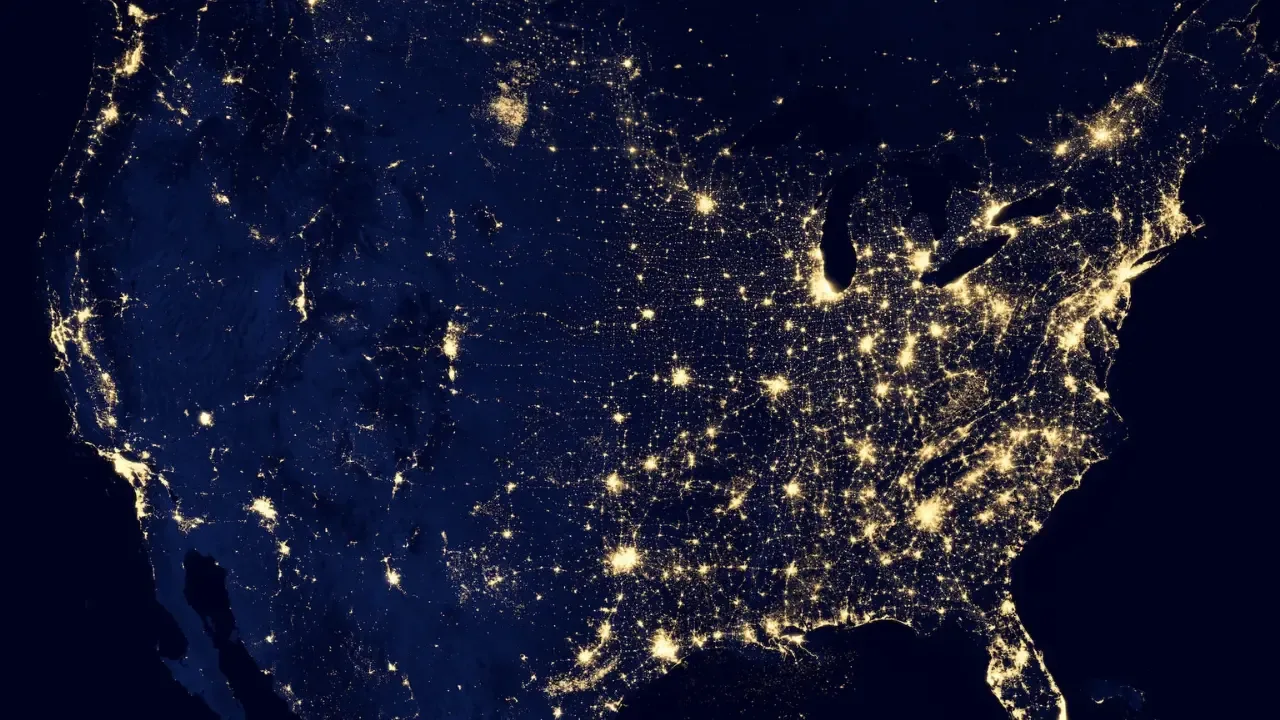
Pretty-Print JSON Using Go Like a Boss! 🌟
Have you ever found yourself staring at a wall of unreadable JSON code? 🤔 Don't worry, you're not alone. We've all been there, squinting our eyes and desperately trying to make sense of it all. But fear not, my fellow Go enthusiasts! I'm here to show you how to pretty-print JSON using Go with ease and style! 💪🏼
The Problem: Unreadable JSON 😖
We've all used json.Marshal
to convert our Go structs to JSON strings. However, the default output tends to be a single line of compact and unreadable JSON. This can be problematic when we need to debug or simply understand the structure of our data.
The Solution: Enter the encoding/json
Package! 🚀
The encoding/json
package in Go provides us with the tools we need to prettify our JSON output. Let's dive right in with some easy solutions to your JSON formatting troubles!
Solution #1: MarshalIndent 🌈
The MarshalIndent
function from the encoding/json
package is your best friend when it comes to pretty-printing JSON. It indents the JSON output using spaces or tabs, making it easier to read and understand.
import (
"encoding/json"
"fmt"
)
func main() {
// Your existing JSON string or Go struct
jsonData := `{"name":"John","age":30,"city":"New York"}`
// Pretty-print the JSON string
var prettyJSON bytes.Buffer
json.Indent(&prettyJSON, []byte(jsonData), "", " ")
fmt.Println(prettyJSON.String())
// Alternatively, you can also print the JSON to a file
// ioutil.WriteFile("pretty.json", prettyJSON.Bytes(), 0644)
}
In this example, we create a JSON string named jsonData
. Then, we use the MarshalIndent
function to prettify the JSON and store the output in a bytes.Buffer
called prettyJSON
. Finally, we print the pretty JSON using fmt.Println
.
Solution #2: Use gojsonq
Package 🌟
If you're looking for a more advanced way of working with JSON in Go, the gojsonq
package provides a powerful and intuitive query builder for JSON. It allows you to retrieve and manipulate JSON data using a simple and expressive syntax.
Here's a quick example of how to use gojsonq
to prettify your JSON:
import (
"fmt"
"github.com/thedevsaddam/gojsonq/v2"
)
func main() {
// Your existing JSON string or Go struct
jsonData := `{"name":"John","age":30,"city":"New York"}`
// Pretty-print the JSON string
prettyJSON, _ := gojsonq.Pretty(jsonData)
fmt.Println(prettyJSON)
}
In this example, we import the github.com/thedevsaddam/gojsonq/v2
package and use the Pretty
function to prettify the JSON and store the output in a variable called prettyJSON
. Finally, we print the pretty JSON using fmt.Println
.
Solution #3: Custom Pretty-Print Package 🎨
If you prefer a custom solution, you can create your own pretty-print package using the built-in encoding/json
package in Go. This gives you complete control over the formatting style and allows you to add your own personal touch!
For a detailed step-by-step guide on how to build your custom pretty-print package, check out our GitHub repository for an example implementation.
Your Turn to Shine! ✨
Now that you have a few options for pretty-printing JSON in Go, it's time to give it a try! Choose the method that suits your needs best and start making your JSON code shine!
Did you find this guide helpful? Do you have any other tips or tricks for pretty-printing JSON in Go? Share your thoughts and suggestions in the comments below. Let's make JSON formatting more accessible and enjoyable for everyone! 🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
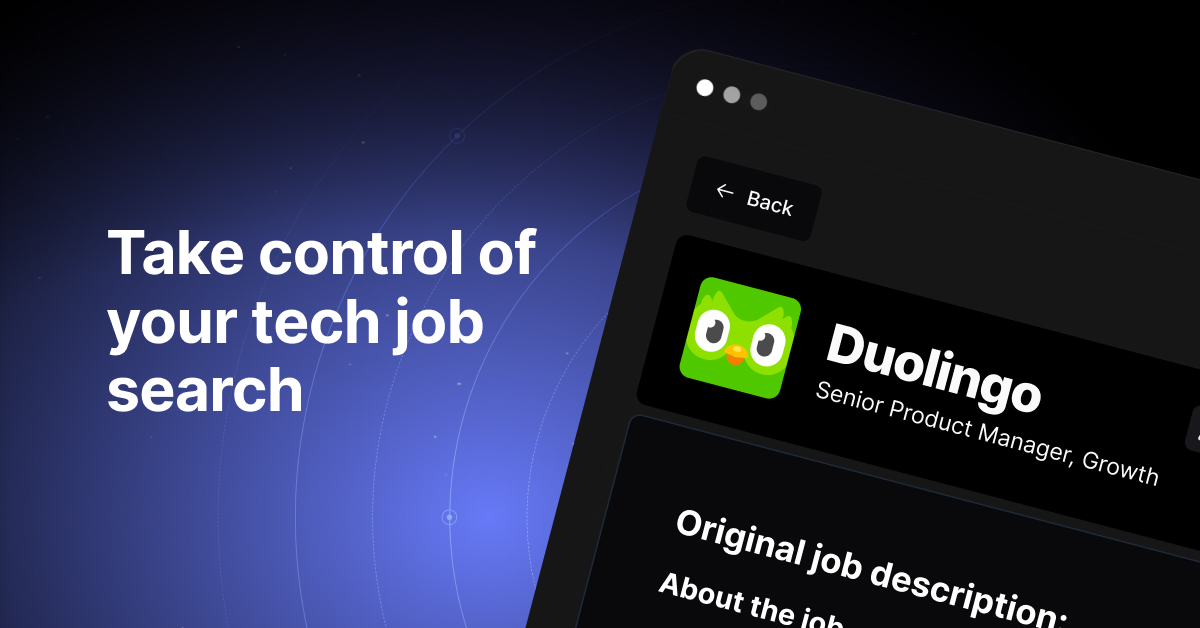