Handling JSON Post Request in Go
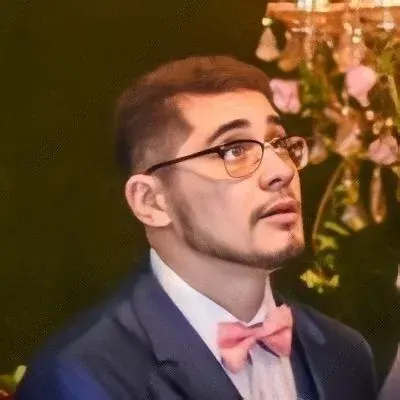
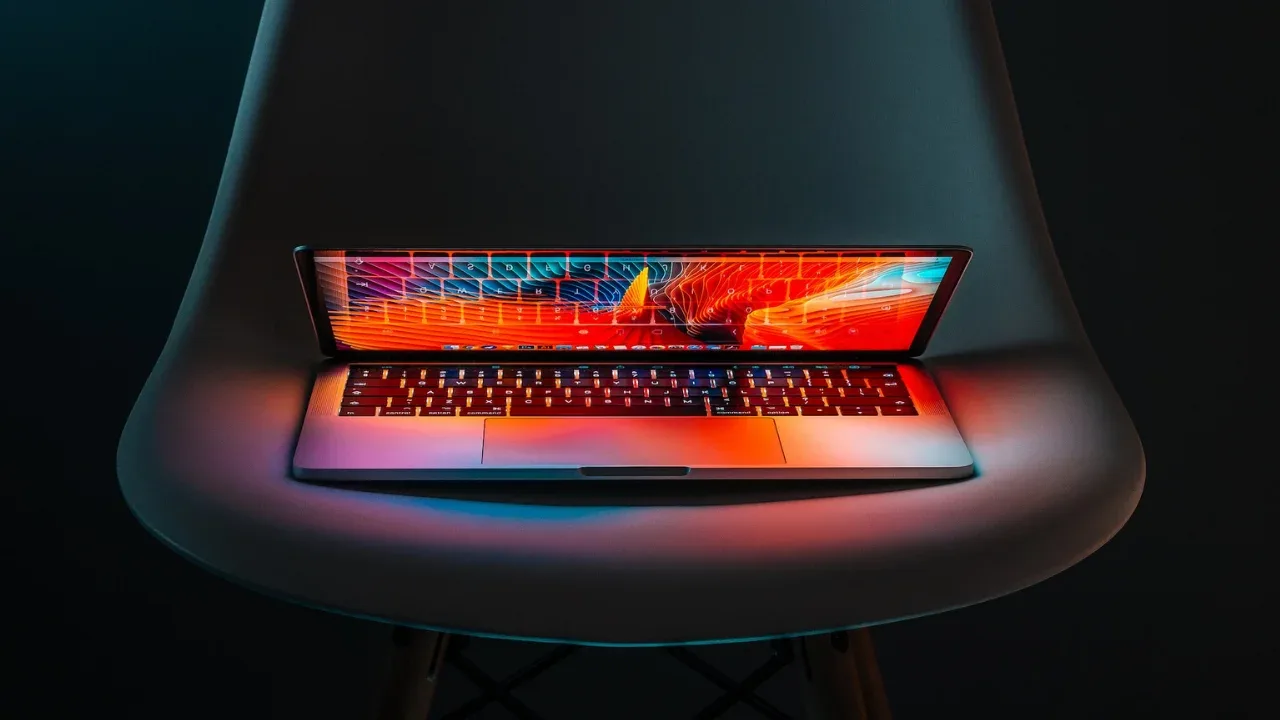
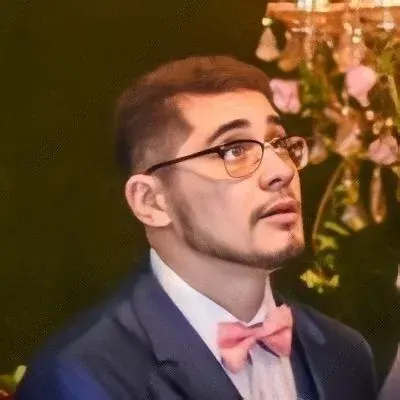
Handling JSON POST Request in Go: A Simplified Guide 📝💡
So you're working with Go, and you've encountered a situation where you need to handle a POST request with JSON data. You're not alone! Many developers have faced this challenge and struggled to find a clean and efficient solution. But fear not, my fellow Gopher! In this guide, we'll explore this problem, discuss common issues, and provide easy-to-implement solutions. Let's jump right in! 💪🐹
The Challenge: Handling a POST Request with JSON Data 😱📥
Go has a reputation for well-designed libraries, but when it comes to handling POST requests with JSON data, many developers find themselves scratching their heads. Most examples and tutorials focus on form data, leaving us with outdated or hacky solutions. But we won't settle for that! We'll find the best practice together! 🤝✨
Understanding the Problem: The Example 🕵️♂️🔍
To better understand the problem, let's examine an example shared by one of our fellow developers:
package main
import (
"encoding/json"
"log"
"net/http"
)
type test_struct struct {
Test string
}
func test(rw http.ResponseWriter, req *http.Request) {
req.ParseForm()
log.Println(req.Form)
//LOG: map[{"test": "that"}:[]]
var t test_struct
for key, _ := range req.Form {
log.Println(key)
//LOG: {"test": "that"}
err := json.Unmarshal([]byte(key), &t)
if err != nil {
log.Println(err.Error())
}
}
log.Println(t.Test)
//LOG: that
}
func main() {
http.HandleFunc("/test", test)
log.Fatal(http.ListenAndServe(":8082", nil))
}
In this example, a test_struct
type is defined, and the test
function is responsible for handling the /test
endpoint. However, the code seems convoluted and relies on parsing the form data, resulting in unexpected behavior. We can do better than this! 🙌
The Solution: Simplifying JSON Post Request Handling in Go 🚀🔧
To handle JSON POST requests in Go, we need to make a few changes. Let's break it down step-by-step:
Remove the
req.ParseForm()
line: Parsing the form data is unnecessary and unrelated to our JSON request. We can safely remove it to simplify our code and avoid any conflicts.Decode the JSON request directly: Go provides a convenient way to decode JSON data using the
json
package. We'll leverage this to parse and decode our request.Implement error handling: JSON decoding can fail due to various reasons such as invalid syntax or incompatible types. It's important to handle these error conditions gracefully to avoid crashes and unexpected behavior.
Applying these changes, our code can be refined as follows:
package main
import (
"encoding/json"
"log"
"net/http"
)
type test struct {
Test string `json:"test"`
}
func handleTest(rw http.ResponseWriter, req *http.Request) {
var t test
err := json.NewDecoder(req.Body).Decode(&t)
if err != nil {
http.Error(rw, err.Error(), http.StatusBadRequest)
return
}
log.Println(t.Test)
// LOG: that
}
func main() {
http.HandleFunc("/test", handleTest)
log.Fatal(http.ListenAndServe(":8082", nil))
}
Voila! 🎉 We've simplified our code and improved the approach to handle JSON POST requests in Go. By using json.NewDecoder
and Decode
, we can directly parse the JSON request and decode it into our desired struct. Error handling is also included, ensuring a graceful response in case of any issues.
Time to Celebrate: Engage and Share Your Thoughts! 🎉📣
Now that we've explored and solved the problem of handling JSON POST requests in Go, it's your turn to Put your newfound knowledge to use! Implement it in your projects, experiment, and share your thoughts with the community. Let's encourage a conversation and help fellow Gophers overcome this challenge! 💬🧠
Have you encountered any other obstacles in Go development? Are there any topics you'd like us to cover in our next blog post? Let us know in the comments below! Your engagement and feedback are vital to building a thriving community of developers.
Until then, happy coding! Keep rocking the Go world, one JSON request at a time! 🚀💻
(Go is also known as Golang to the search engines, and mentioned here so others can find it.)