Display curl output in readable JSON format in Unix shell script
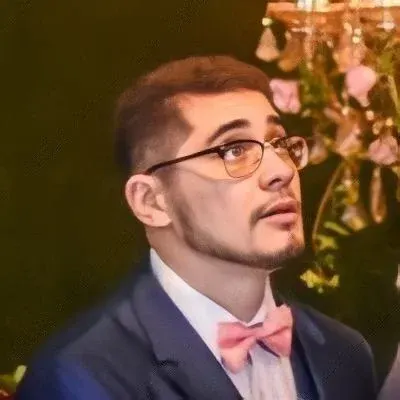
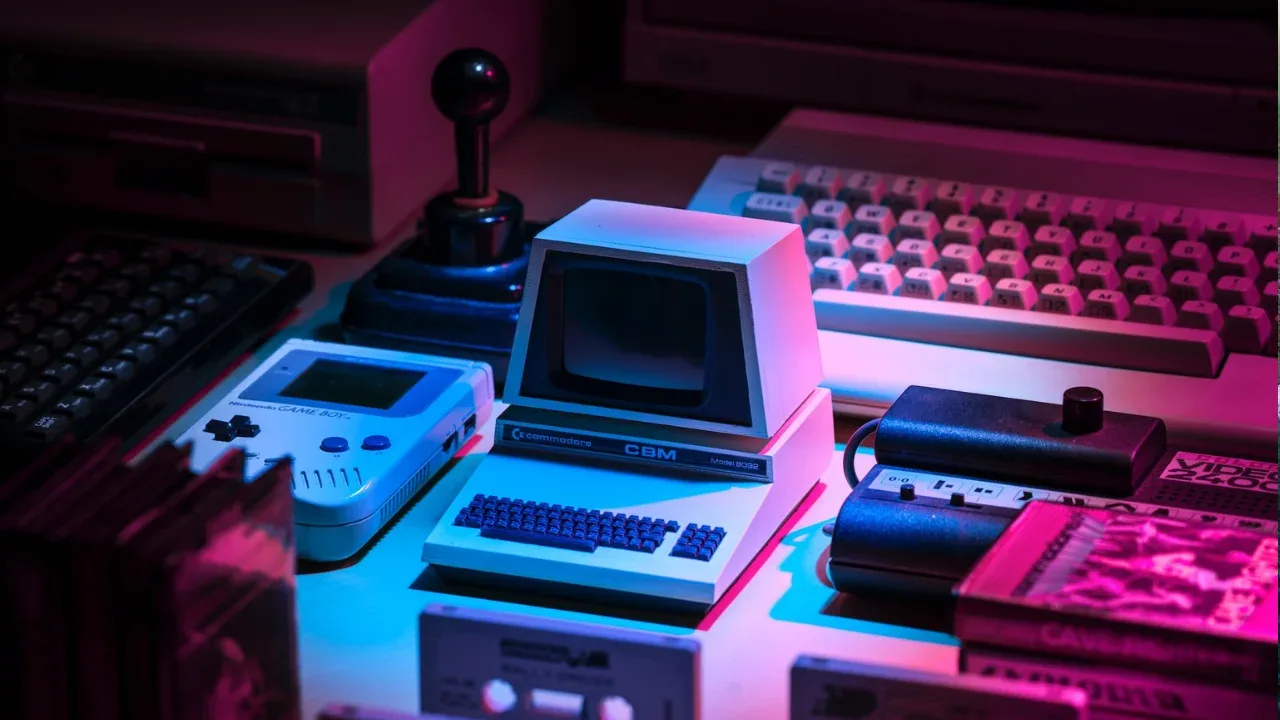
Formatting curl output to readable JSON format in Unix shell script
So, you're building a Unix shell script and want to display the output of a curl command in a readable JSON format? No worries, I've got you covered! 🚀
The Problem: Unreadable JSON output
By default, when you execute a curl command and redirect the output to a file, the JSON data may appear as a single long line, making it difficult to read and understand. In your case, the output looks something like this:
{"type":"Show","id":"123","title":"name","description":"Funny","channelTitle":"ifood.tv","lastUpdateTimestamp":"2014-04-20T20:34:59","numOfVideos":"15"}
The Solution: Formatting JSON with jq
To achieve the desired readable JSON format, we will use a command-line tool called jq. Jq is a lightweight and flexible JSON processor that enables us to manipulate, filter, and format JSON data effortlessly.
Step 1: Installation
Before we proceed, let's ensure that jq is installed on your system. Open your terminal and type the following command to check if jq is installed:
jq --version
If you see the version number, great! You already have jq installed. Otherwise, you can install it using package managers like apt
, homebrew
, or by downloading the binary from the official website.
Step 2: Formatting the JSON output
Now that we have jq installed let's dive into the actual formatting process. In your shell script, assuming you have the JSON data stored in a variable called $jsonOutput
, you can pipe it to jq using the echo
command and the -r
flag to produce the formatted output.
Here's an example command to format your JSON output:
echo "$jsonOutput" | jq '.' > formatted_output.json
In the above command, we use the .
operator in jq to represent the top-level object. By passing $jsonOutput
as input to jq, it formats the JSON with proper indentation, making it human-readable. The formatted output is then stored in a file called formatted_output.json
.
Take it further: Pretty-printing JSON in terminal
But what if you don't want to save the formatted output in a file? Suppose you want to directly print the pretty-printed JSON on your terminal. Well, guess what? jq can do that too! 🎉
You can modify the last step of the previous command to achieve this:
echo "$jsonOutput" | jq '.'
Now, when you run your shell script, the formatted JSON output will be displayed directly in your terminal.
Putting it all together
Here's the complete shell script code snippet to format your JSON output using jq:
#!/bin/bash
jsonOutput=$(curl some_api_endpoint)
formattedOutput=$(echo "$jsonOutput" | jq '.')
echo "$formattedOutput"
Save the code snippet into a shell script file, make it executable, and run it. You should see your JSON output beautifully formatted on your screen!
Conclusion and Call-to-Action
That's it! You've learned how to format the output of a curl command into a readable JSON format using jq in Unix shell scripts. Now you can easily understand and work with JSON data in your scripts.
Try implementing this solution in your own script and let me know in the comments below if you faced any issues or if it worked like a charm! Feel free to share any other handy tips or tricks you use for working with JSON in shell scripts. Happy coding! 🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
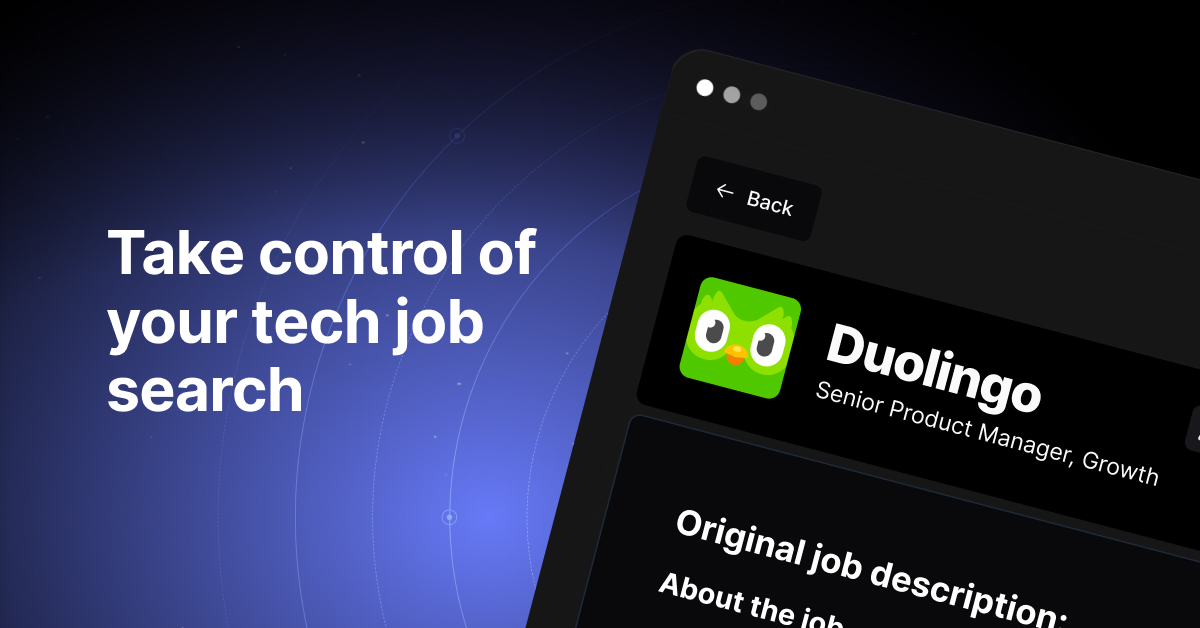