Decoding JSON using json.Unmarshal vs json.NewDecoder.Decode
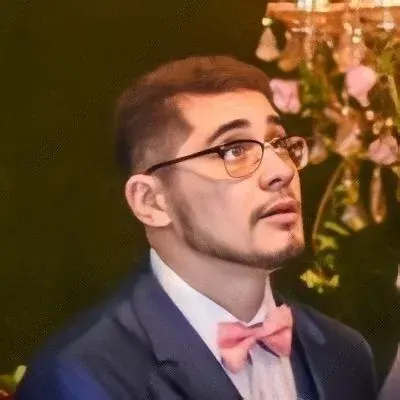
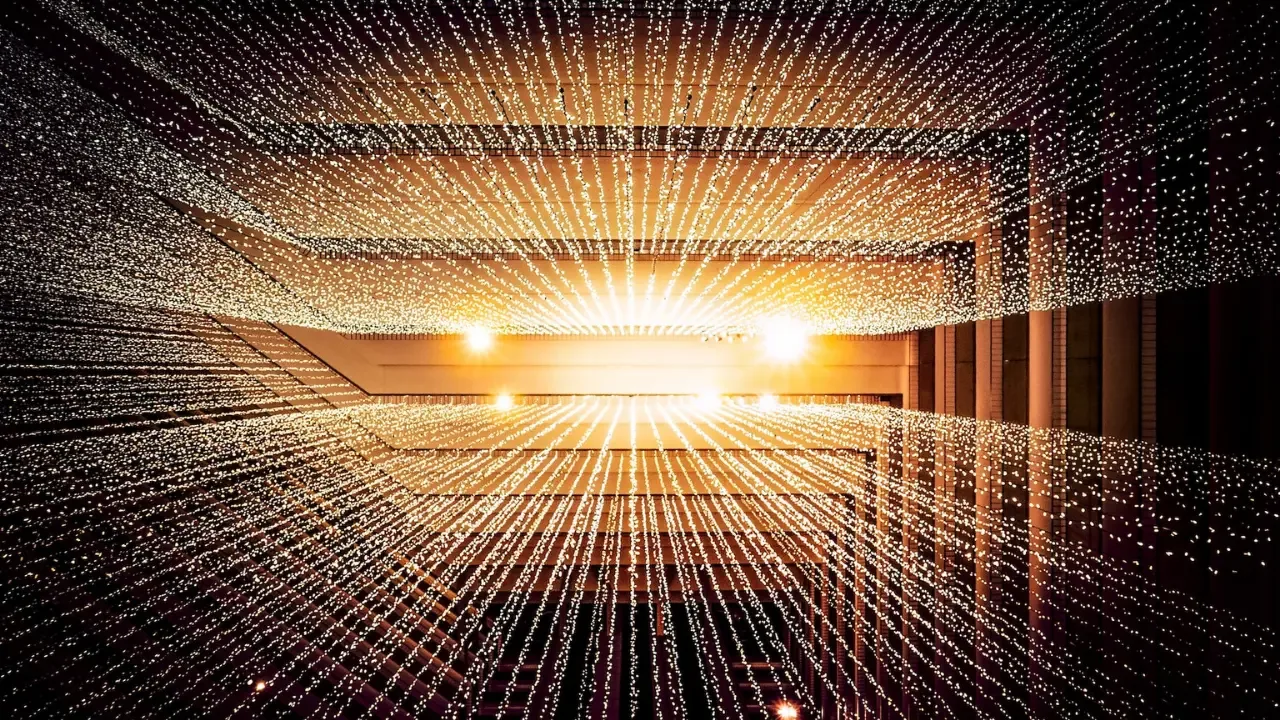
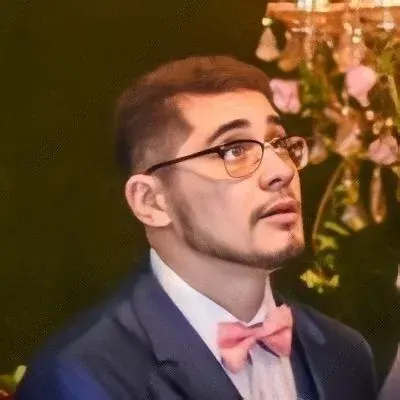
Decoding JSON using json.Unmarshal
vs json.NewDecoder.Decode
š Hey there! Are you developing an API client and struggling with encoding and decoding JSON payloads? Don't worry, I've got you covered! In this blog post, we'll explore two popular methods for decoding JSON in Go: json.Unmarshal
and json.NewDecoder.Decode
. We'll address common issues, provide easy solutions, and help you choose the best approach for your API client. š
The Two Possibilities
When it comes to decoding JSON in Go, you generally have two options. Let's take a closer look at both methods:
1. Using json.Unmarshal
With json.Unmarshal
, you can pass the entire response string to decode the JSON payload. Here's an example:
data, err := ioutil.ReadAll(resp.Body)
if err == nil && data != nil {
err = json.Unmarshal(data, value)
}
2. Using json.NewDecoder.Decode
Alternatively, you can utilize json.NewDecoder.Decode
, which is specifically designed for decoding readers. Here's an example:
err = json.NewDecoder(resp.Body).Decode(value)
Both methods work perfectly fine, but your choice may depend on the specific circumstances of your use case. š¤
Preferences and Recommendations
Considering the HTTP responses that implement io.Reader
, the second method (json.NewDecoder.Decode
) seems to require less code in your scenario. However, you might still wonder if there is a preference for one solution over the other.
While both options are valid, the accepted answer from this Stack Overflow question recommends using json.Decoder
instead of json.Unmarshal
. However, it doesn't explicitly mention the reason behind the recommendation.
Why json.Decoder
Should Be Your Preferred Choice
Although the Stack Overflow answer doesn't explain the rationale, there are a few advantages to using json.Decoder
over json.Unmarshal
. Here's why we recommend using json.Decoder
:
Efficiency: The
json.Decoder
approach is more memory-efficient since it reads and decodes JSON incrementally from the reader, rather than reading the entire response into memory.Streaming Support:
json.Decoder
enables you to handle large, continuous streams of JSON data, which can be incredibly useful when processing live updates or real-time data.Flexibility:
json.Decoder
provides more fine-grained control over the decoding process, allowing you to handle various scenarios such as parsing specific JSON elements or skipping unwanted fields.
Considering these advantages, we strongly recommend using json.Decoder
for decoding JSON in your API client.
Wrapping Up
Decoding JSON can be a breeze if you know which approach to use. In this blog post, we discussed the differences between json.Unmarshal
and json.NewDecoder.Decode
and why we prefer the latter. By utilizing json.Decoder
, you'll benefit from improved efficiency, streaming support, and flexibility.
So go ahead and level up your API client by implementing json.Decoder
! If you have any questions or suggestions, feel free to leave a comment below. Happy coding! šš©āš»šØāš»