Converting Go struct to JSON
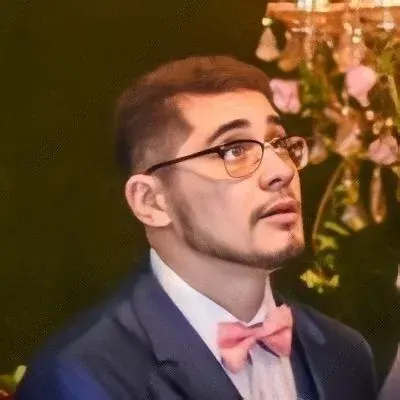
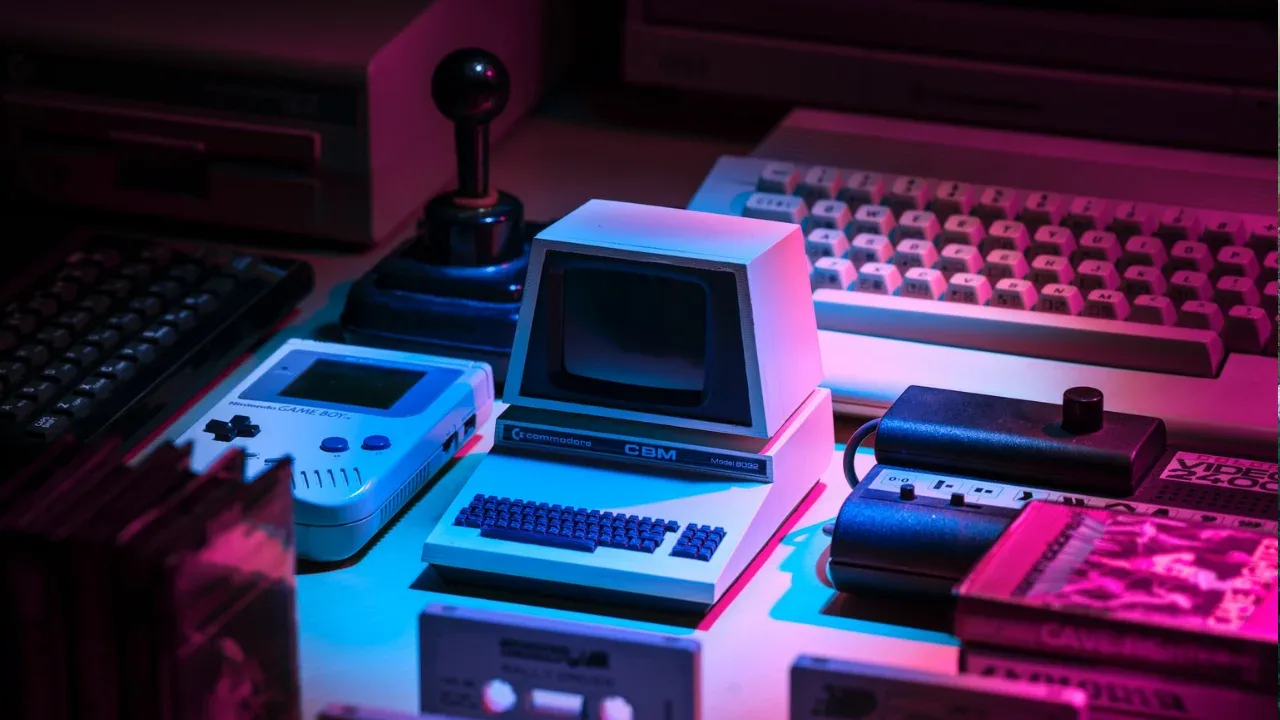
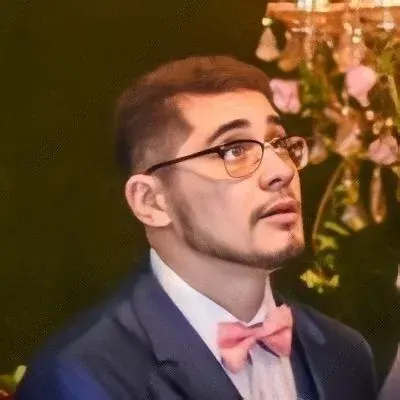
Converting Go struct to JSON: A Simple Guide 🐳
Are you facing the frustrating issue of your Go struct being converted to an empty JSON object? 😫 Don't worry, we've got you covered! In this blog post, we will explore this common problem and provide you with easy solutions to resolve it.
Let's start by examining the code snippet you provided:
package main
import (
"fmt"
"encoding/json"
)
type User struct {
name string
}
func main() {
user := &User{name: "Frank"}
b, err := json.Marshal(user)
if err != nil {
fmt.Printf("Error: %s", err)
return
}
fmt.Println(string(b))
}
When running this code, the output you receive is {}
– an empty JSON object. But why does this happen? 🤔
The Problem: Unexported Fields in Go Structs 😨
In Go, when you define a struct field with a lowercase letter, it becomes unexported and is not visible outside the package. The json.Marshal
function uses reflection to convert exported fields to JSON, but it cannot access unexported fields. Therefore, the resulting JSON will be empty.
In our example, the name
field of the User
struct is lowercase, making it unexported. As a result, it is not included in the JSON output.
Solution 1: Export Struct Fields 🌟
To resolve this issue, we need to export the struct field by capitalizing its first letter. Let's modify our code to achieve this:
type User struct {
Name string // Exported field
}
By changing name
to Name
, we export the field and allow it to be marshaled to JSON successfully. Now, when you run the code, you should see the expected JSON output: {"Name":"Frank"}
.
Solution 2: Use Struct Tags 🏷️
Sometimes, you might not be able to modify the struct fields directly, especially when working with third-party libraries or existing codebases. In such cases, you can use struct tags to customize how json.Marshal
handles the field.
Let's update our code to use a struct tag:
type User struct {
name string `json:"name"` // Struct tag
}
By adding the json:"name"
tag to the name
field, we instruct json.Marshal
to use the tag value as the key in the JSON output. Running the code will now produce the desired JSON: {"name":"Frank"}
.
🎉 Show Us What You've Done!
Congratulations on resolving the Go struct to JSON conversion issue! We hope that this guide has helped you understand and overcome this common problem. Remember, the exportation of fields and struct tags can make a significant difference when it comes to marshaling structs into JSON.
Now it's your turn! Feel free to experiment with different struct fields and struct tags. Got any cool code snippets or insights? Share them with us in the comments section below! Let's learn from each other and continue exploring the vast world of Go development! 🐹🔥
Disclaimer: The code samples in this blog post are simplified for better understanding. Always make sure to handle errors appropriately and follow best practices when implementing in a production environment.