Convert Dictionary to JSON in Swift
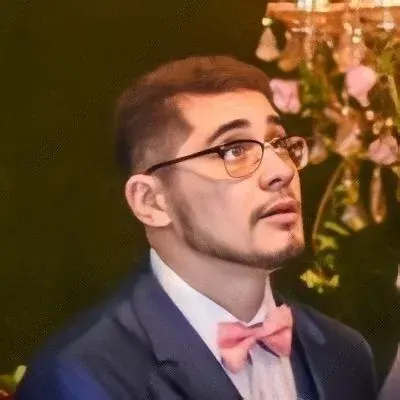
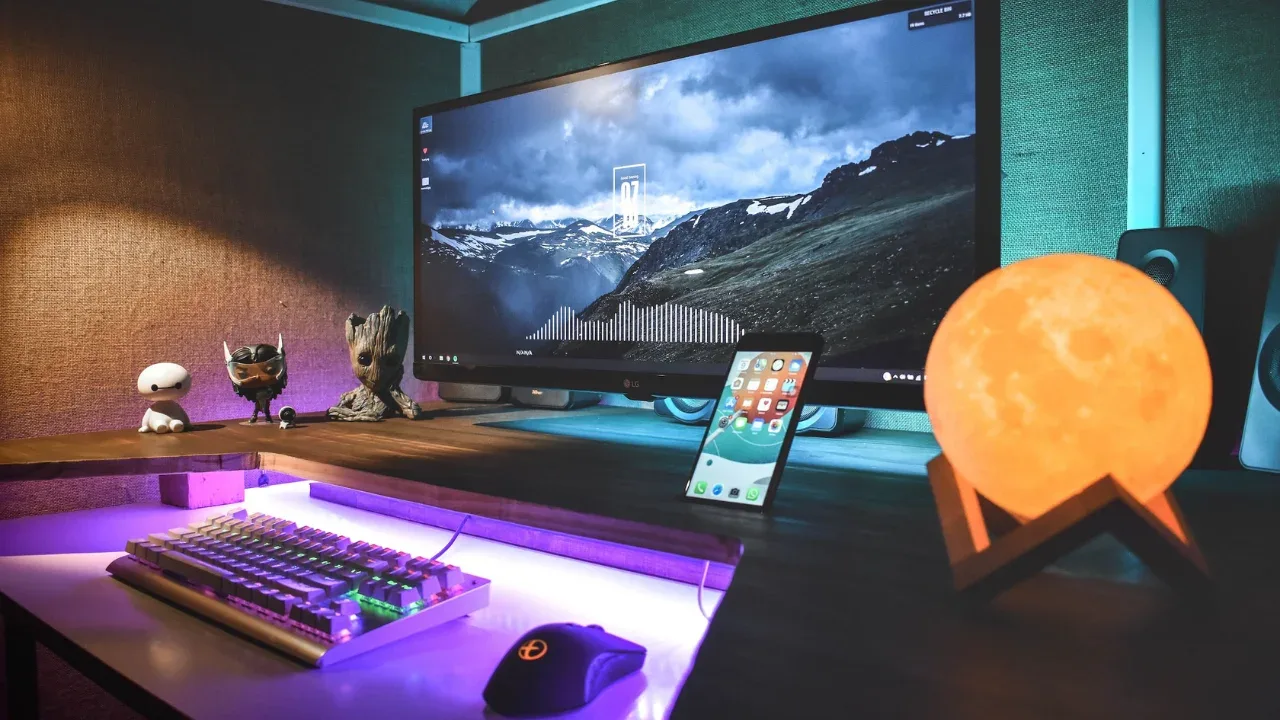
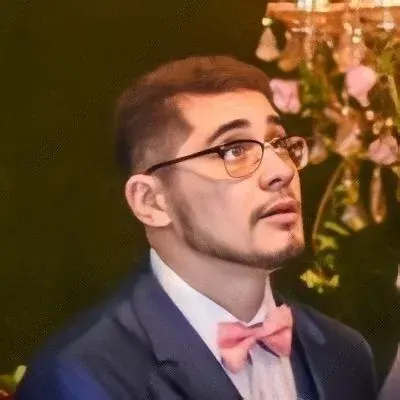
Converting Dictionary to JSON in Swift: A Simple Guide 📝🔀🔢
So, you have a dictionary in Swift, and now you want to convert it to JSON. Don't worry, we've got you covered! In this guide, we'll walk you through the process step by step, addressing common issues and providing easy solutions. By the end, you'll be able to convert your dictionary to JSON like a pro! Let's get started! 🚀
Understanding the Problem 🔍
Before we dive into the solution, let's take a moment to understand the problem at hand. You mentioned that you have created a dictionary in Swift and want to convert it to JSON. The dictionary looks something like this:
var postJSON = [ids[0]:answersArray[0], ids[1]:answersArray[1], ids[2]:answersArray[2]] as Dictionary
print(postJSON)
// Output: [2: B, 1: A, 3: C]
Your goal is to convert this dictionary to a JSON format. Great! Now, let's explore the solutions.
Solution: Using JSONSerialization 🧩
Swift provides a handy built-in class called JSONSerialization
, which makes converting between Swift objects and JSON a breeze. Here's how you can use it to convert your dictionary:
do {
let jsonData = try JSONSerialization.data(withJSONObject: postJSON, options: [])
if let jsonString = String(data: jsonData, encoding: .utf8) {
print(jsonString)
// Output: {"2":"B","1":"A","3":"C"}
}
} catch {
print("Error converting dictionary to JSON: \(error)")
}
Here's what's happening in the above code:
We use
JSONSerialization.data(withJSONObject:options:)
to convert the dictionarypostJSON
toData
representing JSON.We then convert this
Data
to a string usingString(data:encoding:)
. The.utf8
encoding ensures the string is in UTF-8 format, which is the standard for JSON.Finally, we print out the resulting JSON string.
And voila! 🎉 You have successfully converted your dictionary to JSON using the power of JSONSerialization
!
Wrapping It Up with a Call-to-Action 🎯
Converting a dictionary to JSON in Swift doesn't have to be a daunting task. By leveraging the capabilities of JSONSerialization
, you can achieve this in just a few lines of code. Now that you have the knowledge, why not try it out yourself?
Give it a go and let us know how it worked for you! If you have any questions or other cool Swift-related topics you'd like us to cover, feel free to leave a comment below. Happy coding! 💻💡