Convert a Map<String, String> to a POJO
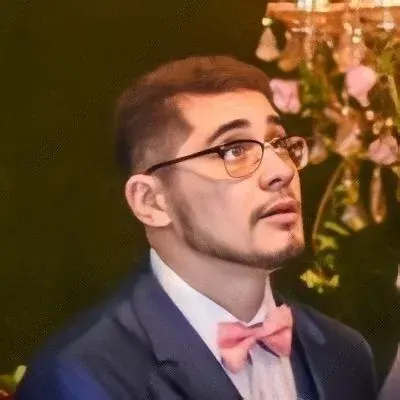
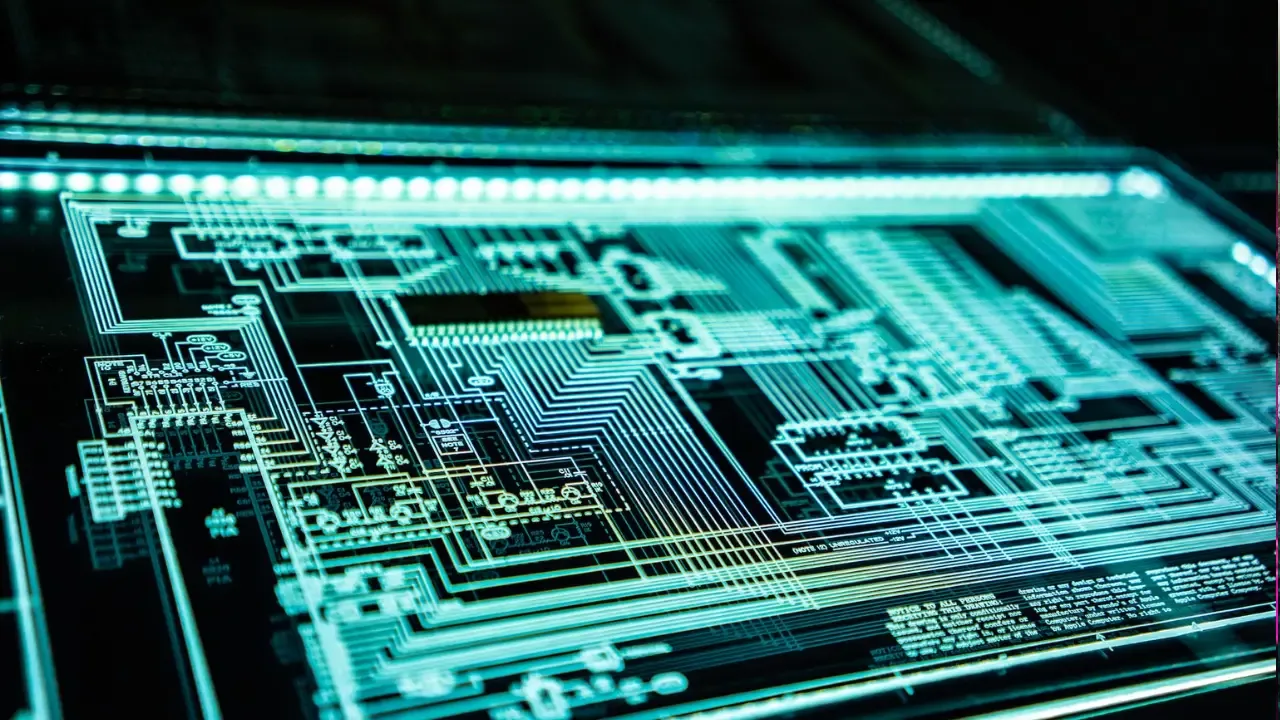
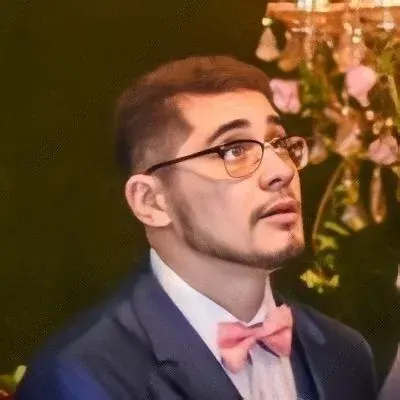
πΊοΈ Converting a Map<String, String> to a POJO: A Hassle-free Guide π
Are you tired of tedious steps to convert a Map<String, String>
to a POJO? Look no further! In this guide, we will explore common issues and provide you with easy solutions to convert a Map directly to a POJO, without the need for intermediate JSON conversions. Don't waste another minute, let's dive right in! πͺ
The Map to JSON Dilemma πΊοΈ β‘οΈ π¦
Many developers find themselves in a tough spot when trying to convert a Map to a POJO. The commonly-suggested approach involves converting the Map to JSON and then mapping it back to the POJO using libraries like Jackson or Gson. However, this feels like a roundabout way of achieving our goal! π
A Solution That Serves Pure POJO Realness π
Turns out, there is a simpler solution that directly converts a Map to a POJO. π Let's take a look at a code snippet that demonstrates this approach using Jackson:
import com.fasterxml.jackson.databind.ObjectMapper;
public class MapToPojoConverter {
public static void main(String[] args) {
// Create a sample Map
Map<String, String> sampleMap = new HashMap<>();
sampleMap.put("name", "John Doe");
sampleMap.put("age", "28");
sampleMap.put("occupation", "Developer");
// Convert the Map to a POJO
ObjectMapper objectMapper = new ObjectMapper();
YourPojo pojo = objectMapper.convertValue(sampleMap, YourPojo.class);
// Voila! The Map is now a POJO!
}
}
By using the convertValue
method from Jackson's ObjectMapper
class, we can seamlessly convert our Map to a POJO. π
Putting the Magic in Action β¨
Let's break down the code snippet and understand how it works:
We create a sample Map using the
HashMap
class and populate it with key-value pairs.Then, we create an instance of
ObjectMapper
, which is responsible for the conversion magic.Finally, we call the
convertValue
method, passing in the Map and the desired POJO class type.
And just like that, our Map is transformed into a full-fledged POJO, ready to take on the world! π
Engage With Us! π£
You've learned a nifty trick to convert a Map to a POJO without any hassle. Why stop here? We have a ton of exciting content and useful tips waiting for you on our blog. Join our community, share your thoughts, and level up your tech game! π
Got questions? Drop them in the comments section below. Our experts are here to assist you and provide further insights. Let's solve problems together! π€
So go ahead, give this technique a try, and let us know how it works for you. Remember, embracing simplicity can lead to incredible breakthroughs! π
Happy coding! π©βπ»π¨βπ»