Sending multipart/formdata with jQuery.ajax
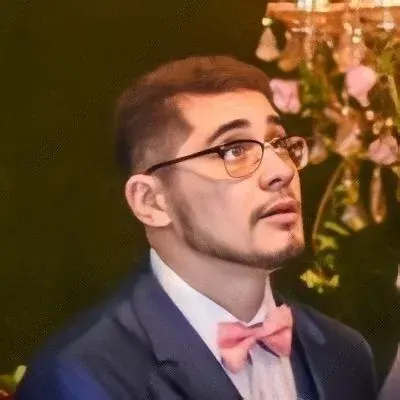
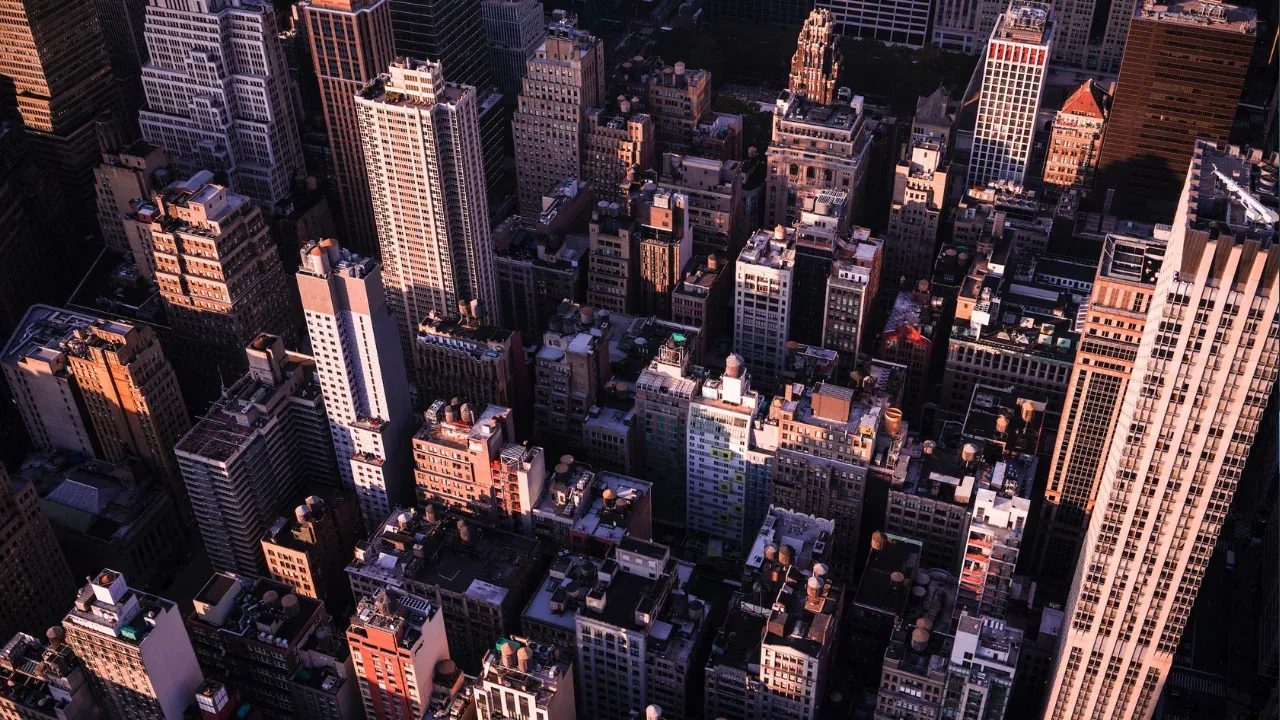
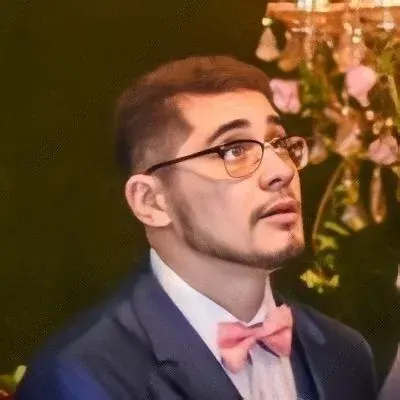
📄 Sending multipart/formdata with jQuery.ajax: A Simple Guide
Are you struggling with sending a file to a server-side PHP script using jQuery's ajax function? Don't worry, we've got you covered! In this guide, we'll address common issues and provide easy solutions to help you overcome this challenge. So, let's dive right in! 💪
The Problem: Sending Data to the Server
You mentioned that you are able to retrieve the file list using $('#fileinput').attr('files')
. However, you are facing difficulties in sending this data to the server. When you check the resulting array $_POST
on the server-side PHP script, it returns 0 or NULL
. This issue can be frustrating, but we're here to help you fix it.
The Solution: Configuring jQuery.ajax
To correctly send the file data to the server, you need to configure your jQuery.ajax call by adjusting a few parameters.
$.ajax({
url: 'php/upload.php',
data: new FormData($('#file')[0]), // Use FormData to handle file input data
cache: false,
contentType: false, // Remove the content type
processData: false, // Avoid processing the data
type: 'POST',
success: function(data){
alert(data);
}
});
Step-by-Step Explanation
URL: Specify the URL of the server-side PHP script (e.g.,
'php/upload.php'
).Data: Instead of directly passing
$('#file').attr('files')
, we usenew FormData($('#file')[0])
. This ensures that the file data is properly serialized and sent to the server.Cache: Set
cache
tofalse
to prevent caching issues.Content Type: Remove the
contentType
parameter or set it tofalse
. This allows the browser to automatically set the correctContent-Type
header, including the necessary multipart form data boundary.Process Data: Set
processData
tofalse
to prevent jQuery from processing the data. This is crucial becauseFormData
takes care of serializing the file data for you.Type: Specify the HTTP method type (e.g.,
'POST'
).Success Callback: In the
success
callback function, you can handle the server's response (e.g., displaying it in an alert).
The Call to Action: Share your Experience!
We hope this guide has helped you solve the issue of sending multipart/formdata with jQuery.ajax. If you found it useful, don't keep it to yourself! Share this post with your friends and colleagues who might also benefit from it. 💡
Have you encountered any other web development challenges you'd like us to address? Feel free to leave a comment below or reach out to us. We'd love to hear from you and continue providing helpful content that solves your real-world problems.
Keep coding! 🚀