Selecting element by data attribute with jQuery
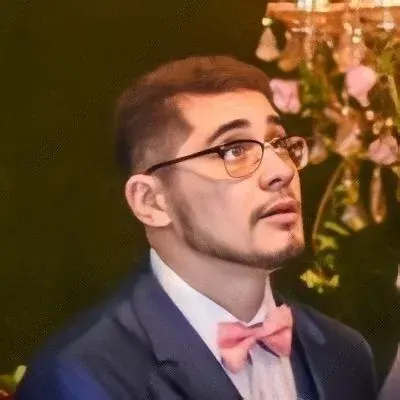
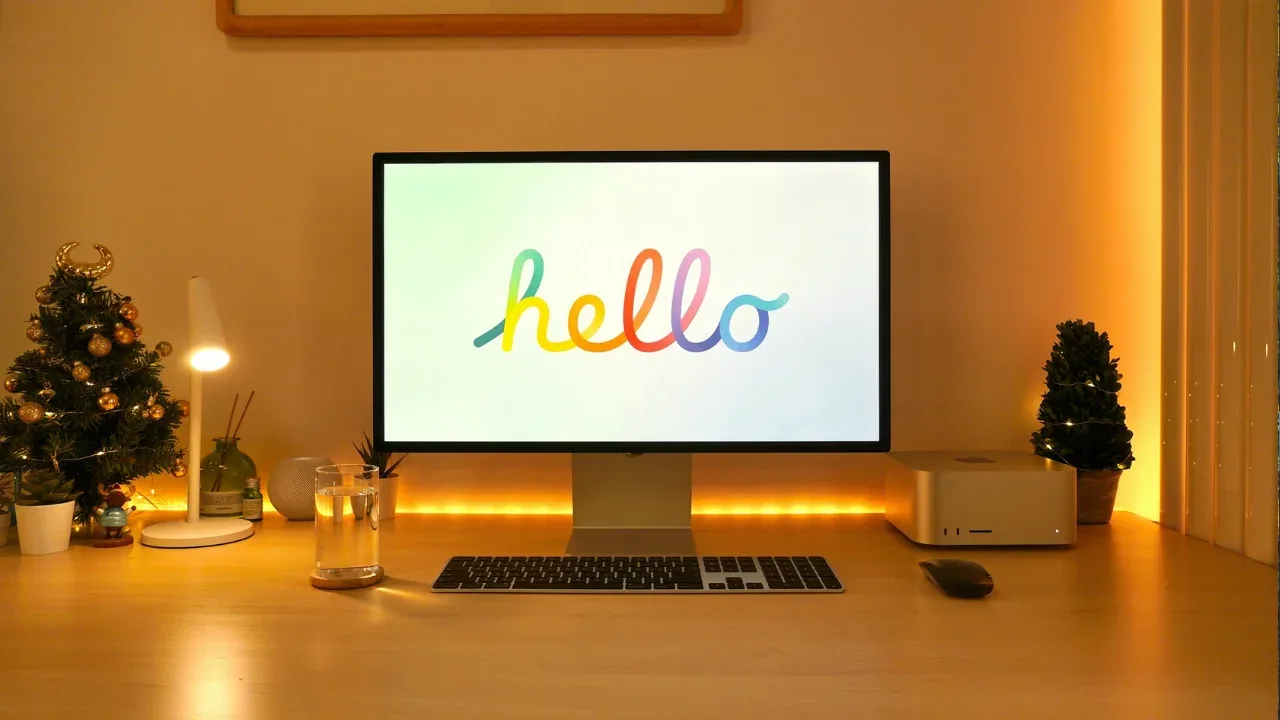
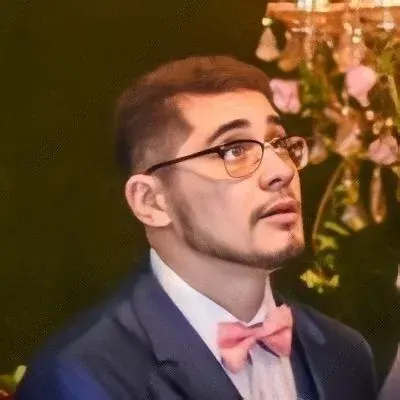
đ Selecting Elements by Data Attribute with jQuery: A Quick and Easy Guide đšī¸
Hey there, tech enthusiasts! đ Today, we're diving into the fascinating world of jQuery and exploring how to select elements based on their data attributes. đ¯ If you've ever found yourself struggling to retrieve elements with specific data values attached to them, worry not! We've got you covered with some simple and effective solutions.
So, let's get started by addressing the common issue presented in our introductory question:
đ The Problem: How can we easily select elements based on their data attributes?
<p>Is there an easy and straight-forward method to select elements based on their <code>data</code> attribute? For example, select all anchors that have a data attribute named <code>customerID</code> with a value of <code>22</code>.</p>
<p>I am kind of hesitant to use <code>rel</code> or other attributes to store such information, but I find it much harder to select an element based on what data is stored in it.</p>
đĄ The Solution: jQuery to the rescue! With jQuery's powerful selector syntax, we can easily target elements based on their data attributes. Here's how you can do it:
1ī¸âŖ Selecting Elements by Data Attribute Name To select all anchors with a data attribute named "customerID," simply use the "$" selector along with the attribute name enclosed in square brackets.
$('a[data-customerID]');
This will return all anchors that have the specified data attribute, regardless of its value.
2ī¸âŖ Filtering Elements by Data Attribute Value To narrow down the selection further and target only anchors with a data attribute named "customerID" and a value of "22," you can use the "$" selector combined with attribute name-value notation.
$('a[data-customerID="22"]');
By specifying the exact value within double quotes, the selector will return only the desired anchors.
đĻ An Example: Let's put everything into perspective with a quick code snippet:
<!DOCTYPE html>
<html>
<head>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function() {
// Select all anchors with a data attribute named "customerID"
var allCustomerAnchors = $('a[data-customerID]');
console.log(allCustomerAnchors);
// Filter and select anchors with data attribute named "customerID" and value of "22"
var specificCustomerAnchors = $('a[data-customerID="22"]');
console.log(specificCustomerAnchors);
});
</script>
</head>
<body>
<a href="#" data-customerID="22">Customer 22</a>
<a href="#" data-customerID="33">Customer 33</a>
<a href="#" data-customerID="44">Customer 44</a>
<a href="#" data-customerID="22">Customer 22 - Duplicate</a>
</body>
</html>
In this example, the first console log will display all anchors that have the "customerID" data attribute, while the second console log will only show the anchors with the specific value of "22."
đĸ Time to Act!: Now that you're equipped with the knowledge of selecting elements by data attribute using jQuery, feel free to experiment, implement, and showcase your newfound skills!
Share your experiences, ask questions, or suggest other cool jQuery tricks in the comments below. Let's learn and grow together! đą
đ¤ That's all for now, folks! Stay tuned for more tech insights, handy tips, and exciting tutorials. Until then, happy coding! đģâ¨