Removing multiple classes (jQuery)
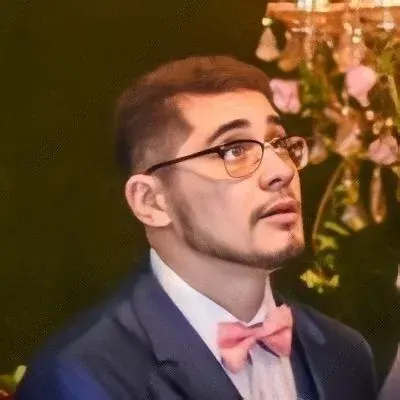
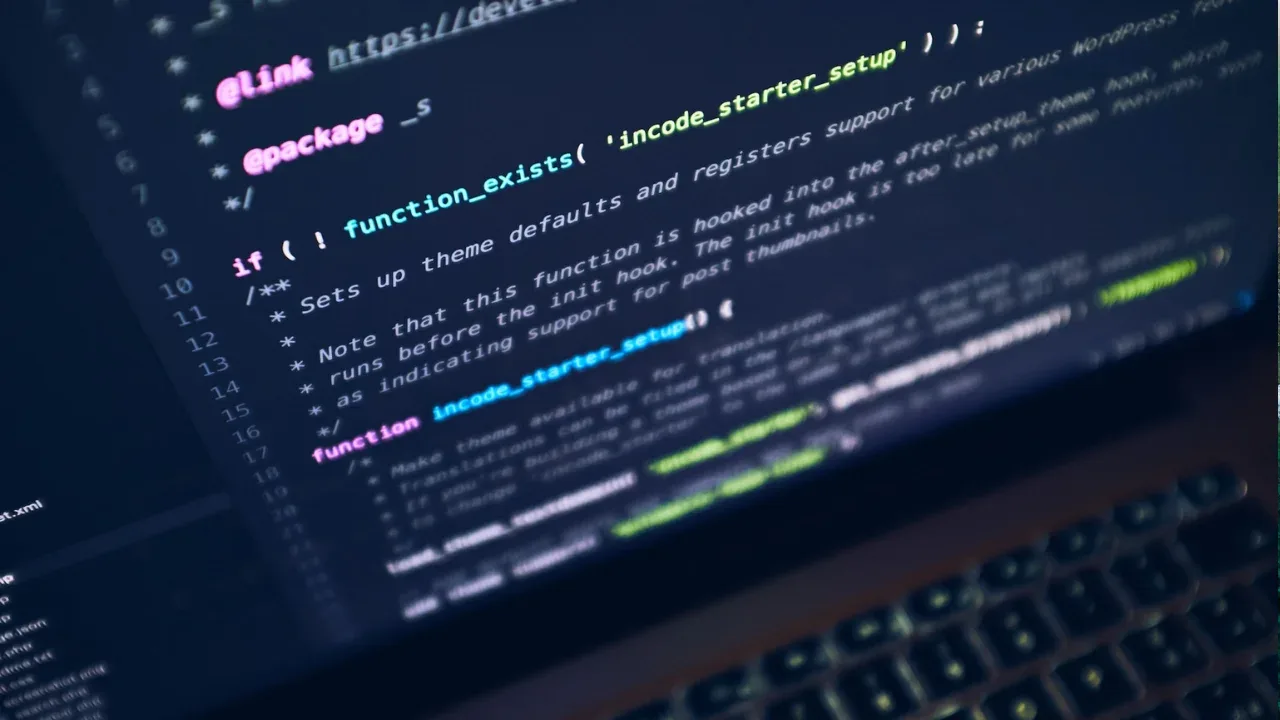
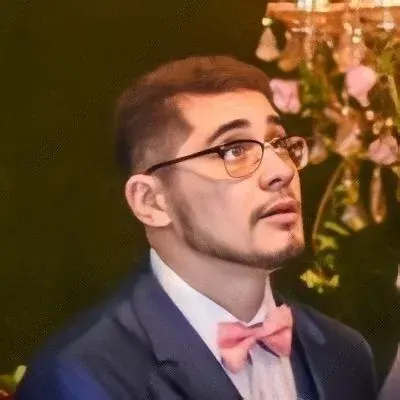
📝 Removing Multiple Classes in jQuery: The Easy Way
Are you tired of removing multiple classes from an element, but afraid to use the removeClass()
method because it removes all classes? Don't worry, we've got you covered! In this guide, we'll show you a better way to rewrite your code without missing a beat. 🚀
The Problem: Removing Multiple Classes
So, you want to remove multiple classes from an element, but you don't want to use removeClass()
because it removes ALL classes. Let's take a look at your code:
$('element').removeClass('class1').removeClass('class2');
Now, although this code does the job, it could be more concise and readable. Plus, what if you have more than two classes to remove? You'd end up with a long chain of removeClass()
methods, which is not the most efficient way to handle this situation.
The Solution: removeClass()
with Multiple Arguments
Good news! jQuery's removeClass()
method allows you to remove multiple classes at once using a neat feature: passing multiple arguments. It's as simple as it sounds. 🙌
Here's the updated code:
$('element').removeClass('class1 class2');
By passing both class names as a single string, separated by a space, you can remove multiple classes in one go. This approach not only reduces the number of lines of code but also makes your intentions clear, improving code readability.
A Better Alternative: Chaining removeClass()
Now, if you're really into concise and readable code, you can take advantage of jQuery's method chaining capability to remove multiple classes in an even cleaner way. Let's see it in action:
$('element').removeClass('class1').removeClass('class2');
By chaining the removeClass()
method multiple times, you can remove your desired classes one after another. However, keep in mind that this approach might not be the best choice if you have a lot of classes to remove.
Conclusion
Removing multiple classes in jQuery is easier than you might think! Whether you opt for using removeClass()
with multiple arguments or chaining the method, remember to choose the method that best suits your situation and code readability.
So, don't be afraid to clean up your code and remove those unwanted classes. Give it a try and enjoy the benefits of cleaner and more expressive code!
🙌 Take Action - Share Your Thoughts!
Now that you know the easy way to remove multiple classes, it's time to put it into practice! Have you encountered any other challenges with jQuery? How did you solve them? Share your experience and thoughts with our community in the comments below. We'd love to hear from you! 😊