Official way to ask jQuery wait for all images to load before executing something
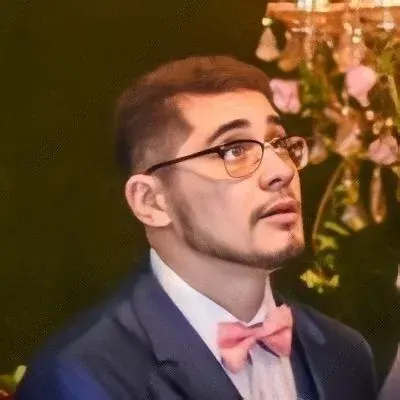
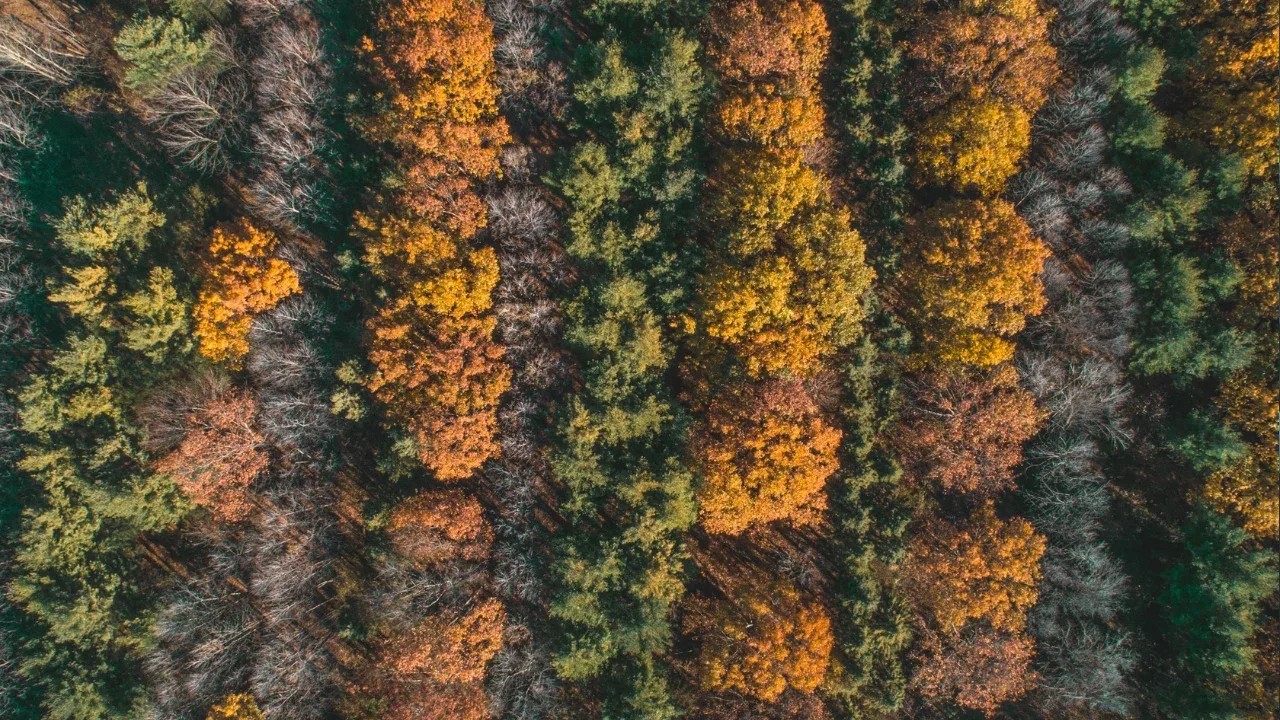
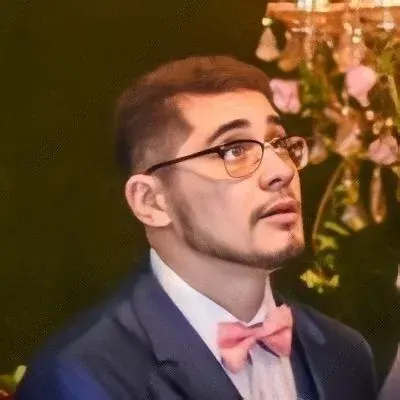
📷 Official way to ask jQuery to wait for all images to load
So you want to add some fancy animations to your website using jQuery, but you're facing a problem. You want to make sure that all the images are loaded before running the animation. After all, no one wants to see a glitchy animation, right? But don't worry, I've got you covered!
The Issue 😕
By default, when you use the following code in jQuery:
$(function() {
alert("DOM is loaded, but images not necessarily all loaded");
});
It waits for the DOM to load and executes your code. But here's the catch - it doesn't guarantee that all the images are loaded. Sometimes, the code might run even before the images have finished loading. And that's not what we want for our animation.
The Solution 💡
Luckily, there's an official way to handle this in jQuery. You can use the window.load
event, which triggers when all the page's content, including images, has finished loading. Here's how you can do it:
$(window).on('load', function() {
alert("All images have finished loading");
// Run your animation code here
});
By using $(window).on('load', function() { });
, you ensure that your code runs only after all the images on the page have finished loading. So now you can run your animation without worrying about any glitches!
The Alternative 🔄
But what if you don't want to use the window.load
event? Maybe you have a valid reason to stay away from it. In that case, you can use a plugin called imagesLoaded
. This plugin detects when images have been loaded and allows you to run your code accordingly.
First, include the imagesLoaded
plugin in your HTML:
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery.imagesloaded/4.1.4/imagesloaded.pkgd.min.js"></script>
Then, you can use it like this:
$('img').imagesLoaded(function() {
alert("All images have finished loading");
// Run your animation code here
});
By calling imagesLoaded()
on your images, you can make sure that the code inside the function runs only after all the images have finished loading. It's a great alternative if you want more control over the process.
Conclusion 🎉
You now have two official ways to ask jQuery to wait for all images to load before running your animation. Whether you choose to use the window.load
event or the imagesLoaded
plugin, both options ensure that your animation runs smoothly and glitch-free.
So go ahead, give it a try! Run those awesome animations on your website and impress your visitors. And don't forget to share your experiences and any other tips you have in the comments below. Let's help each other make the web a more delightful place! 🌟