jQuery if checkbox is checked
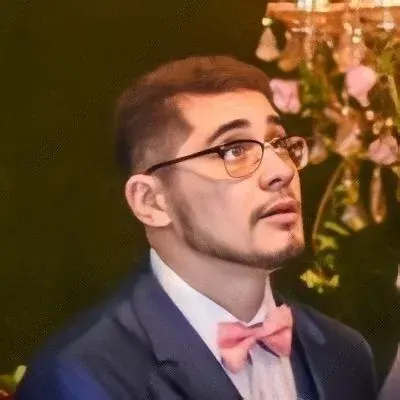
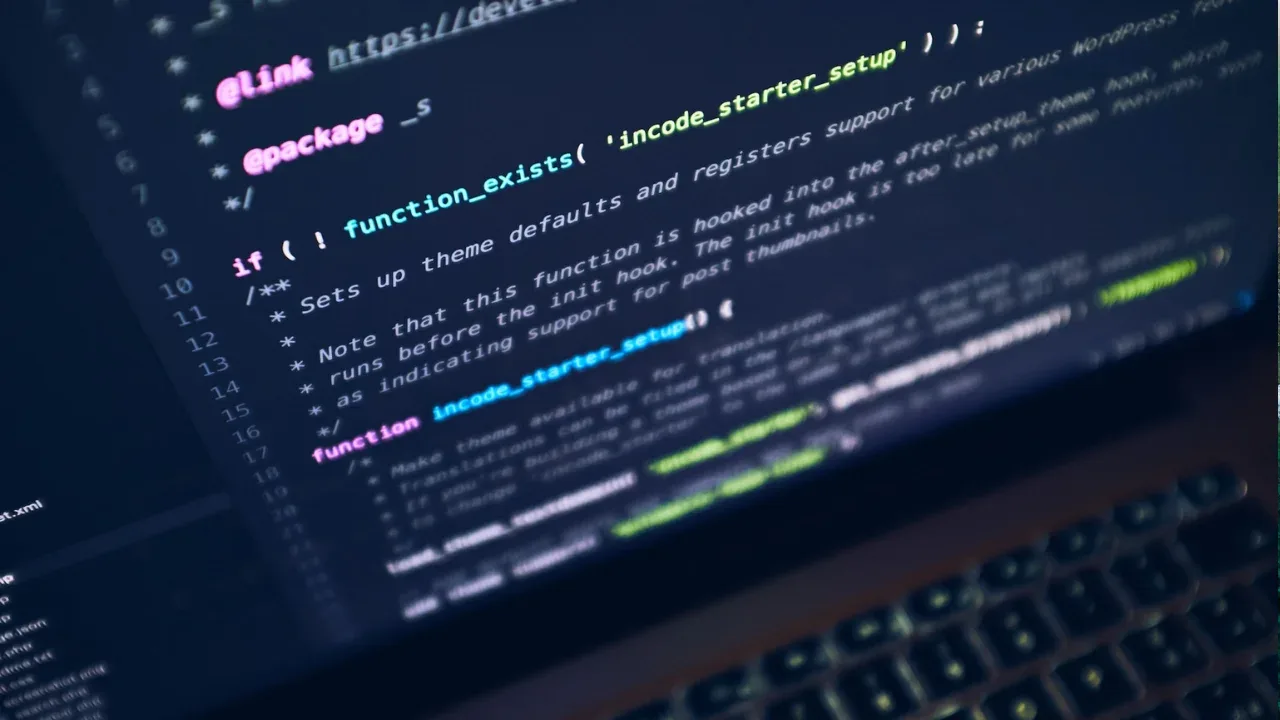
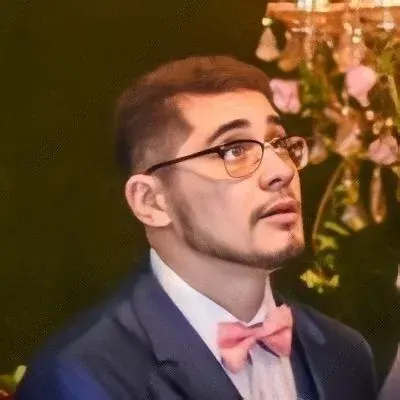
jQuery if Checkbox is Checked: A Complete Guide
Have you ever struggled with triggering a function only when a checkbox in the same tr
is checked using jQuery? 🤔 You're not alone! Many developers encounter issues and find the usual methods not working as expected. But fear not! In this guide, we'll address common problems and provide easy solutions to help you achieve your desired functionality. Let's dive in! 💪
The Problem: Triggering a Function when Checkbox is Checked
Consider the following code snippet:
<table width="316px" border="0" cellspacing="0" cellpadding="0" id="table-data">
<tbody>
<tr>
<td width="20px"><input type="checkbox" style="width:20px;" value="1" name="checkbox"></td>
<td width="200px"><a href="/admin/feedbackmanager/sortby/2/sortdesc/0">Page Name</a></td>
<td width="20px"><a href="/admin/feedbackmanager/sortby/3/sortdesc/0">Add</a></td>
</tr>
<tr>
<td><input type="checkbox" style="width:20px;" value="1" name="checkbox" class="checkbox_check"></td>
<td class="td_name">Timeplot</td>
<td><input class="add_menu_item_table" name="add_menu_item" value="Add" type="button"></td>
</tr>
<tr>
<td><input type="checkbox" style="width:20px;" value="1" name="checkbox" class="checkbox_check"></td>
<td class="td_name">Operations Manuals</td>
<td><input class="add_menu_item_table" name="add_menu_item" value="Add" type="button"></td>
</tr>
</tbody>
</table>
Suppose you want to trigger a function only when the checkbox in the same tr
is checked upon clicking the button with the class add_menu_item_table.
The checkbox element has the class checkbox_check
.
The Solution: Fixing the JavaScript/JQuery Function
Your current JavaScript code is as follows:
$(".add_menu_item_table").live('click', function() {
var value_td = $(this).parents('tr').find('td.td_name').text();
if ($('input.checkbox_check').attr(':checked')); {
// Function code goes here
}
});
The problem lies in the usage of the attr
method. Instead of using attr(':checked')
as you did, you should use is(':checked')
. The correct code should look like this:
$(".add_menu_item_table").live('click', function() {
var value_td = $(this).parents('tr').find('td.td_name').text();
if ($('input.checkbox_check').is(':checked')) {
// Function code goes here
}
});
By using the is(':checked')
method, you can properly check if the checkbox is checked or not within the if
condition.
Putting It All Together
To illustrate how the corrected code works, let's modify it slightly to show an alert when the checkbox is checked:
$(".add_menu_item_table").on('click', function() {
var value_td = $(this).parents('tr').find('td.td_name').text();
if ($('input.checkbox_check').is(':checked')) {
alert("Checkbox is checked! Value: " + value_td);
}
});
By clicking the "Add" button next to the "Timeplot" or "Operations Manuals" row, you'll see an alert only if the respective checkbox is checked. This way, the function will only trigger when the checkbox meets the specified condition.
Conclusion: Customize it!
With the corrected code, you can now trigger a function only when a checkbox in the same tr
is checked using jQuery. Feel free to customize it further to suit your specific needs. You can perform various actions like manipulating DOM elements, making AJAX requests, or updating UI components based on the checkbox's checked state.
Now that you know the solution, go ahead and apply it to your projects! Let us know how it works for you and if you encounter any new challenges. Don't forget to share this guide with fellow developers who may find it helpful too. Cheers! 🎉